


How to implement load balancing and high availability in FastAPI
How to achieve load balancing and high availability in FastAPI
Introduction:
With the development of Internet applications, the requirements for system load balancing and high availability are getting higher and higher. FastAPI is a high-performance Python-based web framework that provides a simple and powerful way to build, deploy and scale web applications. This article will introduce how to implement load balancing and high availability in FastAPI and provide corresponding code examples.
- Use Nginx to achieve load balancing
Nginx is a popular high-performance web server and reverse proxy server that can be used to achieve load balancing. Load balancing can be achieved by specifying proxy servers for multiple FastAPI applications in the Nginx configuration and using a load balancing algorithm to distribute requests. The following is an example configuration file using Nginx to implement load balancing:
http { upstream fastapi { server 127.0.0.1:8000; server 127.0.0.1:8001; } server { listen 80; location / { proxy_pass http://fastapi; } } }
In the above configuration, we specified the proxy server addresses of two FastAPI applications and used the default polling algorithm to distribute requests. By adding more proxy servers to the Nginx configuration, more advanced load balancing algorithms can be implemented, such as weighted polling, IP hashing, etc.
- Using Docker to achieve high availability
Docker is a popular containerization platform that can help us achieve high availability. By packaging FastAPI applications as Docker images and using Docker Swarm or Kubernetes to manage container clusters, container-level failure recovery and automatic scaling can be achieved. The following is an example command to use Docker Swarm to achieve high availability:
# 创建Docker服务 $ docker service create --name fastapi --replicas 3 -p 8000:8000 my_fastapi_image:latest
The above command will create a service containing 3 FastAPI application containers and use port 8000 for load balancing. When a container fails, Docker Swarm will automatically reschedule the container to ensure high availability of the service.
In addition, by using Docker Compose, we can easily define and manage relationships between multiple services. The following is an example configuration file using Docker Compose to define a FastAPI service and an Nginx load balancer:
version: '3' services: fastapi: build: . ports: - 8000:8000 nginx: image: nginx ports: - 80:80 volumes: - ./nginx.conf:/etc/nginx/nginx.conf
In the above configuration, we have defined a service cluster that contains a FastAPI application and an Nginx load balancer.
Conclusion:
By using Nginx for load balancing and Docker for high availability, it can help us build FastAPI applications with high performance and scalability. As Internet applications develop, these technologies will become increasingly important. I hope the introduction and sample code in this article can help readers better apply it in practice.
The above is the detailed content of How to implement load balancing and high availability in FastAPI. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


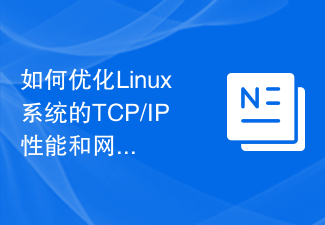
In the field of modern computers, the TCP/IP protocol is the basis for network communication. As an open source operating system, Linux has become the preferred operating system used by many businesses and organizations. However, as network applications and services become more and more critical components of business, administrators often need to optimize network performance to ensure fast and reliable data transfer. This article will introduce how to improve the network transmission speed of Linux systems by optimizing TCP/IP performance and network performance of Linux systems. This article will discuss a
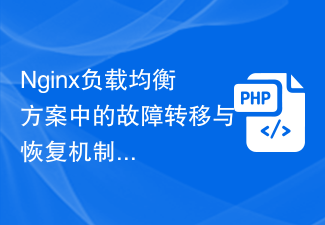
