How to connect to Oracle database using PDO
How to use PDO to connect to Oracle database
Overview:
PDO (PHP Data Objects) is an extension library for operating databases in PHP. It provides a unified API to access multiple types of database. In this article, we will discuss how to use PDO to connect to an Oracle database and perform some common database operations.
Steps:
- Install the Oracle database driver extension
Before using PDO to connect to the Oracle database, we need to install the corresponding Oracle database driver extension. On Windows, we can enable the extension by editing the php.ini file and uncommenting the following line:;extension=php_pdo_oci.dll
;extension=php_oci8.dll
Then restart the Apache or Nginx server.
On Linux, we need to use the PECL command or manually compile and install the OCI8 or PDO_OCI extension. The specific installation process may vary according to different operating systems and PHP versions. We can refer to PHP official documentation or related forums for installation. -
Create database connection
In the PHP program, we can use the following code to create a PDO object to connect to the Oracle database:$dsn = 'oci:dbname=//hostname:port/oracle_sid'; $username = 'your_username'; $password = 'your_password'; try { $conn = new PDO($dsn, $username, $password); } catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage(); exit; }
Copy after loginIn the code, $dsn Is a string containing the host name, port and Oracle SID. $username and $password are the username and password required to connect to the Oracle database.
Execute SQL Query
Once we successfully connect to the Oracle database, we can use the PDO object to execute the SQL query. The following is a simple example that demonstrates how to query data in the database and output the results to the browser:$sql = 'SELECT * FROM employees'; $stmt = $conn->query($sql); $result = $stmt->fetchAll(PDO::FETCH_ASSOC); foreach ($result as $row) { echo $row['employee_id'] . ' ' . $row['first_name'] . ' ' . $row['last_name'] . '<br>'; }
Copy after loginIn the code, $sql is the SELECT statement we want to execute, and $stmt is a PDOStatement object , which represents the result set of the query. By calling the fetchAll method of the PDOStatement object, you can obtain an associative array containing the query results. We can then use a foreach loop to iterate through the results and output the data for each row to the browser.
Execute prepared statements
In addition to ordinary SQL queries, PDO also supports executing prepared statements. Prepared statements can not only improve the performance of database operations, but also prevent SQL injection attacks. The following is a sample code that uses prepared statements to query the database:$sql = 'SELECT * FROM employees WHERE department_id = :dept_id'; $stmt = $conn->prepare($sql); $stmt->bindParam(':dept_id', $dept_id); $dept_id = 1; $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC); foreach ($result as $row) { echo $row['employee_id'] . ' ' . $row['first_name'] . ' ' . $row['last_name'] . '<br>'; }
Copy after loginIn the code, we use placeholders (:dept_id) to represent query conditions, and then use the bindParam method to bind the placeholders to the actual value. Next, we can execute the query by executing the execute method and save the results into the $result variable.
Summary:
Through the above steps, we can use PDO to successfully connect to the Oracle database and perform some common database operations. When developing PHP applications, using PDO to connect to the database not only improves performance, but also provides better security. I hope this article will help you understand how to use PDO to connect to an Oracle database.
The above is the detailed content of How to connect to Oracle database using PDO. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


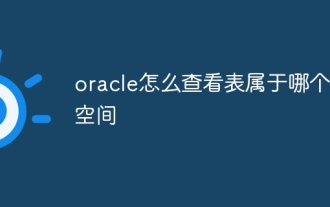
How to check which table space a table belongs to in Oracle: 1. Use the "SELECT" statement and specify the table name to find the table space to which the specified table belongs; 2. Use the database management tools provided by Oracle to check the table space to which the table belongs. Tools usually provide a graphical interface, making the operation more intuitive and convenient; 3. In SQL*Plus, you can view the table space to which the table belongs by entering the "DESCRIBEyour_table_name;" command.
