


Symfony framework middleware: strengthening application security protection measures
Symfony framework middleware: Strengthening application security protection measures
Introduction:
With the rapid development of the Internet, network security issues have become increasingly prominent, and application security protection measures have become very important. . As a popular PHP development framework, the Symfony framework provides a variety of security features and tools, one of which is middleware. This article will introduce the basic concepts and usage of Symfony framework middleware and provide some code examples.
1. What is middleware?
1.1 Definition of middleware:
Middleware is a software design pattern similar to a filter, a series of processing procedures executed between the request and the response. Each middleware is able to examine the request and corresponding content, and then decide whether to proceed to the next middleware. This pattern can be used to implement various application functions, such as logging, permission verification, caching, etc.
1.2 Characteristics of middleware:
- Can execute multiple middleware in sequence to implement complex processing logic.
- Data can be processed between the request and the response, and the data can be modified, verified or filtered.
- Different middleware can be flexibly combined to meet different business needs.
2. Middleware in the Symfony framework
The Symfony framework has introduced the concept of middleware since version 4.3. It regards middleware as a pluggable component that can be accessed through a simple Configuration and code implement the functions of middleware.
2.1 Middleware configuration
In the Symfony framework, middleware is defined through configuration files. The configuration options for the middleware can be found in the config/packages/framework.yaml
file. The following is a sample configuration:
framework: middleware: - AppMiddlewareMyMiddleware1 - AppMiddlewareMyMiddleware2
In the above configuration, AppMiddlewareMyMiddleware1
and AppMiddlewareMyMiddleware2
respectively represent two custom middleware classes.
2.2 Writing middleware
By inheriting the SymfonyComponentHttpKernelHttpKernelInterface
interface and implementing the handle
method, you can define a middleware class. The following is a simple example middleware class:
namespace AppMiddleware; use SymfonyComponentHttpFoundationRequest; use SymfonyComponentHttpFoundationResponse; use SymfonyComponentHttpKernelHttpKernelInterface; class MyMiddleware1 implements HttpKernelInterface { private $app; public function __construct(HttpKernelInterface $app) { $this->app = $app; } public function handle(Request $request, $type = HttpKernelInterface::MASTER_REQUEST, $catch = true) { // 在请求处理之前执行的逻辑 $response = $this->app->handle($request, $type, $catch); // 在请求处理之后执行的逻辑 return $response; } }
In the above example middleware class, MyMiddleware1
will execute some logic before and after request processing.
2.3 Use of middleware
To use middleware, we need to register the middleware into the kernel of the Symfony framework. This can be achieved by adding a middleware class to the middleware configuration in the config/packages/framework.yaml
file. The example is as follows:
framework: middleware: - AppMiddlewareMyMiddleware1 - AppMiddlewareMyMiddleware2
The above example will execute the middleware in the configured order. In the handle
method of each middleware, customized processing logic can be performed, such as permission verification, logging, etc.
3. Application scenarios of middleware
Middleware is a very flexible design pattern that can be applied to various applications. In the Symfony framework, middleware can be used to enhance application security protection measures.
3.1 Permission verification middleware
A common application scenario is permission verification. By writing a middleware, we can verify the user's permissions before each request reaches the application. If validation fails, an error response can be returned.
The following is a simple permission verification middleware example:
namespace AppMiddleware; use SymfonyComponentHttpFoundationRequest; use SymfonyComponentHttpFoundationResponse; use SymfonyComponentHttpKernelHttpKernelInterface; class AuthMiddleware implements HttpKernelInterface { private $app; public function __construct(HttpKernelInterface $app) { $this->app = $app; } public function handle(Request $request, $type = HttpKernelInterface::MASTER_REQUEST, $catch = true) { // 进行权限验证逻辑 if (!$this->isAuthenticated($request)) { return new Response('Unauthorized', 401); } return $this->app->handle($request, $type, $catch); } private function isAuthenticated(Request $request) { // 检查用户是否已验证 // 假设该方法将返回一个布尔值 } }
In the above example, the AuthMiddleware
middleware will be based on the custom isAuthenticated
The method performs permission verification on the request. If the verification fails, an unauthorized error response is returned, otherwise the request continues to be processed.
3.2 Logging middleware
Another application scenario is logging. By writing a middleware, you can log request and response information before and after each request reaches your application.
The following is a simple logging middleware example:
namespace AppMiddleware; use SymfonyComponentHttpFoundationRequest; use SymfonyComponentHttpFoundationResponse; use SymfonyComponentHttpKernelHttpKernelInterface; class LogMiddleware implements HttpKernelInterface { private $app; public function __construct(HttpKernelInterface $app) { $this->app = $app; } public function handle(Request $request, $type = HttpKernelInterface::MASTER_REQUEST, $catch = true) { // 在请求处理之前记录请求的信息 $this->logRequest($request); $response = $this->app->handle($request, $type, $catch); // 在请求处理之后记录响应的信息 $this->logResponse($response); return $response; } private function logRequest(Request $request) { // 记录请求的信息到日志文件或其他媒体 } private function logResponse(Response $response) { // 记录响应的信息到日志文件或其他媒体 } }
In the above example, the LogMiddleware
middleware will be called before and after the request is processed. The logRequest
and logResponse
methods record request and response information.
Conclusion:
Middleware in the Symfony framework is a powerful tool that can be used to strengthen application security measures. By using middleware, we can easily implement functions such as permission verification and logging, and flexibly configure multiple middleware to meet different business needs. We hope that the middleware concepts and sample code provided in this article will be helpful to your application development in the Symfony framework.
The above is the detailed content of Symfony framework middleware: strengthening application security protection measures. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


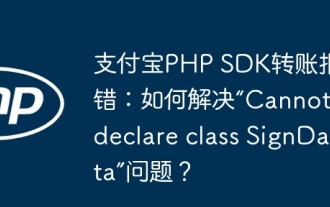
Alipay PHP...
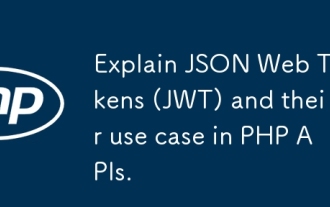
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
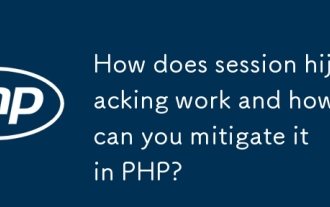
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
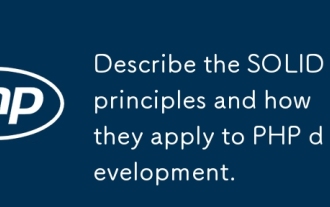
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
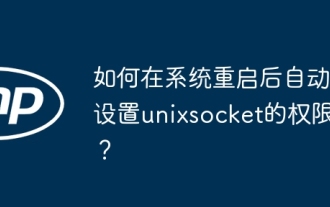
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
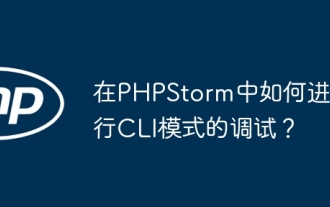
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
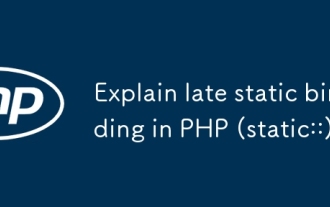
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
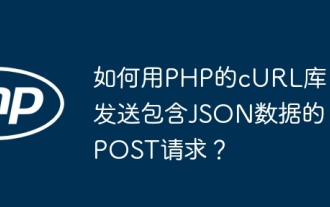
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
