How to implement failover and retry of requests in FastAPI
How to implement request failure recovery and retry in FastAPI
Introduction:
In developing web applications, we often need to communicate with other services. However, these services may experience failures, such as temporary network outages or response timeouts. To keep our applications reliable, we need to recover from failures and retry when necessary. In this article, we will learn how to implement failover and retry of requests in FastAPI.
FastAPI is a modern web framework based on Python that provides simple and efficient request processing and routing functions. It uses an asynchronous method internally to process requests, which makes it easier to implement failure recovery and retries.
Implementation ideas for fault recovery and retry:
Before implementing the fault recovery and retry functions, we first need to understand some basic concepts.
- Circuit Breaker mode:
The circuit breaker is a design pattern that can prevent the spread of faults. When a service fails, the circuit breaker temporarily closes requests to the service for a certain period of time and returns the requests directly. This avoids further load on the failed service and gives the service some time to recover. When no fault occurs within a certain period of time, the circuit breaker will automatically recover and forward the request to the service again. - Retry mechanism:
The retry mechanism is to resend the same request when the request fails, usually adding some delay time. The retry mechanism can ensure the reliability of the request and resend the request after failure recovery.
Based on the above ideas, we can achieve request failure recovery and retry by using the circuit breaker mode and retry mechanism.
Implementation steps:
The following code example demonstrates how to implement failure recovery and retry of requests in FastAPI:
from fastapi import FastAPI import requests app = FastAPI() @app.get("/retry") def retry(): url = "http://example.com/api" # 要请求的URL max_retries = 3 # 最大重试次数 retry_interval = 1 # 重试间隔时间(秒) retries = 0 while retries <= max_retries: try: response = requests.get(url) return response.json() except requests.exceptions.RequestException as error: print(f"Request failed: {error}") retries += 1 time.sleep(retry_interval) return {"message": "Max retries exceeded"} @app.get("/circuit-breaker") def circuit_breaker(): url = "http://example.com/api" # 要请求的URL breaker = Breaker(url, max_failures=3, reset_timeout=10) try: response = breaker.execute(requests.get) return response.json() except BreakerOpenError: return {"message": "Service temporarily unavailable"} class Breaker: def __init__(self, url, max_failures, reset_timeout): self.url = url self.max_failures = max_failures self.reset_timeout = reset_timeout self.failures = 0 self.last_failure = None def execute(self, func): if self.failures >= self.max_failures: if self.last_failure and time.time() - self.last_failure < self.reset_timeout: raise BreakerOpenError() else: self.reset() try: response = func(self.url) self.failures = 0 return response except requests.exceptions.RequestException as error: print(f"Request failed: {error}") self.failures += 1 self.last_failure = time.time() def reset(self): self.failures = 0 self.last_failure = None class BreakerOpenError(Exception): pass
In the above code, we use retry
and circuit_breaker
are two routing examples to implement request failure recovery and retry. retry
The routing example uses a while loop to retry. When the request fails, it will wait for a period of time and then resend the request. circuit_breaker
The routing example uses a custom Circuit Breaker class, which will throw a custom BreakerOpenError exception after reaching the maximum number of failures.