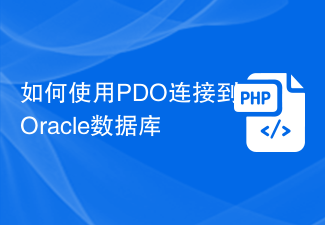
Overview of how to use PDO to connect to Oracle database: PDO (PHPDataObjects) is an extension library for operating databases in PHP. It provides a unified API to access multiple types of databases. In this article, we will discuss how to use PDO to connect to an Oracle database and perform some common database operations. Step: Install the Oracle database driver extension. Before using PDO to connect to the Oracle database, we need to install the corresponding Oracle
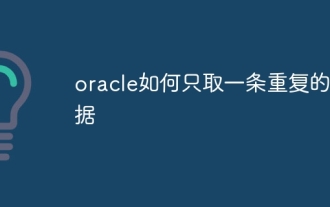
Steps for Oracle to fetch only one piece of duplicate data: 1. Use the SELECT statement combined with the GROUP BY and HAVING clauses to find duplicate data; 2. Use ROWID to delete duplicate data to ensure that accurate duplicate data records are deleted, or use "ROW_NUMBER" ()" function to delete duplicate data, which will delete all records except the first record in each set of duplicate data; 3. Use the "select count(*) from" statement to return the number of deleted records to ensure the result.
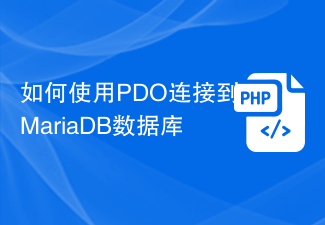
How to use PDO to connect to MariaDB database 1. Introduction PDO (PHPDataObjects) is a lightweight abstraction layer used in PHP to access the database. It provides developers with a unified set of interfaces to connect and operate different types of databases, including MariaDB, MySQL, SQLite, etc. This article will introduce how to use PDO to connect to the MariaDB database and give sample code. 2. Install and configure using PDO to connect to MariaDB
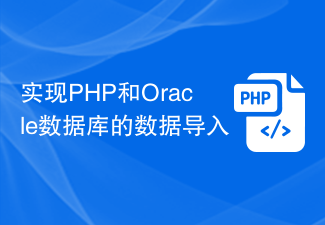
Implementing data import into PHP and Oracle databases In web development, using PHP as a server-side scripting language can conveniently operate the database. As a common relational database management system, Oracle database has powerful data storage and processing capabilities. This article will introduce how to use PHP to import data into an Oracle database and give corresponding code examples. First, we need to ensure that PHP and Oracle database have been installed, and that PHP has been configured to
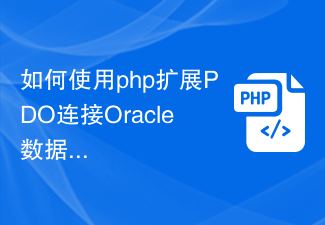
How to use PHP to extend PDO to connect to Oracle database Introduction: PHP is a very popular server-side programming language, and Oracle is a commonly used relational database management system. This article will introduce how to use PHP extension PDO (PHPDataObjects) to connect to Oracle database. 1. Install the PDO_OCI extension. To connect to the Oracle database, you first need to install the PDO_OCI extension. Here are the steps to install the PDO_OCI extension: Make sure
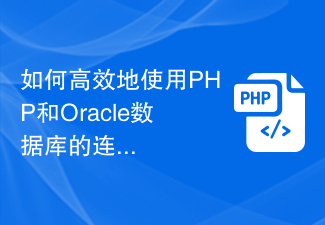
How to efficiently use connection pooling in PHP and Oracle databases Introduction: When developing PHP applications, using a database is an essential part. When interacting with Oracle databases, the use of connection pools is crucial to improving application performance and efficiency. This article will introduce how to use Oracle database connection pool efficiently in PHP and provide corresponding code examples. 1. The concept and advantages of connection pooling Connection pooling is a technology for managing database connections. It creates a batch of connections in advance and maintains a
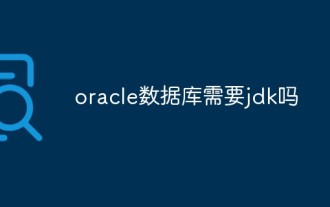
The oracle database requires jdk. The reasons are: 1. When using specific software or functions, other software or libraries included in the JDK are required; 2. Java JDK needs to be installed to run Java programs in the Oracle database; 3. JDK provides Develop and compile Java application functions; 4. Meet Oracle's requirements for Java functions to help implement and implement specific functions.
