


How to use the Aurora Push extension to implement batch message push function in PHP applications
How to use the Aurora Push extension to implement batch message push function in PHP applications
In the development of mobile applications, message push is a very important function. Jiguang Push is a commonly used message push service that provides rich functions and interfaces. This article will introduce how to use the Aurora Push extension to implement batch message push functionality in PHP applications.
Step one: Register a Jiguang Push account and obtain an API key
First, we need to register an account on the Jiguang Push official website (https://www.jiguang.cn/push). and create an app. After creating an application, you can find the corresponding App Key and Master Secret in the application details page. These two keys will be used for subsequent API calls.
Step 2: Install and configure the Aurora Push extension
In PHP applications, we can use third-party Aurora Push extensions to simplify the implementation of the push function. Here, we choose to use the JPush extension (https://github.com/jpush/jpush-api-php-client).
First, use composer to install the extension:
composer require jpush/jpush
After the installation is complete, create a new PHP file and enable the extension:
require __DIR__ . '/vendor/autoload.php'; use JPushClient as JPush; $appKey = 'your_app_key'; $masterSecret = 'your_master_secret'; $jpush = new JPush($appKey, $masterSecret);
In the above code, we introduced JPush class, and created a JPush instance by passing in the App Key and Master Secret.
Step 3: Write push code
Next, we can write the corresponding push code according to our needs. The JPush extension provides a variety of push methods, including broadcast push, alias push, label push, registration ID push, etc.
The following takes broadcast push as an example to demonstrate how to send a message to all devices:
$message = 'Hello, World!'; $response = $jpush->push() ->setPlatform('all') ->addAllAudience() ->setNotification([ 'alert' => $message, ]) ->send(); print_r($response);
In the above code, we first specify the push platform as all platforms (iOS, Android, etc.), Then select all audiences. Next, the push message content is set. Here we set the message content to be sent as "Hello, World!". Finally, call the send() method to send the push and get the response.
Step 4: Batch push messages
In addition to broadcast push, we can also use alias push to implement batch message push. Alias push can be pushed according to the alias of the device, and the target range of the push can be controlled according to your own needs.
The following is a sample code for batch push messages:
$alias1 = "alias1"; $alias2 = "alias2"; $messages = [ ["title" => "Message 1", "content" => "This is message 1"], ["title" => "Message 2", "content" => "This is message 2"] ]; $response = $jpush->push() ->setPlatform('all') ->addAlias([$alias1, $alias2]) ->addAndroidNotification('Hello', [ 'title' => 'New Message', 'extras' => [ 'messages' => json_encode($messages) ] ]) ->addIosNotification('Hello', [ 'sound' => 'default', 'category' => 'message', 'extras' => [ 'messages' => json_encode($messages) ] ]) ->send(); print_r($response);
In the above code, we first define two aliases "alias1" and "alias2", and then define the multiple aliases to be sent. Messages, each message includes title and content. Next, use the addAlias() method to specify the target alias for push. Finally, the push message content of the Android and iOS platforms are set respectively by calling the addAndroidNotification() and addIosNotification() methods. In push messages, we use the message content in JSON format, which can be modified appropriately as needed.
The above is how to use the Aurora Push extension to implement the batch message push function in PHP applications. Through Jiguang Push, we can easily implement the message push function and provide a better experience for the users of the application.
