


Symfony framework middleware: provides error handling and exception management functions
Symfony framework middleware: Provides error handling and exception management functions
When we develop applications, we often encounter errors and exceptions. In order to optimize user experience and provide better developer tools, the Symfony framework provides powerful error handling and exception management functions. In this article, we will introduce the use of Symfony framework middleware and sample code.
The error handling and exception management functions in the Symfony framework are mainly implemented through middleware. Middleware is a special functional component that handles requests and responses before they reach the controller or before the response is returned to the client. Middleware can be used for a variety of purposes, including error and exception handling.
First, we need to install and configure the Symfony framework in order to use the middleware function. You can install the Symfony framework through Composer, using the following command:
composer require symfony/symfony
After the installation is complete, we need to add middleware to the application's entry file. In the Symfony framework, middleware can be managed through the Kernel. Add the following code in the public/index.php
file:
use AppKernel; use SymfonyComponentDebugDebug; use SymfonyComponentDotenvDotenv; use SymfonyComponentHttpFoundationRequest; require dirname(__DIR__).'/config/bootstrap.php'; if ($_SERVER['APP_DEBUG']) { umask(0000); Debug::enable(); } $kernel = new Kernel($_SERVER['APP_ENV'], (bool) $_SERVER['APP_DEBUG']); $request = Request::createFromGlobals(); $response = $kernel->handle($request); $response->send(); $kernel->terminate($request, $response);
In the above code, we use the Kernel
class to initialize the kernel of the application. We then create a Request
object and send it to the kernel for processing. Finally, we send the response to the client and terminate the kernel.
Next, we can use middleware to handle errors and exceptions. In the Symfony framework, we can use FaultTolerantMiddleware
middleware to catch and handle errors and exceptions. In the src/Kernel.php
file, add the following code:
use SymfonyBundleFrameworkBundleKernelMicroKernelTrait; use SymfonyComponentDependencyInjectionContainerBuilder; use SymfonyComponentHttpFoundationResponse; use SymfonyComponentHttpKernelExceptionHttpExceptionInterface; use SymfonyComponentHttpKernelKernel as BaseKernel; use SymfonyComponentRoutingRouteCollectionBuilder; class Kernel extends BaseKernel { use MicroKernelTrait; protected function configureContainer(ContainerBuilder $c, LoaderInterface $loader) { // 配置服务容器 } protected function configureRoutes(RouteCollectionBuilder $routes) { // 配置路由 } protected function build(ContainerBuilder $container) { $container->register('middleware.fault_tolerant', FaultTolerantMiddleware::class) ->setAutowired(true); } protected function renderException(Throwable $exception): Response { if ($exception instanceof HttpExceptionInterface) { $statusCode = $exception->getStatusCode(); } else { $statusCode = Response::HTTP_INTERNAL_SERVER_ERROR; } $response = new Response(); $response->setStatusCode($statusCode); $response->setContent($exception->getMessage()); return $response; } }
In the above code, we registered the FaultTolerantMiddleware
middleware and set its automatic injection to real. Then, we override the renderException
method, which is used to handle the exception and return the response.
Now, we can demonstrate the use of middleware through sample code. Let's say our application has a route that handles user login requests. In the src/Controller/LoginController.php
file, add the following code:
use SymfonyComponentHttpFoundationRequest; use SymfonyComponentHttpFoundationResponse; use SymfonyComponentRoutingAnnotationRoute; class LoginController { /** * @Route("/login", name="login", methods={"POST"}) */ public function login(Request $request): Response { $username = $request->request->get('username'); $password = $request->request->get('password'); if ($username === 'admin' && $password === 'secret') { return new Response('Login successful'); } else { throw new Exception('Invalid username or password'); } } }
In the above code, we have created a controller method that handles user login requests. If the username and password are correct, we return a successful response; otherwise, we throw an exception.
When the user sends a login request, the middleware will catch the exception and call the renderException
method to handle the exception and return the corresponding response to the client. In the above example, if the username and password are incorrect, we will return a response with an error message.
Summary:
This article introduces the use of Symfony framework middleware and sample code. By using middleware, we can easily handle errors and exceptions and return appropriate responses to the client. The Symfony framework provides powerful error handling and exception management functions to make our applications more stable and reliable.
The above is the detailed content of Symfony framework middleware: provides error handling and exception management functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


