Use PHP function 'sort' to sort an array in ascending order
Use the PHP function "sort" to sort the array in ascending order
In PHP, you can easily use the built-in function to sort the array. Among them, the sort function is one of the most commonly used functions, which can sort an array in ascending order. This article will introduce how to use the sort function and give corresponding code examples.
The syntax of the sort function is as follows:
sort(array &$array, int $sort_flags = SORT_REGULAR): bool
Parameter description:
- $array : The array to be sorted, required.
- $sort_flags: Optional parameter, used to specify the sorting method. The default value is SORT_REGULAR, which is the usual sorting method. Other available sorting methods include SORT_NUMERIC (sort by numeric size), SORT_STRING (sort by lexicographic order of strings), etc. For details, please refer to the PHP official documentation.
Return value: If the sorting is successful, return true; otherwise, return false.
Next, let us use a simple example to demonstrate how to use the sort function to sort an array.
1 2 3 4 5 6 7 8 9 |
|
The output of the above code will be: apple banana grape orange
In the above example code, we have created an array $fruits containing four fruits. Then, use the sort function to sort the array. Finally, use a foreach loop to iterate through the sorted array $fruits and output each element in turn.
It should be noted that the sort function will directly modify the original array instead of returning a new sorted array. Therefore, operations can be performed directly on the sorted array without creating a new variable.
In addition, we can also use the sort function to sort combinations of numbers and strings. Here is an example:
1 2 3 4 5 6 7 8 9 |
|
The output of the above code will be: 1 2 5 10 30
In this example, we have created an array $numbers containing five numbers. Then, use the sort function to sort the array, specifying the sorting method as SORT_NUMERIC, that is, sorting according to numerical size. Finally, use a foreach loop to iterate through the sorted array $numbers and output each number in turn.
To summarize, using the PHP function sort can sort an array in ascending order very conveniently. You only need to pass in the array to be sorted, and the sort function will directly modify the original array. At the same time, we can also specify different sorting methods through the second parameter of the sort function to meet different sorting requirements. I hope the sample code in this article can help you understand and use the sort function. For more details, please refer to the PHP official documentation.
Reference materials:
- PHP official documentation: https://www.php.net/manual/en/function.sort.php
The above is the detailed content of Use PHP function 'sort' to sort an array in ascending order. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




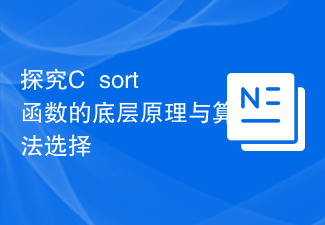
The bottom layer of the C++sort function uses merge sort, its complexity is O(nlogn), and provides different sorting algorithm choices, including quick sort, heap sort and stable sort.
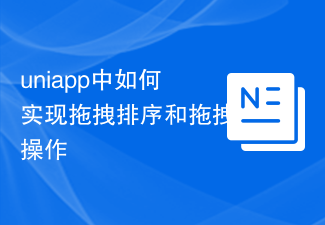
Uniapp is a cross-platform development framework. Its powerful cross-end capabilities allow developers to develop various applications quickly and easily. It is also very simple to implement drag-and-drop sorting and drag-and-drop operations in Uniapp, and it can support drag-and-drop operations of a variety of components and elements. This article will introduce how to use Uniapp to implement drag-and-drop sorting and drag-and-drop operations, and provide specific code examples. The drag-and-drop sorting function is very common in many applications. For example, it can be used to implement drag-and-drop sorting of lists, drag-and-drop sorting of icons, etc. Below we list
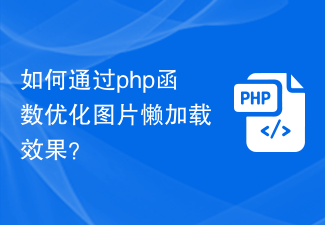
How to optimize the lazy loading effect of images through PHP functions? With the development of the Internet, the number of images in web pages is increasing, which puts pressure on page loading speed. In order to improve user experience and reduce loading time, we can use image lazy loading technology. Lazy loading of images can delay the loading of images. Images are only loaded when the user scrolls to the visible area, which can reduce the loading time of the page and improve the user experience. When writing PHP web pages, we can optimize the lazy loading effect of images by writing some functions. Details below
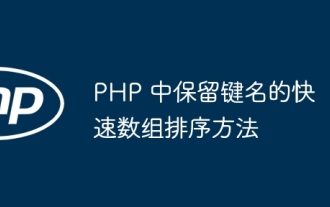
Fast array sorting method in PHP that preserves key names: Use the ksort() function to sort the keys. Use the uasort() function to sort using a user-defined comparison function. Practical case: To sort an array of user IDs and scores by score while retaining the user ID, you can use the uasort() function and a custom comparison function.
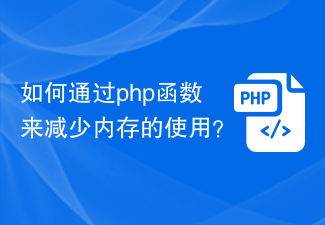
How to reduce memory usage through PHP functions. In development, memory usage is a very important consideration. If a large amount of memory is used in a program, it may cause slowdowns or even program crashes. Therefore, reasonably managing and reducing memory usage is an issue that every PHP developer should pay attention to. This article will introduce some methods to reduce memory usage through PHP functions, and provide specific code examples for readers' reference. Use the unset() function to release variables in PHP. When a variable is no longer needed, use
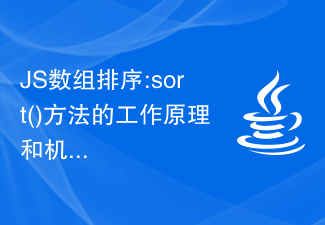
To deeply understand JS array sorting: the principles and mechanisms of the sort() method, specific code examples are required. Introduction: Array sorting is one of the very common operations in our daily front-end development work. The array sorting method sort() in JavaScript is one of the most commonly used array sorting methods. However, do you really understand the principles and mechanisms of the sort() method? This article will give you an in-depth understanding of the principles and mechanisms of JS array sorting, and provide specific code examples. 1. Basic usage of sort() method
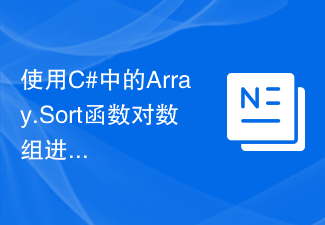
Title: Example of using the Array.Sort function to sort an array in C# Text: In C#, array is a commonly used data structure, and it is often necessary to sort the array. C# provides the Array class, which has the Sort method to conveniently sort arrays. This article will demonstrate how to use the Array.Sort function in C# to sort an array and provide specific code examples. First, we need to understand the basic usage of the Array.Sort function. Array.So
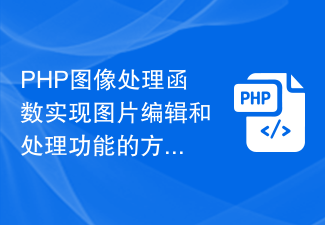
PHP image processing functions are a set of functions specifically used to process and edit images. They provide developers with rich image processing functions. Through these functions, developers can implement operations such as cropping, scaling, rotating, and adding watermarks to images to meet different image processing needs. First, I will introduce how to use PHP image processing functions to achieve image cropping function. PHP provides the imagecrop() function, which can be used to crop images. By passing the coordinates and size of the cropping area, we can crop the image
