How to use PHP functions to verify data storage and reading?
How to use PHP functions to verify data storage and reading?
When developing PHP applications, data verification is a very important step. By verifying the data entered by the user, malicious input and the storage of incorrect data can be effectively prevented, ensuring the integrity and security of the data. PHP provides some built-in functions that can easily perform data verification operations.
- Email format verification
During user registration or implementation of email-related functions, it is often necessary to verify the email address entered by the user. PHP provides the filter_var function to verify whether the format of the email address is correct:
$email = "test@example.com"; if(filter_var($email, FILTER_VALIDATE_EMAIL)){ echo "邮箱地址格式正确"; }else{ echo "邮箱地址格式错误"; }
- URL format verification
For functions that need to process URL addresses, you can also use the filter_var function. Verify whether the URL format is correct:
$url = "http://www.example.com"; if(filter_var($url, FILTER_VALIDATE_URL)){ echo "URL地址格式正确"; }else{ echo "URL地址格式错误"; }
- IP address verification
PHP's filter_var function can also be used to verify whether the IP address format is correct:
$ip = "192.168.1.1"; if(filter_var($ip, FILTER_VALIDATE_IP)){ echo "IP地址格式正确"; }else{ echo "IP地址格式错误"; }
- Integer verification
In some scenarios, it is necessary to perform integer verification on the data input by the user. You can use the is_numeric function in PHP to determine whether a value is a number:
$number = "123"; if(is_numeric($number)){ echo "输入的值是一个整数"; }else{ echo "输入的值不是一个整数"; }
- Length limit
When storing some sensitive information, it is often necessary to limit the length of the input data. The strlen function in PHP can easily obtain the length of the string and then perform limit verification:
$password = "123456"; if(strlen($password) < 6){ echo "密码长度太短"; }else{ echo "密码长度合适"; }
- Database query verification
For some scenarios that require querying the database for verification , you can use PHP's database operation function to determine whether the data exists or meets the requirements:
$username = "test"; // 假设连接数据库的代码已经完成 $sql = "SELECT * FROM users WHERE username = '$username'"; $result = mysqli_query($connection, $sql); if(mysqli_num_rows($result) > 0){ echo "用户名已存在"; }else{ echo "用户名可用"; }
It should be noted that the above code only provides a simple example and needs to be modified according to specific needs in actual development. .
In actual development, data verification is a key step to ensure application security. By using the built-in functions provided by PHP and some common verification techniques, you can easily verify the data format, length and legality, thereby avoiding the storage and use of erroneous data. I hope this article can be helpful to readers.
The above is the detailed content of How to use PHP functions to verify data storage and reading?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


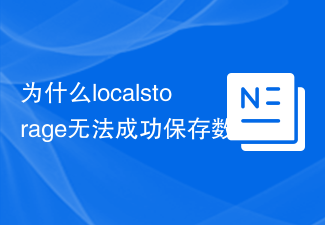
Why does storing data to localstorage always fail? Need specific code examples In front-end development, we often need to store data on the browser side to improve user experience and facilitate subsequent data access. Localstorage is a technology provided by HTML5 for client-side data storage. It provides a simple way to store data and maintain data persistence after the page is refreshed or closed. However, when we use localstorage for data storage, sometimes
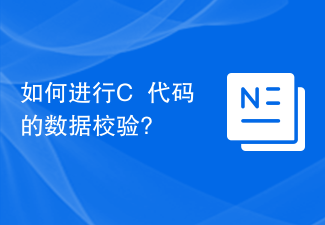
How to perform data verification on C++ code? Data verification is a very important part when writing C++ code. By verifying the data entered by the user, the robustness and security of the program can be enhanced. This article will introduce some common data verification methods and techniques to help readers effectively verify data in C++ code. Input data type check Before processing the data input by the user, first check whether the type of the input data meets the requirements. For example, if you need to receive integer input from the user, you need to ensure that the user input is