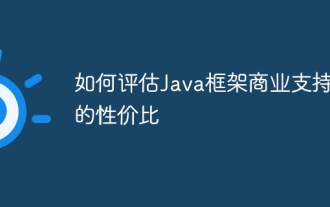
Evaluating the cost/performance of commercial support for a Java framework involves the following steps: Determine the required level of assurance and service level agreement (SLA) guarantees. The experience and expertise of the research support team. Consider additional services such as upgrades, troubleshooting, and performance optimization. Weigh business support costs against risk mitigation and increased efficiency.

The lightweight PHP framework improves application performance through small size and low resource consumption. Its features include: small size, fast startup, low memory usage, improved response speed and throughput, and reduced resource consumption. Practical case: SlimFramework creates REST API, only 500KB, high responsiveness and high throughput
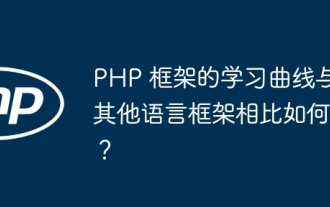
The learning curve of a PHP framework depends on language proficiency, framework complexity, documentation quality, and community support. The learning curve of PHP frameworks is higher when compared to Python frameworks and lower when compared to Ruby frameworks. Compared to Java frameworks, PHP frameworks have a moderate learning curve but a shorter time to get started.
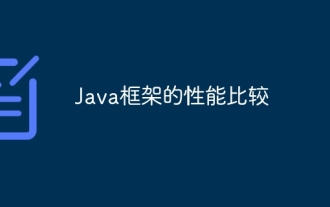
According to benchmarks, for small, high-performance applications, Quarkus (fast startup, low memory) or Micronaut (TechEmpower excellent) are ideal choices. SpringBoot is suitable for large, full-stack applications, but has slightly slower startup times and memory usage.
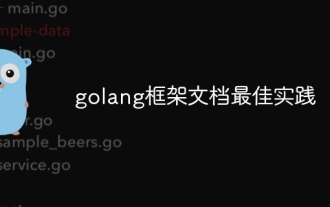
Writing clear and comprehensive documentation is crucial for the Golang framework. Best practices include following an established documentation style, such as Google's Go Coding Style Guide. Use a clear organizational structure, including headings, subheadings, and lists, and provide navigation. Provides comprehensive and accurate information, including getting started guides, API references, and concepts. Use code examples to illustrate concepts and usage. Keep documentation updated, track changes and document new features. Provide support and community resources such as GitHub issues and forums. Create practical examples, such as API documentation.
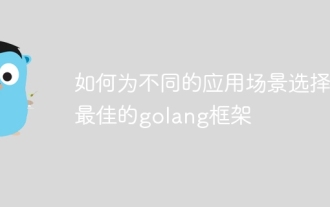
Choose the best Go framework based on application scenarios: consider application type, language features, performance requirements, and ecosystem. Common Go frameworks: Gin (Web application), Echo (Web service), Fiber (high throughput), gorm (ORM), fasthttp (speed). Practical case: building REST API (Fiber) and interacting with the database (gorm). Choose a framework: choose fasthttp for key performance, Gin/Echo for flexible web applications, and gorm for database interaction.
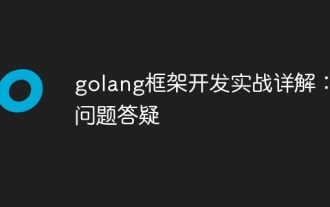
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
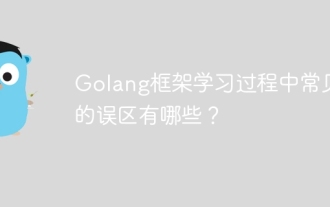
There are five misunderstandings in Go framework learning: over-reliance on the framework and limited flexibility. If you don’t follow the framework conventions, the code will be difficult to maintain. Using outdated libraries can cause security and compatibility issues. Excessive use of packages obfuscates code structure. Ignoring error handling leads to unexpected behavior and crashes.
