


How to use Alibaba Cloud Mobile Push Extension to implement global message push in PHP applications
How to use Alibaba Cloud Mobile Push extension to implement global message push in PHP applications
Alibaba Cloud Mobile Push (Aliyun Push) is a global message push service provided by Alibaba Cloud, supporting mobile applications, Huawei Push, Xiaomi Push and other major mobile platforms push messages quickly. This article will introduce how to use Alibaba Cloud mobile push extension in PHP applications to implement global message push.
First, we need to create a mobile application in the Alibaba Cloud console and obtain the corresponding AppKey and AppSecret. Next, we need to install the two extensions aliyun/aliyun-openapi-php-sdk and aliyun/aliyun-mns-php-sdk to implement Alibaba Cloud mobile push operations. These two extensions can be installed through composer. The following is the content of the composer.json file:
{ "require": { "aliyun/aliyun-openapi-php-sdk": "^1.2", "aliyun/aliyun-mns-php-sdk": "^0.9.1" } }
Execute the composer install
command on the command line to install the extension.
After the installation is complete, we can create a PHP file named push.php
and use the following code to implement global message push:
<?php require 'vendor/autoload.php'; use AliyunOpenApiRegionsEndpointConfig; use AliyunPushRequestV20160801 as Push; // 配置AppKey和AppSecret $appKey = 'your_app_key'; $appSecret = 'your_app_secret'; // 配置阿里云的接入地址和区域 $regionId = 'cn-hangzhou'; $endpointName = 'cn-hangzhou'; // 配置推送目标和消息内容 $deviceId = 'your_device_id'; $message = 'your_message'; $endpoints = EndpointConfig::getEndpoints(); // 获取推送相关的阿里云Endpoint $pushEndpoint = $endpoints[$endpointName][PushRequest::SERVICE_NAME][$regionId]; // 初始化阿里云移动推送 $client = AliyunOpenApiCoreDefaultAcsClient::getAcsClient($regionId, $pushEndpoint, $appKey, $appSecret); // 构造推送请求 $request = new PushPushRequest(); // 设置推送目标 $request->setAppKey($appKey); $request->setTarget('DEVICE'); $request->setTargetValue($deviceId); // 设置推送消息 $request->setMessageType('NOTICE'); $request->setMessageBody($message); // 执行推送 $response = $client->getAcsResponse($request); // 判断推送结果 if ($response->getCode() == "OK") { echo "消息推送成功"; } else { echo "消息推送失败:" . $response->getMessage(); }
In the above code, You need to replace your_app_key
and your_app_secret
with the AppKey and AppSecret of the mobile application you created in the Alibaba Cloud console. You need to replace your_device_id
with the device ID you want to push messages to. Replace your_message
with the content of the message you want to send.
Global message push can be achieved by executing the php push.php
command.
Summary:
This article introduces how to use the Alibaba Cloud mobile push extension to implement global message push in PHP applications. By configuring AppKey and AppSecret, and constructing push requests, we can use Alibaba Cloud Mobile Push to quickly push messages to major mobile platforms such as mobile applications, Huawei Push, and Xiaomi Push. Through the above sample code, you can easily implement the global message push function. At the same time, Alibaba Cloud Mobile Push also provides more advanced functions, such as scheduled push, conditional push, etc., which can be expanded according to actual needs. I hope this article will help you implement global message push in PHP applications.
The above is the detailed content of How to use Alibaba Cloud Mobile Push Extension to implement global message push in PHP applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


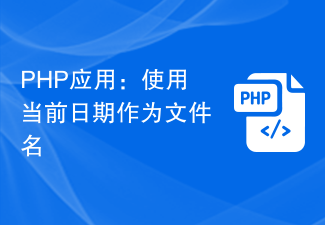
In PHP applications, we sometimes need to save or upload files using the current date as the file name. Although it is possible to enter the date manually, it is more convenient, faster and more accurate to use the current date as the file name. In PHP, we can use the date() function to get the current date. The usage method of this function is: date(format, timestamp); where format is the date format string, and timestamp is the timestamp representing the date and time. If this parameter is not passed, it will be used
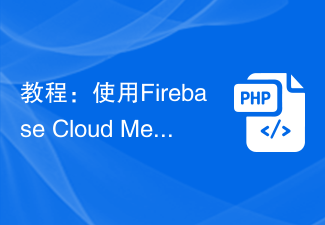
Tutorial: Using Firebase Cloud Messaging to implement scheduled message push functions in PHP applications Overview Firebase Cloud Messaging (FCM) is a free message push service provided by Google, which can help developers send real-time messages to Android, iOS and Web applications. This tutorial will lead you to use FCM to implement scheduled message push functions through PHP applications. Step 1: Create a Firebase project First, in F

