


Use java's Character.isDefined() function to determine whether a character is a defined character
Use Java's Character.isDefined() function to determine whether a character is a defined character
In Java programming, sometimes you need to determine whether a character is a defined character. For convenience, Java provides the isDefined() function of the Character class, which can help us quickly determine whether a character is a defined character. This article explains how to use this function and provides some code examples.
The Character class is one of the basic types that represents a single character in Java. In Java, characters are represented by Unicode encoding, and there are more than 130,000 characters in the Unicode character set. Some characters may be defined characters and some may be undefined characters. The so-called defined characters refer to characters with corresponding encodings in the Unicode character set. Undefined characters refer to characters that have no corresponding encoding in the Unicode character set.
The isDefined() function is a method provided by the Character class to determine whether a character is a defined character. The syntax of this function is as follows:
public static boolean isDefined(char ch)
Among them, ch is the character to be judged. Returns true if the character is a defined character; otherwise returns false.
The following is a sample code that uses the isDefined() function to determine whether a character is a defined character:
public class Main { public static void main(String[] args) { char ch = 'A'; if(Character.isDefined(ch)) { System.out.println("字符 " + ch + " 是已定义字符。"); } else { System.out.println("字符 " + ch + " 不是已定义字符。"); } } }
In the above code, first define a character variable ch and initialize it to 'A '. Then use the isDefined() function to determine whether ch is a defined character. If it is a defined character, output "Character A is a defined character."; otherwise, output "Character A is not a defined character."
Run the above code, the output result is "Character A is a defined character." ". Because the uppercase letter 'A' is a defined character, the corresponding Unicode encoding is 65.
Use the isDefined() function to easily determine whether a character is a defined character. In practical applications, we can apply it to character verification, data filtering, character replacement and other scenarios.
It should be noted that the isDefined() function can only determine whether a single character is a defined character, and cannot directly determine whether a string is a defined character. If you need to determine whether a string consists of defined characters, you can traverse each character in the string and determine whether it is a defined character one by one.
In summary, using Java's Character.isDefined() function can easily determine whether a character is a defined character. The usage of this function is simple and clear, and it can help us deal with character-related problems. In actual development, we can flexibly apply the isDefined() function according to needs to improve the robustness and maintainability of the code.
The above is the detailed content of Use java's Character.isDefined() function to determine whether a character is a defined character. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


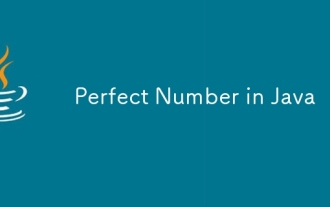
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
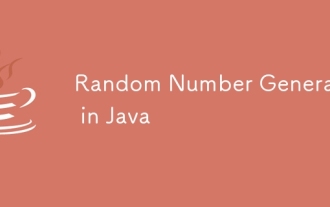
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
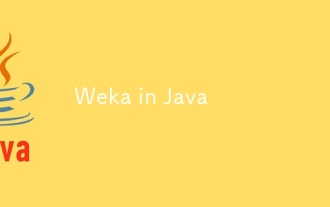
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
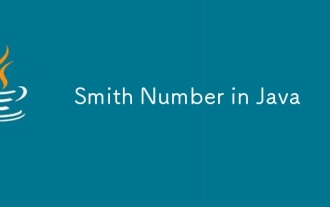
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
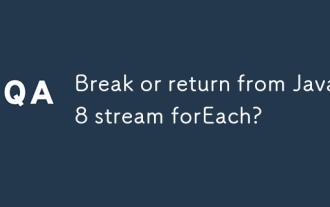
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
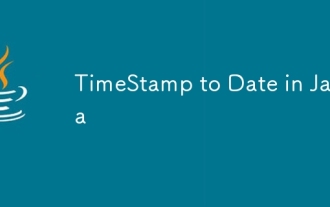
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
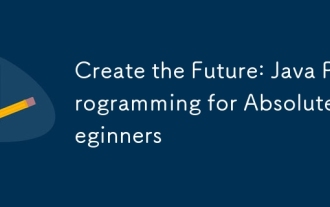
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
