


How to convert other types to string using valueOf() function of String class in Java
How does Java use the valueOf() function of the String class to convert other types into strings
In Java, we often need to convert other types of values into strings. At this time we can use the String class The valueOf() function is used to complete this operation. The valueOf() function of the String class has multiple overloaded forms that can be used to convert various types of values into strings. Below we will introduce how to use the valueOf() function for conversion and give some sample code.
- Convert basic data types to strings
We can use the valueOf() function to convert values of basic data types into strings. For example, convert an int type value to a string:
int num = 100; String str = String.valueOf(num); System.out.println("转换后的字符串为:" + str);
The output result is: "The converted string is: 100".
Similarly, we can also convert values of other basic data types (such as double, float, long, etc.) into strings using the valueOf() function.
- Convert a character array or character sequence to a string
We can use the valueOf() function to convert a character array or character sequence into a string. For example, convert a character array to a string:
char[] chars = {'H', 'e', 'l', 'l', 'o'}; String str = String.valueOf(chars); System.out.println("转换后的字符串为:" + str);
The output result is: "The converted string is: Hello".
In addition, we can also convert character sequences (such as StringBuilder, StringBuffer, etc.) into strings using the valueOf() function.
- Convert objects to strings
Using the valueOf() function, we can also convert some specific types of objects to strings. For example, convert an integer object to a string:
Integer number = new Integer(100); String str = String.valueOf(number); System.out.println("转换后的字符串为:" + str);
The output result is: "The converted string is: 100".
Similarly, we can also convert other types of objects (such as Boolean, Float, Double, etc.) into strings using the valueOf() function.
It should be noted that if the object's class does not override the toString() method, then the valueOf() function will call the object's toString() method by default for conversion.
- Summary
By using the valueOf() function of the String class, we can easily convert other types of values into strings. Whether it is a basic data type, character array, character sequence or object, conversion can be achieved by calling the valueOf() function. When writing Java programs, we often need to convert different types of values into strings. At this time, the valueOf() function becomes a very useful tool.
This article gives some relevant sample codes for readers’ reference and use. Of course, in actual applications, we also need to decide whether to use the valueOf() function for conversion based on specific needs and scenarios. At the same time, for some special needs, we can also use other provided type conversion methods or libraries for conversion. In short, the valueOf() function of the String class is a flexible and convenient way to perform type conversion in Java.
I hope this article can help readers and bring some basic understanding about using the valueOf() function of the String class for type conversion.
The above is the detailed content of How to convert other types to string using valueOf() function of String class in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


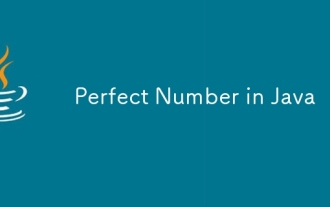
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
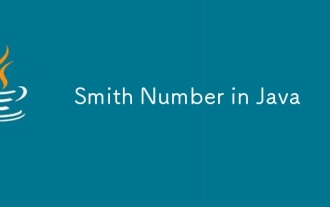
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
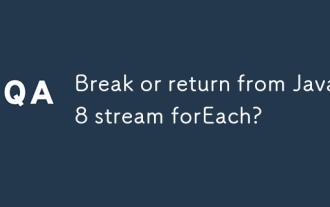
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
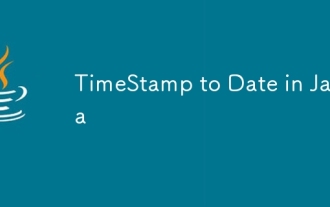
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
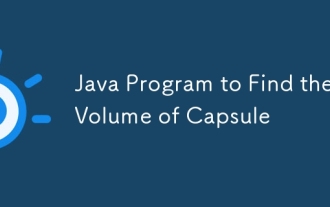
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
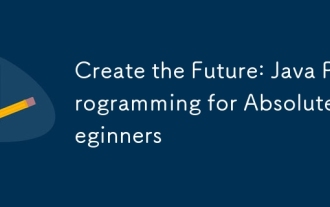
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
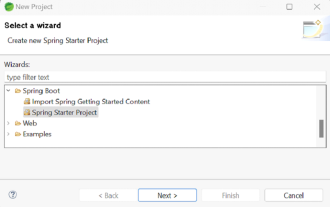
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
