


How to use the database query builder (Query Builder) in the CakePHP framework to perform multi-table join queries
How to use the database query builder (Query Builder) in the CakePHP framework to perform multi-table join queries
When developing web applications, using a database for data storage and retrieval is a very common requirement. In practical applications, there are often correlations between different data tables, so multi-table joint queries are required.
The CakePHP framework provides a powerful database query builder (Query Builder) function, making multi-table joint queries simple and efficient. This article will introduce how to use the database query builder in the CakePHP framework to perform multi-table join queries, as well as code examples of some common operations.
- Configuring the database connection
First, we need to configure the database connection in the CakePHP configuration file. Open the config/app.php
file and find the default
configuration item under the Datasources
array. In this configuration item, set the relevant information of the database as follows:
'Datasources' => [ 'default' => [ 'className' => 'CakeDatabaseConnection', 'driver' => 'CakeDatabaseDriverMysql', 'database' => 'your_database_name', 'username' => 'your_database_username', 'password' => 'your_database_password', 'host' => 'localhost', 'port' => '3306', 'encoding' => 'utf8', 'timezone' => 'UTC', 'cacheMetadata' => true, // ... ], // ... ],
Configure accordingly according to the actual situation.
- Create model
In the CakePHP framework, each data table corresponds to a model (Model). We need to create the corresponding model file in the src/Model
directory.
Suppose we have two data tables: users
and posts
, corresponding to users and articles respectively. First, create the src/Model/User.php
file with the following code:
<?php namespace AppModel; use CakeORMTable; class UserTable extends Table { }
Then, create the src/Model/Post.php
file with the following code:
<?php namespace AppModel; use CakeORMTable; class PostTable extends Table { }
In this way, we created two model files and associated them with the corresponding data tables.
- Perform multi-table joint query
Next, we will use the database query builder to perform multi-table joint query. We assume that we need to query the latest article published by each user.
First, add the following code in the src/Controller/UsersController.php
file to load the relevant model:
<?php namespace AppController; use AppControllerAppController; use AppModelUser; use AppModelPost; class UsersController extends AppController { public function index() { $users = $this->Users->find() ->contain(['Posts']) ->where(['Posts.id' => function($query) { return $query->select(['max' => $query->func()->max('id')]) ->from(['Posts' => 'posts']) ->where(['Posts.user_id = Users.id']); }]) ->all(); $this->set(compact('users')); } }
In the above code, we use The $this->Users->find()
method starts building the query. Then, use the $this->Users->find()->contain(['Posts'])
method to tell the query builder to associate the query Posts
model.
Next, we use the ->where()
method to set the query conditions, which uses a subquery to filter the latest article of each user. This subquery uses the ->select()
method to select the largest id
value, and uses the ->from()
method to specify the query The data table and its alias, and the association conditions are set using the ->where()
method.
Finally, we use the ->all()
method to get the query results, and use the $this->set()
method to pass the query results to the view .
- Display query results in the view
Finally, we add the following code in the src/Template/Users/index.ctp
file to Display the query results in the view:
<h1>List of Users and Their Latest Posts</h1> <table> <tr> <th>User ID</th> <th>User Name</th> <th>Latest Post</th> </tr> <?php foreach ($users as $user): ?> <tr> <td><?= $user->id ?></td> <td><?= $user->name ?></td> <td><?= $user->posts[0]->title ?></td> </tr> <?php endforeach; ?> </table>
In the above code, we use foreach
to loop through the query results and display the ID, name and title of the latest article for each user come out.
So far, we have completed the example operation of using the database query builder to perform multi-table join queries in the CakePHP framework. Through concise and efficient code, we can easily implement complex multi-table query requirements, improving development efficiency and code maintainability.
Summary
This article introduces how to use the database query builder in the CakePHP framework to perform multi-table joint queries. By configuring database connections, creating models, and using the query builder for multi-table queries, we can easily implement complex data query needs. I hope this article can help you perform multi-table joint queries in the CakePHP framework.
The above is the detailed content of How to use the database query builder (Query Builder) in the CakePHP framework to perform multi-table join queries. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
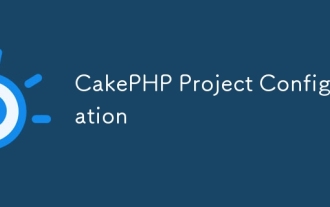
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
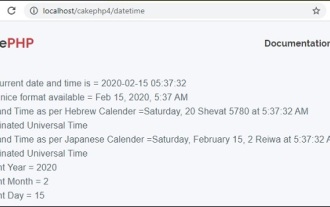
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
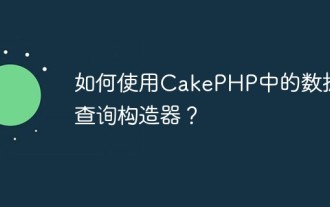
CakePHP is an open source PHPMVC framework which is widely used in web application development. CakePHP has many features and tools, including a powerful database query builder for interactive performance databases. This query builder allows you to execute SQL queries using object-oriented syntax without having to write cumbersome SQL statements. This article will introduce how to use the database query builder in CakePHP. Establishing a database connection Before using the database query builder, you first need to create a database connection in Ca
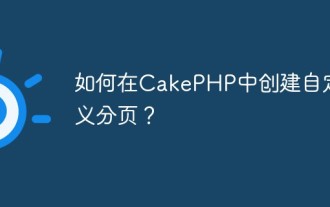
CakePHP is a powerful PHP framework that provides developers with many useful tools and features. One of them is pagination, which helps us divide large amounts of data into several pages, making browsing and manipulation easier. By default, CakePHP provides some basic pagination methods, but sometimes you may need to create some custom pagination methods. This article will show you how to create custom pagination in CakePHP. Step 1: Create a custom pagination class First, we need to create a custom pagination class. this
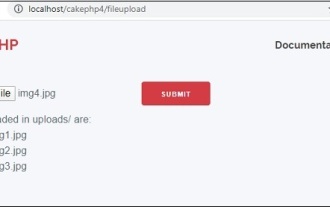
To work on file upload we are going to use the form helper. Here, is an example for file upload.
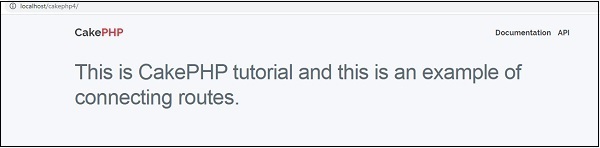
In this chapter, we are going to learn the following topics related to routing ?
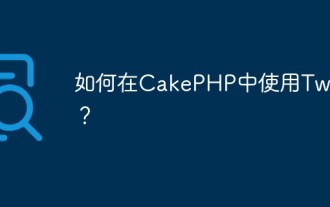
Using Twig in CakePHP is a way to separate templates and views, making the code more modular and maintainable. This article will introduce how to use Twig in CakePHP. 1. Install Twig. First install the Twig library in the project. You can use Composer to complete this task. Run the following command in the console: composerrequire "twig/twig:^2.0" This command will be displayed in the project's vendor
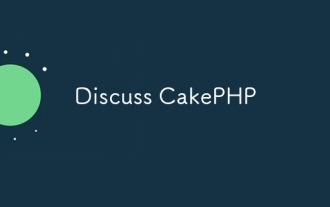
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
