


PHP function introduction—empty(): Check whether the variable is empty
PHP function introduction—empty(): Check whether a variable is empty
In PHP programming, it is often necessary to judge variables. It is a common requirement to judge whether a variable is empty. PHP's built-in empty() function is used to check whether the variable is empty. This article will introduce the usage of the empty() function and provide some practical code examples.
The usage of the empty() function is very simple. It accepts one parameter and returns a Boolean value. The empty() function returns true when the value of the parameter is one of the following situations, otherwise it returns false:
- If the value of the variable is 0 or the string "0", it is considered empty.
- If the value of the variable is false or null, it is considered empty.
- If the value of a variable is an empty array (array()) or an empty object with no elements, it is considered empty.
- If the variable is not set at all, it is considered empty.
The following is a sample code showing the usage of the empty() function:
<?php $var1 = ''; $var2 = 0; $var3 = false; $var4 = null; $var5 = array(); $var6; // not set echo 'var1 is empty: ' . (empty($var1) ? 'true' : 'false') . '<br>'; echo 'var2 is empty: ' . (empty($var2) ? 'true' : 'false') . '<br>'; echo 'var3 is empty: ' . (empty($var3) ? 'true' : 'false') . '<br>'; echo 'var4 is empty: ' . (empty($var4) ? 'true' : 'false') . '<br>'; echo 'var5 is empty: ' . (empty($var5) ? 'true' : 'false') . '<br>'; echo 'var6 is empty: ' . (empty($var6) ? 'true' : 'false') . '<br>'; ?>
Running the above code will output the following results:
var1 is empty: true var2 is empty: true var3 is empty: true var4 is empty: true var5 is empty: true var6 is empty: true
In actual programming In , we often need to judge whether a variable is empty to make logical judgments. For example, we can use the empty() function to check whether the form data entered by the user is empty to ensure the validity of the data.
<?php if (empty($_POST['username'])) { echo '请输入用户名'; } else { // 其他逻辑处理 } ?>
In the above code snippet, we use the empty() function to check whether the user name entered by the user is empty. If it is empty, prompt information will be output, otherwise other logical processing will be performed.
It should be noted that the empty() function can only be used for variable checking and cannot be used to directly determine the truth or falsehood of constants or expressions. For example, if we need to determine whether a constant is empty, we should use the isset() function. If you need to determine whether an expression is true or false, you should use an if statement.
In short, the empty() function is a very practical function in PHP, which allows us to conveniently check whether a variable is empty. In actual programming, we often need to use the empty() function to perform form data verification, logical judgment, etc. I hope that the introduction and sample code of this article can help readers better understand and use the empty() function.
The above is the detailed content of PHP function introduction—empty(): Check whether the variable is empty. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


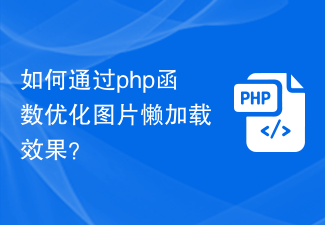
How to optimize the lazy loading effect of images through PHP functions? With the development of the Internet, the number of images in web pages is increasing, which puts pressure on page loading speed. In order to improve user experience and reduce loading time, we can use image lazy loading technology. Lazy loading of images can delay the loading of images. Images are only loaded when the user scrolls to the visible area, which can reduce the loading time of the page and improve the user experience. When writing PHP web pages, we can optimize the lazy loading effect of images by writing some functions. Details below
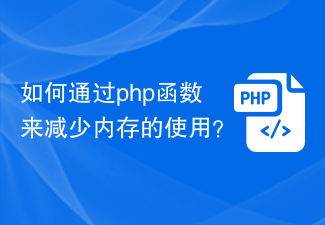
How to reduce memory usage through PHP functions. In development, memory usage is a very important consideration. If a large amount of memory is used in a program, it may cause slowdowns or even program crashes. Therefore, reasonably managing and reducing memory usage is an issue that every PHP developer should pay attention to. This article will introduce some methods to reduce memory usage through PHP functions, and provide specific code examples for readers' reference. Use the unset() function to release variables in PHP. When a variable is no longer needed, use
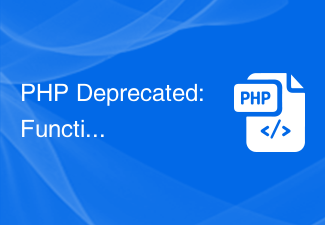
PHPDeprecated: Functionereg_replace()isdeprecated-Solution When developing in PHP, we often encounter the problem of some functions being declared deprecated. This means that in the latest PHP versions, these functions may be removed or replaced. One common example is the ereg_replace() function. ereg_replace
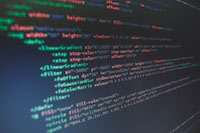
This article will explain in detail how PHP determines whether a specified key exists in an array. The editor thinks it is very practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP determines whether a specified key exists in an array: In PHP, there are many ways to determine whether a specified key exists in an array: 1. Use the isset() function: isset($array["key"]) This function returns a Boolean value, true if the specified key exists, false otherwise. 2. Use array_key_exists() function: array_key_exists("key",$arr
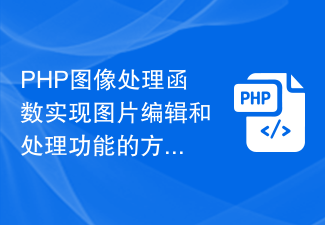
PHP image processing functions are a set of functions specifically used to process and edit images. They provide developers with rich image processing functions. Through these functions, developers can implement operations such as cropping, scaling, rotating, and adding watermarks to images to meet different image processing needs. First, I will introduce how to use PHP image processing functions to achieve image cropping function. PHP provides the imagecrop() function, which can be used to crop images. By passing the coordinates and size of the cropping area, we can crop the image
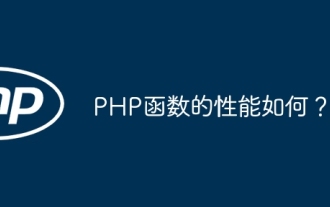
The performance of different PHP functions is crucial to application efficiency. Functions with better performance include echo and print, while functions such as str_replace, array_merge, and file_get_contents have slower performance. For example, the str_replace function is used to replace strings and has moderate performance, while the sprintf function is used to format strings. Performance analysis shows that it only takes 0.05 milliseconds to execute one example, proving that the function performs well. Therefore, using functions wisely can lead to faster and more efficient applications.
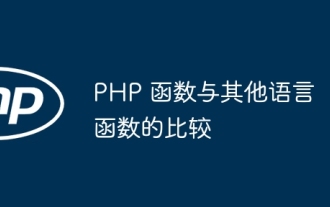
PHP functions have similarities with functions in other languages, but also have some unique features. Syntactically, PHP functions are declared with function, JavaScript is declared with function, and Python is declared with def. In terms of parameters and return values, PHP functions accept parameters and return a value. JavaScript and Python also have similar functions, but the syntax is different. In terms of scope, functions in PHP, JavaScript and Python all have global or local scope. Global functions can be accessed from anywhere, and local functions can only be accessed within their declaration scope.

PHP function introduction: strtr() function In PHP programming, the strtr() function is a very useful string replacement function. It is used to replace specified characters or strings in a string with other characters or strings. This article will introduce the usage of strtr() function and give some specific code examples. The basic syntax of the strtr() function is as follows: strtr(string$str, array$replace) where $str is the original word to be replaced.
