


Use the ioutil.ReadFile function to read the file contents and return a byte slice
Title: Use the ioutil.ReadFile function to read file contents and return byte slices
Article content:
In the standard library of the Go language, there is a very commonly used function ioutil. ReadFile(), which can be used to read the content from the specified file and return a byte slice. This function provides a simple and convenient way to read files and conveniently process the file contents for further processing.
Below, we will use a simple code example to show how to use ioutil.ReadFile() to read the file content and process the file content.
First, we need a text file, such as a file named sample.txt, to be used as our test file. Write a few lines of text content in sample.txt, for example:
Hello World! Welcome to Go programming. File handling in Go is simple and powerful.
Next, we can use the following code to read the content in sample.txt and return a byte slice:
package main import ( "fmt" "io/ioutil" ) func main() { filePath := "sample.txt" content, err := ioutil.ReadFile(filePath) if err != nil { fmt.Println("Failed to read file:", err) return } fmt.Println(string(content)) }
In the above code, we first introduced the two standard library packages "fmt" and "io/ioutil". Then, we defined a file path variable filePath
, specifying the file path we want to read. Next, we called the ioutil.ReadFile() function, passing in filePath
as a parameter to read the file content. This function returns two values, a byte slice of the file's contents and an error that may have occurred. We use err to receive the return value of the function and perform error handling.
If the file is read successfully, we convert the byte slice into a string and use the fmt.Println() function to print out the file content. If the file reading fails, the corresponding error message is printed.
Run the above code, the output result will be:
Hello World! Welcome to Go programming. File handling in Go is simple and powerful.
By using the ioutil.ReadFile() function, we can easily and quickly read the file content and perform further processing. We can also write content to the file through other functions provided by the ioutil package, such as the ioutil.WriteFile() function, to achieve more complete file processing functions.
To sum up, the ioutil.ReadFile() function is a convenient way to read files in the Go language. It can quickly read the file content and convert it into byte slices. By combining other file processing related functions, we can achieve more powerful file processing functions. I hope this article will be helpful to you in the process of reading file contents using the ioutil.ReadFile() function.
The above is the detailed content of Use the ioutil.ReadFile function to read the file contents and return a byte slice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
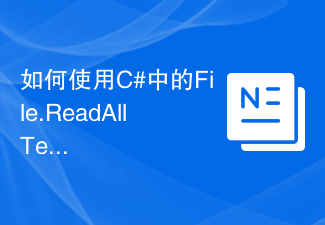
How to use the File.ReadAllText function in C# to read the contents of a text file. In C# programming, we often need to read the contents of a text file. File.ReadAllText is a very convenient function that can help us quickly read the entire contents of a text file. This article will introduce how to use the File.ReadAllText function and provide specific code examples. First, we need to introduce the System.IO namespace in order to use the related methods of the File class.
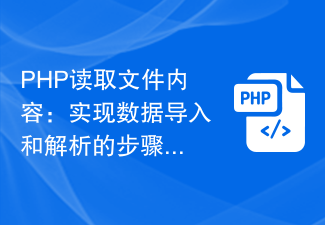
Reading file contents with PHP: Steps to implement data import and parsing Importing and parsing file contents is one of the very common operations in web development. File importing and parsing can be easily achieved using PHP, and this article will describe the steps to achieve this process and provide code examples. Step 1: Select the file to import and parse In PHP, you first need to select the file to import and parse. You can use the file selection form or specify the file path manually. Here is sample code for a file selection form: <formmethod
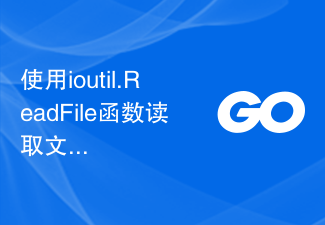
Title: Use the ioutil.ReadFile function to read file contents and return byte slices Article content: In the standard library of the Go language, there is a very commonly used function ioutil.ReadFile(), which can be used to read from a specified file content and returns a byte slice. This function provides a simple and convenient way to read files and conveniently process the file contents for further processing. Below, we will show you how to use ioutil.R with a simple code example
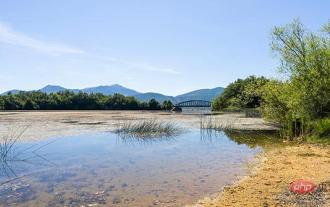
Files are everywhere, no matter which programming language we use, handling files is essential for every programmer. File processing is a process used to create files, write data and read data from them, Python There are a wealth of packages for processing different file types, which makes it easier and more convenient for us to complete file processing work. Outline of this article: Use the context manager to open files. File reading mode in Python. Read text files. Read CSV files. Get JSON File Open File Before accessing the contents of the file, we need to open the file. Python provides a built-in function that helps us open files in different modes. The open() function accepts two bases
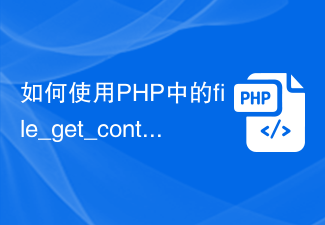
In PHP, we often need to read data from files. In this case, we can use the file_get_contents function. This function can simply read everything from a file and return it as a string. This is very useful in many scenarios, such as reading configuration files, reading log files, etc. In this article, we will explain how to read file contents using the file_get_contents function in PHP. Step 1: Open the file using file
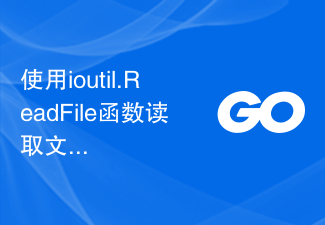
Title: Use the ioutil.ReadFile function to read file contents and return a string In Go language, there are many ways to read file contents and process them, one of them is to use the ReadFile function in the ioutil package. This article will introduce how to use the ioutil.ReadFile function to read a file and return its contents as a string. The ioutil.ReadFile function is a convenient method for reading file contents provided in the Go language standard library. it accepts a
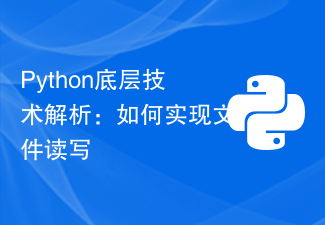
Analysis of Python's underlying technology: How to implement file reading and writing, specific code examples are required. In Python programming, file operations are one of the most common and important operations. File reading and writing involves Python's underlying I/O technology. This article will explore how to use Python to implement file reading and writing operations, and provide specific code examples. 1. File reading Python provides a variety of methods for reading file contents. The most common ones include using the open() function and using the with statement. Use the open() function
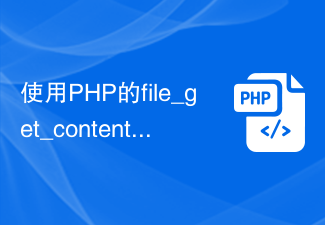
In PHP programming, reading file contents is a basic task. PHP provides many functions to accomplish this task, one of the most popular is file_get_contents. It reads the entire file into a string for further processing. In this article, we will learn how to read file contents using file_get_contents function. Syntax The syntax of file_get_contents is as follows: stringfile_get_cont