1. What is generic programming? Generic programming refers to the implementation of a common data type in a programming language so that this data type can be applied to different data types, thereby achieving code reuse and efficiency. PHP is a dynamically typed language. It does not have a strong type mechanism like C++, Java and other languages, so it is not easy to implement generic programming in PHP. 2. Generic programming in PHP There are two ways to implement generic programming in PHP: using interfaces and using traits. Create an interface in PHP using an interface
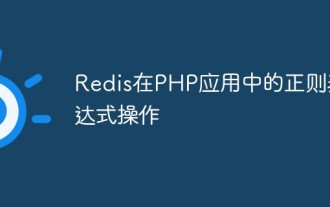
Redis is a high-performance key-value storage system that supports a variety of data structures, including strings, hash tables, lists, sets, ordered sets, etc. At the same time, Redis also supports regular expression matching and replacement operations on string data, which makes it highly flexible and convenient in developing PHP applications. To use Redis for regular expression operations in PHP applications, you need to install the phpredis extension first. This extension provides a way to communicate with the Redis server.
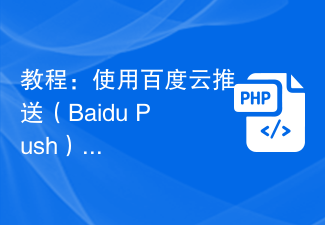
Tutorial: Use Baidu Cloud Push (BaiduPush) extension to implement message push function in PHP applications Introduction: With the rapid development of mobile applications, message push function is becoming more and more important in applications. In order to realize instant notification and message push functions, Baidu provides a powerful cloud push service, namely Baidu Cloud Push (BaiduPush). In this tutorial, we will learn how to use Baidu Cloud Push Extension (PHPSDK) to implement message push functionality in PHP applications. We will use Baidu Cloud
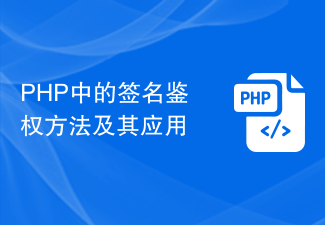
Signature Authentication Method and Application in PHP With the development of the Internet, the security of Web applications has become increasingly important. Signature authentication is a common security mechanism used to verify the legitimacy of requests and prevent unauthorized access. This article will introduce the signature authentication method and its application in PHP, and provide code examples. 1. What is signature authentication? Signature authentication is a verification mechanism based on keys and algorithms. The request parameters are encrypted to generate a unique signature value. The server then decrypts the request and verifies the signature using the same algorithm and key.
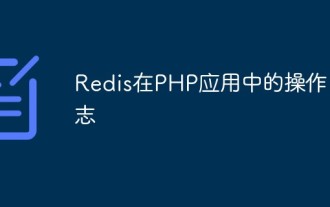
Redis operation logs in PHP applications In PHP applications, it has become more and more common to use Redis as a solution for caching or storing data. Redis is a high-performance key-value storage database that is fast, scalable, highly available, and has diverse data structures. When using Redis, in order to better understand the operation of the application and for data security, we need to have a Redis operation log. Redis operation log can record all clients on the Redis server
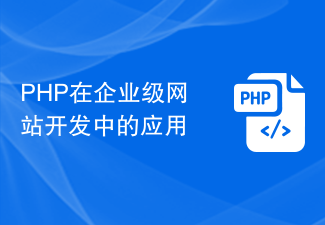
As one of the most popular server-side scripting languages, PHP is widely used in the development of enterprise-level websites. Its flexibility, scalability, and ease of use make PHP the language of choice for enterprise-level website development. This article will discuss the application of PHP in enterprise-level website development. First of all, PHP plays a key role in the development of enterprise-level websites. It can be used to build a variety of functions, including user authentication, data storage, data analysis, and report generation. PHP can be seamlessly integrated with databases and supports mainstream data
