Use the PHP function 'mysqli_query' to execute MySQL queries
Use PHP function "mysqli_query" to execute MySQL queries
MySQL is a widely used relational database management system. When developing Web applications, it is often necessary to perform various query operations. As a commonly used server-side programming language, PHP provides a variety of functions to connect and operate the MySQL database. Among them, the "mysqli_query" function is one of the commonly used functions to perform query operations.
The "mysqli_query" function can execute various types of MySQL query statements, including SELECT, INSERT, UPDATE, DELETE, etc. It accepts two parameters, the first parameter is the database connection object, and the second parameter is the query statement to be executed. The following is a sample code that uses the "mysqli_query" function to execute a SELECT query:
<?php // 创建数据库连接 $connection = mysqli_connect('localhost', 'username', 'password', 'database'); // 检查连接是否成功 if (!$connection) { die('数据库连接失败: ' . mysqli_connect_error()); } // 执行SELECT查询 $query = "SELECT id, name, age FROM users"; $result = mysqli_query($connection, $query); // 检查查询是否成功 if (!$result) { die('查询失败: ' . mysqli_error($connection)); } // 处理查询结果 while ($row = mysqli_fetch_assoc($result)) { echo 'ID: ' . $row['id'] . ', 姓名: ' . $row['name'] . ', 年龄: ' . $row['age'] . '<br>'; } // 释放结果集 mysqli_free_result($result); // 关闭数据库连接 mysqli_close($connection); ?>
The above code first creates a database connection object and checks whether the connection is successful. Then, a SELECT query is executed using the "mysqli_query" function, and the query results are saved in the $result variable. Next, loop through the data in the $result variable and print out the ID, name, and age of each row. Finally, the query result set is released and the database connection is closed.
In addition to executing SELECT queries, the "mysqli_query" function can also execute other types of queries. For example, you can use this function to execute an INSERT statement to insert data into the database, use an UPDATE statement to update data in the database, use a DELETE statement to delete data in the database, and so on. Just pass in the corresponding SQL statement as the second parameter of the "mysqli_query" function.
It should be noted that when the "mysqli_query" function executes a query statement, it returns a result set object. By traversing this result set object, you can obtain each row of data in the query results. You can use "mysqli_fetch_assoc", "mysqli_fetch_row", "mysqli_fetch_array" and other functions to obtain different forms of query results.
In summary, it is very convenient and fast to use the PHP function "mysqli_query" to execute MySQL queries. Whether you are performing SELECT, INSERT, UPDATE or DELETE operations, you only need to pass in the corresponding query statement to easily operate the database.
The above is the detailed content of Use the PHP function 'mysqli_query' to execute MySQL queries. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


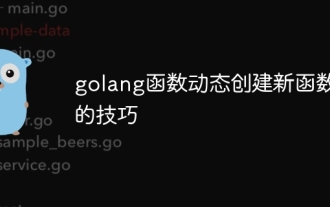
Go language provides two dynamic function creation technologies: closure and reflection. closures allow access to variables within the closure scope, and reflection can create new functions using the FuncOf function. These technologies are useful in customizing HTTP routers, implementing highly customizable systems, and building pluggable components.
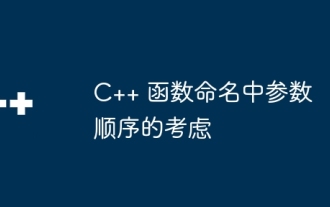
In C++ function naming, it is crucial to consider parameter order to improve readability, reduce errors, and facilitate refactoring. Common parameter order conventions include: action-object, object-action, semantic meaning, and standard library compliance. The optimal order depends on the purpose of the function, parameter types, potential confusion, and language conventions.
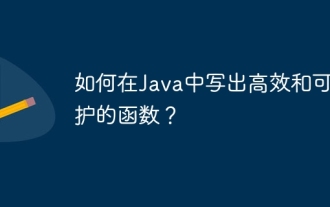
The key to writing efficient and maintainable Java functions is: keep it simple. Use meaningful naming. Handle special situations. Use appropriate visibility.
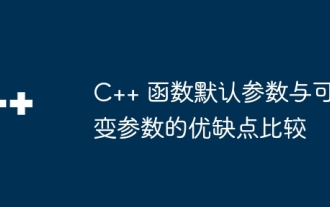
The advantages of default parameters in C++ functions include simplifying calls, enhancing readability, and avoiding errors. The disadvantages are limited flexibility and naming restrictions. Advantages of variadic parameters include unlimited flexibility and dynamic binding. Disadvantages include greater complexity, implicit type conversions, and difficulty in debugging.
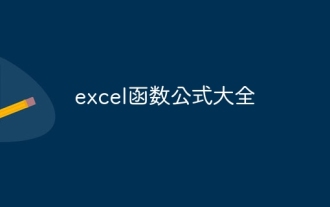
1. The SUM function is used to sum the numbers in a column or a group of cells, for example: =SUM(A1:J10). 2. The AVERAGE function is used to calculate the average of the numbers in a column or a group of cells, for example: =AVERAGE(A1:A10). 3. COUNT function, used to count the number of numbers or text in a column or a group of cells, for example: =COUNT(A1:A10) 4. IF function, used to make logical judgments based on specified conditions and return the corresponding result.
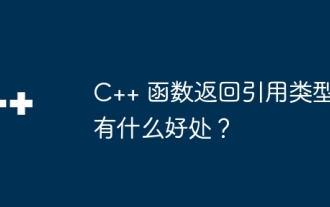
The benefits of functions returning reference types in C++ include: Performance improvements: Passing by reference avoids object copying, thus saving memory and time. Direct modification: The caller can directly modify the returned reference object without reassigning it. Code simplicity: Passing by reference simplifies the code and requires no additional assignment operations.
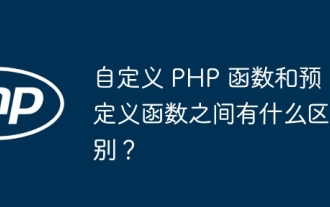
The difference between custom PHP functions and predefined functions is: Scope: Custom functions are limited to the scope of their definition, while predefined functions are accessible throughout the script. How to define: Custom functions are defined using the function keyword, while predefined functions are defined by the PHP kernel. Parameter passing: Custom functions receive parameters, while predefined functions may not require parameters. Extensibility: Custom functions can be created as needed, while predefined functions are built-in and cannot be modified.
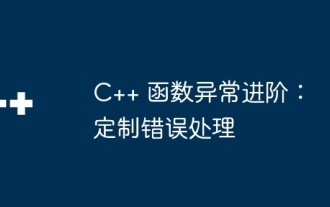
Exception handling in C++ can be enhanced through custom exception classes that provide specific error messages, contextual information, and perform custom actions based on the error type. Define an exception class inherited from std::exception to provide specific error information. Use the throw keyword to throw a custom exception. Use dynamic_cast in a try-catch block to convert the caught exception to a custom exception type. In the actual case, the open_file function throws a FileNotFoundException exception. Catching and handling the exception can provide a more specific error message.
