Convert a string to lowercase using java's String.toLowerCase() function
String.toLowerCase() function is a very useful and common string processing function in Java, which can convert a string to lowercase form. In this article, we will introduce how to use this function and give some related code examples.
First, let us take a look at the basic syntax of the String.toLowerCase() function. It doesn't have any parameters, just call it. The following is a sample code:
String str = "Hello World"; String lowercaseStr = str.toLowerCase(); System.out.println(lowercaseStr);
The output result will be:
hello world
It can be seen that the function converts all uppercase letters in the original string into lowercase letters, and returns a new string.
In practical applications, we often need to convert the input string into lowercase for processing. For example, when users enter their username or password, we usually convert them into lowercase to avoid verification problems caused by uppercase and lowercase. The following is a code example for user login:
import java.util.Scanner; public class LoginSystem { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("请输入用户名:"); String username = scanner.nextLine(); System.out.print("请输入密码:"); String password = scanner.nextLine(); // 将用户名和密码转换为小写形式 String lowercaseUsername = username.toLowerCase(); String lowercasePassword = password.toLowerCase(); // 进行验证 if (lowercaseUsername.equals("admin") && lowercasePassword.equals("password")) { System.out.println("登录成功!"); } else { System.out.println("用户名或密码错误!"); } } }
In the above code, we first use the Scanner class to obtain the user name and password entered by the user. Then, use the String.toLowerCase() function to convert them to lowercase. Finally, we verify the username and password. In this way, regardless of whether the user enters uppercase or lowercase letters, the correct result will be obtained.
In addition to user login to the system, the String.toLowerCase() function can also be used to compare whether strings are equal. The following is a code example:
String str1 = "HELLO"; String str2 = "hello"; if (str1.toLowerCase().equals(str2.toLowerCase())) { System.out.println("两个字符串相等!"); } else { System.out.println("两个字符串不相等!"); }
The output result is:
The two strings are equal!
By calling the String.toLowerCase() function, we can avoid sensitivity to string case and provide more flexible and accurate string comparison.
In programming, string processing is one of the common tasks. The String.toLowerCase() function provides us with a simple and convenient way to convert strings to lowercase, making string processing more flexible and easier to operate. Whether it is user-entered strings, string comparisons, or other application scenarios, the String.toLowerCase() function can come in handy. I hope this article can help you better understand and use this important string processing function.
The above is the detailed content of Convert a string to lowercase using java's String.toLowerCase() function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
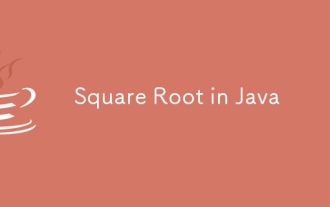
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
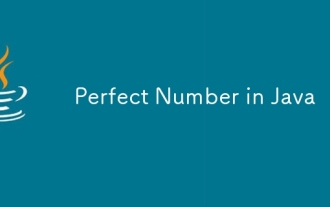
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
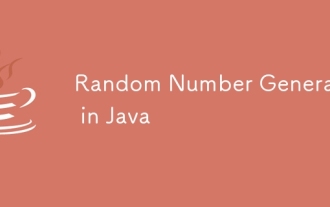
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
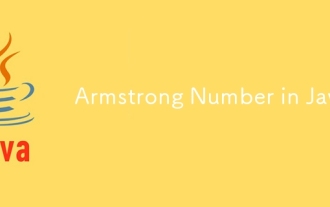
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
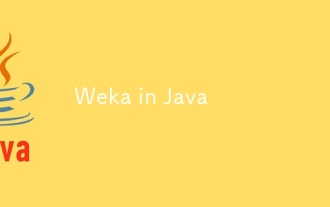
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
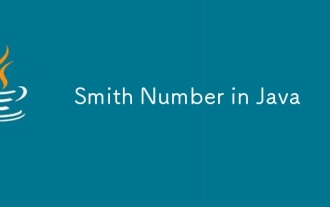
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
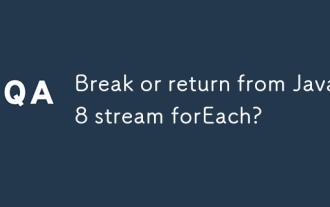
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