The above is the detailed content of How to use the Aurora Push extension to implement batch message push function in PHP applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
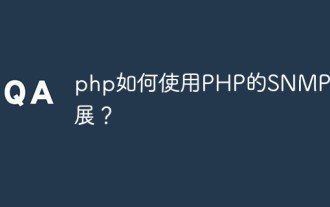
The SNMP extension for PHP is an extension that enables PHP to communicate with network devices through the SNMP protocol. Using this extension, you can easily obtain and modify the configuration information of network devices, such as CPU, memory, network interface and other information of routers, switches, etc. You can also perform control operations such as switching device ports. This article will introduce the basic knowledge of the SNMP protocol, how to install the SNMP extension of PHP, and how to use the SNMP extension in PHP to monitor and control network devices. 1. SN
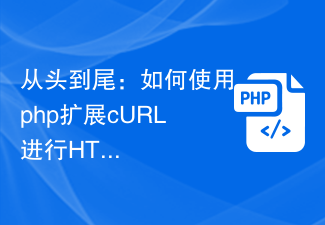
From start to finish: How to use php extension cURL for HTTP requests Introduction: In web development, it is often necessary to communicate with third-party APIs or other remote servers. Using cURL to make HTTP requests is a common and powerful way. This article will introduce how to use PHP to extend cURL to perform HTTP requests, and provide some practical code examples. 1. Preparation First, make sure that php has the cURL extension installed. You can execute php-m|grepcurl on the command line to check
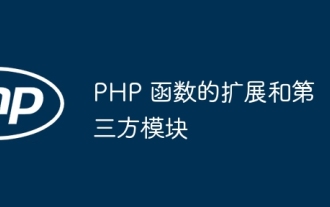
To extend PHP function functionality, you can use extensions and third-party modules. Extensions provide additional functions and classes that can be installed and enabled through the pecl package manager. Third-party modules provide specific functionality and can be installed through the Composer package manager. Practical examples include using extensions to parse complex JSON data and using modules to validate data.
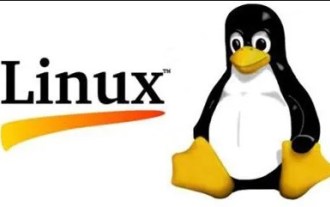
1.UncaughtError:Calltoundefinedfunctionmb_strlen(); When the above error occurs, it means that we have not installed the mbstring extension; 2. Enter the PHP installation directory cd/temp001/php-7.1.0/ext/mbstring 3. Start phpize(/usr/local/bin /phpize or /usr/local/php7-abel001/bin/phpize) command to install php extension 4../configure--with-php-config=/usr/local/php7-abel
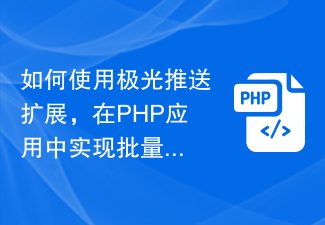
How to use the Aurora Push extension to implement batch message push function in PHP applications. In the development of mobile applications, message push is a very important function. Jiguang Push is a commonly used message push service that provides rich functions and interfaces. This article will introduce how to use the Aurora Push extension to implement batch message push functionality in PHP applications. Step 1: Register a Jiguang Push account and obtain an API key. First, we need to register on the Jiguang Push official website (https://www.jiguang.cn/push)
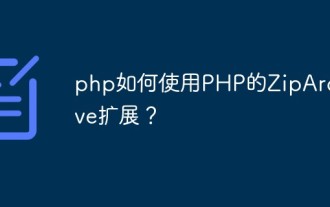
PHP is a popular server-side language that can be used to develop web applications and process files. The ZipArchive extension for PHP is a powerful tool for manipulating zip files in PHP. In this article, we’ll cover how to use PHP’s ZipArchive extension to create, read, and modify zip files. 1. Install the ZipArchive extension. Before using the ZipArchive extension, you need to ensure that the extension has been installed. The installation method is as follows: 1. Install
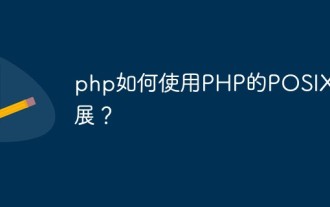
The POSIX extensions for PHP are a set of functions and constants that allow PHP to interact with POSIX-compliant operating systems. POSIX (PortableOperatingSystemInterface) is a set of operating system interface standards designed to allow software developers to write applications that can run on various UNIX or UNIX-like operating systems. This article will introduce how to use POSIX extensions for PHP, including installation and use. 1. Install the POSIX extension of PHP in
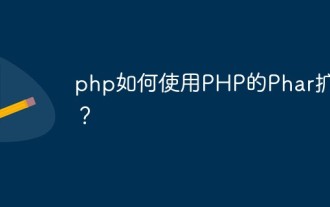
With the development of PHP and the continuous expansion of application scenarios, Phar extension has become an important part of PHP programming. Phar is the abbreviation of PHPArchive, which can package multiple PHP files and resources into a single file for easy distribution and management. This article will introduce how to use PHP's Phar extension for packaging and management. Installing the Phar extension First, we need to check whether PHP has the Phar extension installed. Under Linux, enter the following command through the terminal: php -m