Introduction to the failover and recovery mechanism in the Nginx load balancing solution: For high-load websites, the use of load balancing is one of the important means to ensure high availability of the website and improve performance. As a powerful open source web server, Nginx's load balancing function has been widely used. In load balancing, how to implement failover and recovery mechanisms is an important issue that needs to be considered. This article will introduce the failover and recovery mechanism in Nginx load balancing and give specific code examples. 1. Failover mechanism
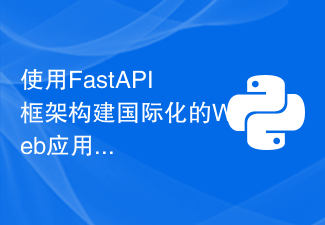
Use the FastAPI framework to build international Web applications. FastAPI is a high-performance Python Web framework that combines Python type annotations and high-performance asynchronous support to make developing Web applications simpler, faster, and more reliable. When building an international Web application, FastAPI provides convenient tools and concepts that can make the application easily support multiple languages. Below I will give a specific code example to introduce how to use the FastAPI framework to build
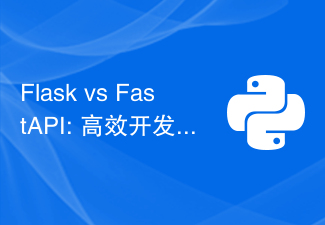
FlaskvsFastAPI: The best choice for efficient development of WebAPI Introduction: In modern software development, WebAPI has become an indispensable part. They provide data and services that enable communication and interoperability between different applications. When choosing a framework for developing WebAPI, Flask and FastAPI are two choices that have attracted much attention. Both frameworks are very popular and each has its own advantages. In this article, we will look at Fl
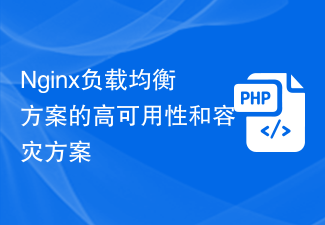
High Availability and Disaster Recovery Solution of Nginx Load Balancing Solution With the rapid development of the Internet, the high availability of Web services has become a key requirement. In order to achieve high availability and disaster tolerance, Nginx has always been one of the most commonly used and reliable load balancers. In this article, we will introduce Nginx’s high availability and disaster recovery solutions and provide specific code examples. High availability of Nginx is mainly achieved through the use of multiple servers. As a load balancer, Nginx can distribute traffic to multiple backend servers to
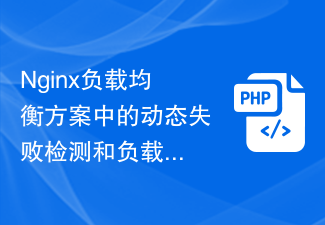
Dynamic failure detection and load weight adjustment strategies in the Nginx load balancing solution require specific code examples. Introduction In high-concurrency network environments, load balancing is a common solution that can effectively improve the availability and performance of the website. Nginx is an open source, high-performance web server that provides powerful load balancing capabilities. This article will introduce two important features in Nginx load balancing, dynamic failure detection and load weight adjustment strategy, and provide specific code examples. 1. Dynamic failure detection Dynamic failure detection
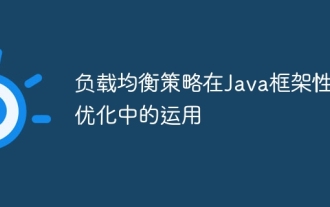
Load balancing strategies are crucial in Java frameworks for efficient distribution of requests. Depending on the concurrency situation, different strategies have different performance: Polling method: stable performance under low concurrency. Weighted polling method: The performance is similar to the polling method under low concurrency. Least number of connections method: best performance under high concurrency. Random method: simple but poor performance. Consistent Hashing: Balancing server load. Combined with practical cases, this article explains how to choose appropriate strategies based on performance data to significantly improve application performance.
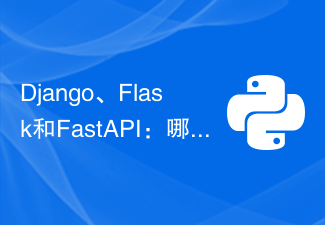
Django, Flask, and FastAPI: Which framework is right for beginners? Introduction: In the field of web application development, there are many excellent Python frameworks to choose from. This article will focus on the three most popular frameworks, Django, Flask and FastAPI. We will evaluate their features and discuss which framework is best for beginners to use. At the same time, we will also provide some specific code examples to help beginners better understand these frameworks. 1. Django: Django