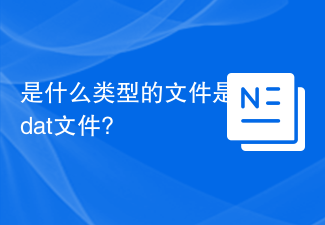
The dat file is a universal data file format that can be used to store various types of data. dat files can contain different data forms such as text, images, audio, and video. It is widely used in many different applications and operating systems. dat files are typically binary files that store data in bytes rather than text. This means that dat files cannot be modified or their contents viewed directly through a text editor. Instead, specific software or tools are required to process and parse the data of dat files. d
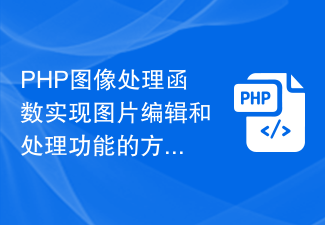
PHP image processing functions are a set of functions specifically used to process and edit images. They provide developers with rich image processing functions. Through these functions, developers can implement operations such as cropping, scaling, rotating, and adding watermarks to images to meet different image processing needs. First, I will introduce how to use PHP image processing functions to achieve image cropping function. PHP provides the imagecrop() function, which can be used to crop images. By passing the coordinates and size of the cropping area, we can crop the image
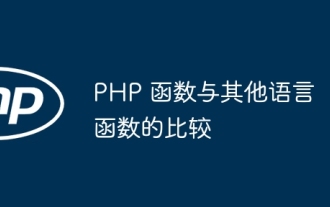
PHP functions have similarities with functions in other languages, but also have some unique features. Syntactically, PHP functions are declared with function, JavaScript is declared with function, and Python is declared with def. In terms of parameters and return values, PHP functions accept parameters and return a value. JavaScript and Python also have similar functions, but the syntax is different. In terms of scope, functions in PHP, JavaScript and Python all have global or local scope. Global functions can be accessed from anywhere, and local functions can only be accessed within their declaration scope.
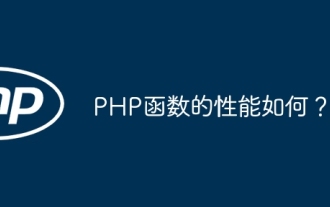
The performance of different PHP functions is crucial to application efficiency. Functions with better performance include echo and print, while functions such as str_replace, array_merge, and file_get_contents have slower performance. For example, the str_replace function is used to replace strings and has moderate performance, while the sprintf function is used to format strings. Performance analysis shows that it only takes 0.05 milliseconds to execute one example, proving that the function performs well. Therefore, using functions wisely can lead to faster and more efficient applications.

The main differences between PHP and Flutter functions are declaration, syntax and return type. PHP functions use implicit return type conversion, while Flutter functions explicitly specify return types; PHP functions can specify optional parameters through ?, while Flutter functions use required and [] to specify required and optional parameters; PHP functions use = to pass naming Parameters, while Flutter functions use {} to specify named parameters.
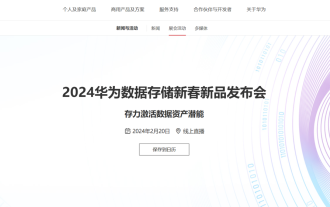
According to news from this site on February 11, according to Huawei official news, the 2024 Huawei Data Storage New Year New Product Launch Conference will be held on February 20. Attached to this site is a conference introduction: Data is an important production factor in the digital economy era, a key source of value creation, and a national strategic resource. Data infrastructure plays a key supporting role in the supply, circulation and application of data elements. It is responsible for reliable storage and efficient management of data assets and their flow according to demand. As an important part of the national data strategy, data infrastructure is the cornerstone of realizing a data power. Huawei continues to innovate in the field of ICT infrastructure, develops advanced data storage capabilities, and plays a fundamental role in ensuring that data assets are “securely stored, readily available, mobile, and well used.” At the same time, Huawei insists on developing
