


Using Slim framework middleware to implement international SMS sending and receiving functions
Using Slim framework middleware to implement international SMS sending and receiving functions
In modern society, SMS has become one of the important communication tools in people's daily lives. With the increase in international exchanges, the function of sending and receiving international text messages has also received increasing attention. This article will introduce how to use the Slim framework middleware to implement the function of sending and receiving international text messages.
Slim is a lightweight PHP micro-framework that provides simple and powerful routing functions and is very suitable for rapid development of small API applications. At the same time, Slim also supports the use of middleware to implement additional functional extensions.
First, we need to install the Slim framework in the project. You can use Composer to install, open a terminal and execute the following command:
1 |
|
After the installation is complete, we can create a simple Slim application. First, create a file named index.php
and add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
In the above code, we created a Slim application and defined two routes, respectively Used to send and receive text messages. Now, we need to write the logic code for sending and receiving text messages.
The logic code for sending text messages can be implemented using the API of a third-party SMS service provider. Here, we take Twilio as an example to demonstrate how to send text messages. First, install Twilio's PHP SDK and execute the following command in the terminal:
1 |
|
After the installation is completed, we can add the logic code for sending SMS in the index.php
file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
In the above code, we use Twilio’s PHP SDK to send text messages. First, you need to replace Your_Account_SID
, Your_Auth_Token
, and Your_Twilio_Number
with your own Twilio account information. We then get the recipient number and SMS content from the request parameters and send the SMS using Twilio's API.
Next, we need to write the logic code for receiving text messages. There are many logical implementation methods for receiving SMS messages. You can use the API provided by the SMS gateway or use SMS hardware devices to receive them. Here, we take receiving and saving text messages to the database as an example to demonstrate how to receive text messages. First, create a SQLite database named sms.db
, and create a table named messages
, containing id
, sender_number
and message
fields. Then, we can add the logic code for receiving text messages in the index.php
file:
1 2 3 4 5 6 7 8 9 10 11 12 |
|
In the above code, we first obtain the sender number and text message content from the request parameters. Then, use the SQLite3 library to save the SMS to the database.
So far, we have completed the code example of using the Slim framework middleware to implement the international SMS sending and receiving function. When we access the /send-sms
route and pass in the recipient number and text message content, the text message will be sent to the specified number. When we send a text message to the /receive-sms
route, the text message will be received and saved in the database.
In summary, using the Slim framework middleware can easily implement international SMS sending and receiving functions. By combining the use of APIs and databases from third-party SMS service providers, we can implement a simple and powerful international SMS application. Of course, the above code is just an example, and actual application needs to be appropriately modified and optimized according to specific needs.
The above is the detailed content of Using Slim framework middleware to implement international SMS sending and receiving functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
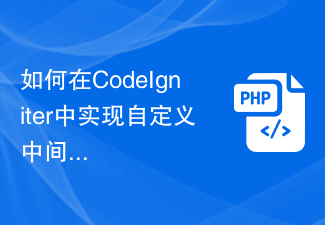
How to implement custom middleware in CodeIgniter Introduction: In modern web development, middleware plays a vital role in applications. They can be used to perform some shared processing logic before or after the request reaches the controller. CodeIgniter, as a popular PHP framework, also supports the use of middleware. This article will introduce how to implement custom middleware in CodeIgniter and provide a simple code example. Middleware overview: Middleware is a kind of request
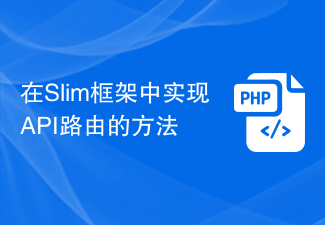
How to implement API routing in the Slim framework Slim is a lightweight PHP micro-framework that provides a simple and flexible way to build web applications. One of the main features is the implementation of API routing, allowing us to map different requests to corresponding handlers. This article will introduce how to implement API routing in the Slim framework and provide some code examples. First, we need to install the Slim framework. The latest version of Slim can be installed through Composer. Open a terminal and
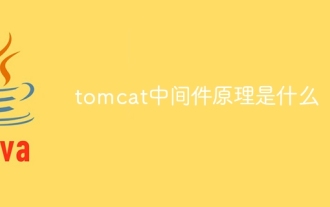
The principle of tomcat middleware is implemented based on Java Servlet and Java EE specifications. As a Servlet container, Tomcat is responsible for processing HTTP requests and responses and providing the running environment for Web applications. The principles of Tomcat middleware mainly involve: 1. Container model; 2. Component architecture; 3. Servlet processing mechanism; 4. Event listening and filters; 5. Configuration management; 6. Security; 7. Clustering and load balancing; 8. Connector technology; 9. Embedded mode, etc.

Implementing user authentication using middleware in the Slim framework With the development of web applications, user authentication has become a crucial feature. In order to protect users' personal information and sensitive data, we need a reliable method to verify the user's identity. In this article, we will introduce how to implement user authentication using the Slim framework’s middleware. The Slim framework is a lightweight PHP framework that provides a simple and fast way to build web applications. One of the powerful features is the middle
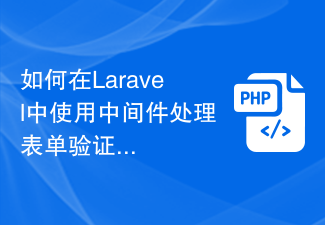
How to use middleware to handle form validation in Laravel, specific code examples are required Introduction: Form validation is a very common task in Laravel. In order to ensure the validity and security of the data entered by users, we usually verify the data submitted in the form. Laravel provides a convenient form validation function and also supports the use of middleware to handle form validation. This article will introduce in detail how to use middleware to handle form validation in Laravel and provide specific code examples.
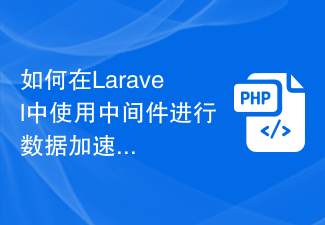
How to use middleware for data acceleration in Laravel Introduction: When developing web applications using the Laravel framework, data acceleration is the key to improving application performance. Middleware is an important feature provided by Laravel that handles requests before they reach the controller or before the response is returned. This article will focus on how to use middleware to achieve data acceleration in Laravel and provide specific code examples. 1. What is middleware? Middleware is a mechanism in the Laravel framework. It is used
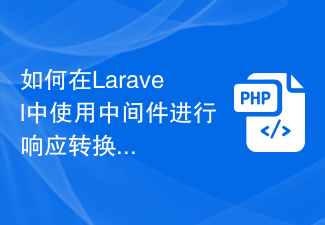
How to use middleware for response conversion in Laravel Middleware is one of the very powerful and practical features in the Laravel framework. It allows us to process requests and responses before the request enters the controller or before the response is sent to the client. In this article, I will demonstrate how to use middleware for response transformation in Laravel. Before starting, make sure you have Laravel installed and a new project created. Now we will follow these steps: Create a new middleware Open
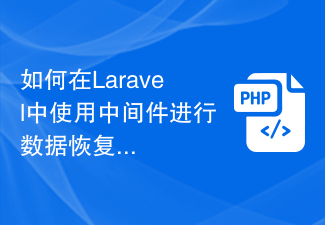
Laravel is a popular PHP web application framework that provides many fast and easy ways to build efficient, secure and scalable web applications. When developing Laravel applications, we often need to consider the issue of data recovery, that is, how to recover data and ensure the normal operation of the application in the event of data loss or damage. In this article, we will introduce how to use Laravel middleware to implement data recovery functions and provide specific code examples. 1. What is Lara?