Conclusion:
In this article, we learned how to implement failure recovery and retry of requests in FastAPI. By using the circuit breaker pattern and the retry mechanism, we can improve the reliability of the application and recover from failures. At the same time, we also demonstrated how to implement failure recovery and retry functions in FastAPI through sample code. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of How to implement failover and retry of requests in FastAPI. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


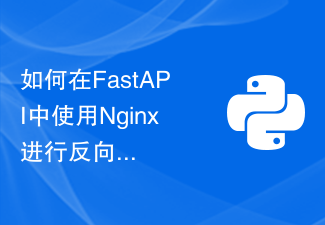
How to use Nginx with FastAPI for reverse proxy and load balancing Introduction: FastAPI and Nginx are two very popular web development tools. FastAPI is a high-performance Python framework, and Nginx is a powerful reverse proxy server. Using these two tools together can improve the performance and reliability of your web applications. In this article, we will learn how to use Nginx with FastAPI for reverse proxy and load balancing. What is reverse generation
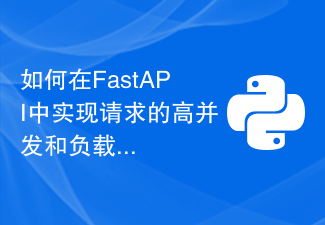
How to achieve high concurrency and load balancing of requests in FastAPI Introduction: With the development of the Internet, high concurrency of web applications has become a common problem. When handling a large number of requests, we need to use efficient frameworks and technologies to ensure system performance and scalability. FastAPI is a high-performance Python framework that can help us achieve high concurrency and load balancing. This article will introduce how to use FastAPI to achieve high concurrency and load balancing of requests. We will use Python3.7
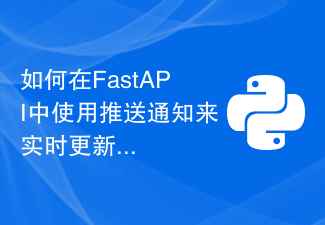
How to use push notifications in FastAPI to update data in real time Introduction: With the continuous development of the Internet, real-time data updates are becoming more and more important. For example, in application scenarios such as real-time trading, real-time monitoring, and real-time gaming, we need to update data in a timely manner to provide the most accurate information and the best user experience. FastAPI is a modern Python-based web framework that provides a simple and efficient way to build high-performance web applications. This article will introduce how to use FastAPI to implement
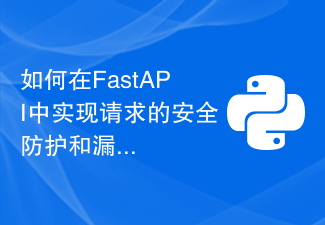
How to implement request security protection and vulnerability repair in FastAPI Introduction: In the process of developing web applications, it is very important to ensure the security of the application. FastAPI is a fast (high-performance), easy-to-use, Python web framework with automatic documentation generation. This article will introduce how to implement request security protection and vulnerability repair in FastAPI. 1. Use the secure HTTP protocol. Using the HTTPS protocol is the basis for ensuring application communication security. FastAPI provides
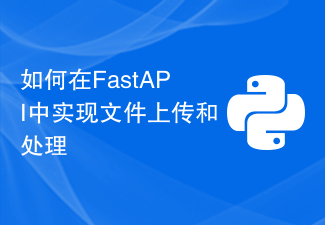
How to implement file upload and processing in FastAPI FastAPI is a modern, high-performance web framework that is easy to use and powerful. It provides native support for file upload and processing. In this article, we will learn how to implement file upload and processing functions in the FastAPI framework, and provide code examples to illustrate specific implementation steps. First, we need to import the required libraries and modules: fromfastapiimportFastAPI,UploadF
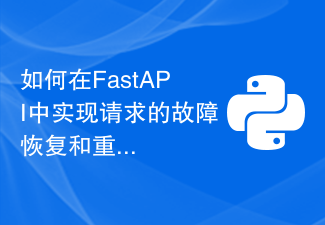
How to implement request failure recovery and retry in FastAPI Introduction: In developing web applications, we often need to communicate with other services. However, these services may experience failures, such as temporary network outages or response timeouts. To keep our applications reliable, we need to recover from failures and retry when necessary. In this article, we will learn how to implement failover and retry of requests in FastAPI. FastAPI is a modern web application based on Python
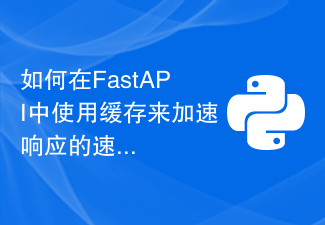
How to use caching in FastAPI to speed up responses Introduction: In modern web development, performance is an important concern. If our application cannot respond to customer requests quickly, it may lead to a decline in user experience or even user churn. Using cache is one of the common methods to improve the performance of web applications. In this article, we will explore how to use caching to speed up the response speed of the FastAPI framework and provide corresponding code examples. 1. What is cache? A cache is a cache that will be accessed frequently

Introduction to how to implement load balancing and high availability in FastAPI: With the development of Internet applications, the requirements for system load balancing and high availability are getting higher and higher. FastAPI is a high-performance Python-based web framework that provides a simple and powerful way to build, deploy and scale web applications. This article will introduce how to implement load balancing and high availability in FastAPI and provide corresponding code examples. Using Nginx to achieve load balancingNginx is a popular
