


Phalcon middleware: Add task queues and asynchronous processing capabilities to applications
Phalcon middleware: Add task queue and asynchronous processing functions to applications
Introduction:
In modern web applications, task queues and asynchronous processing have become increasingly important. They can help us handle some time-consuming operations and improve application performance and response speed. In the Phalcon framework, we can easily use middleware to implement these functions. This article will introduce how to use middleware in Phalcon to add task queue and asynchronous processing functions, and provide relevant code examples.
- What is middleware?
In Phalcon, middleware is a plug-in mechanism for handling HTTP requests and responses. It can execute some additional code before or after the request reaches the controller. Middleware is great for adding common functionality such as authentication, logging, etc. In this article, we will use middleware to implement task queue and asynchronous processing functions. - Add task queue function
Task queue is a mechanism that stores tasks that need to be delayed in a queue and then executes them one by one according to certain rules. In Phalcon, we can use redis as the storage engine of the task queue. The following is a simple sample code:
use PhalconMvcUserPlugin; use PhalconQueueBeanstalk; use PhalconDiInjectable; class QueuePlugin extends Plugin { private $queue; public function __construct() { $this->queue = new Beanstalk([ 'host' => '127.0.0.1', 'port' => 11300, ]); } public function enqueue($data) { $this->queue->putInTube('tasks', $data); } public function dequeue() { $job = $this->queue->reserveFromTube('tasks'); $this->queue->delete($job); return $job->getBody(); } }
In the above code, we created a class named QueuePlugin, which inherits From Phalcon's Plugin class, and implements the enqueue() and dequeue() methods. The enqueue() method is used to store task data into the task queue, while the dequeue() method is used to obtain and delete a task from the task queue.
- Add asynchronous processing function
Asynchronous processing refers to placing some long-time operations in the background to improve the response speed of the program. In Phalcon, we can use the PhalconAsyncTask class to implement asynchronous processing. The following is a simple sample code:
use PhalconMvcUserPlugin; use PhalconAsyncTask; class AsyncPlugin extends Plugin { private $taskManager; public function __construct() { $this->taskManager = $this->getDI()->getShared('taskManager'); } public function processAsync($data) { $task = new AsyncTask($data); $this->taskManager->execute($task); } }
In the above code, we define a class named AsyncPlugin, which inherits from Phalcon's Plugin class and implements the processAsync() method. The processAsync() method is used to create an asynchronous task and hand it over to the task manager (taskManager) for execution.
- Applying middleware to the application
In order to apply the previously defined middleware to the Phalcon application, we need to configure it accordingly in the application's Bootstrap file. The following is A sample code:
use PhalconDiFactoryDefault; use PhalconMvcApplication; use PhalconEventsManager as EventsManager; $di = new FactoryDefault(); $di->setShared('queuePlugin', function () { return new QueuePlugin(); }); $di->setShared('asyncPlugin', function () { return new AsyncPlugin(); }); $di->setShared('taskManager', function () { return new PhalconAsyncTaskManager(); }); $eventsManager = new EventsManager(); $eventsManager->attach('application:beforeHandleRequest', function ($event, $application) use ($di) { $application->queuePlugin = $di->getShared('queuePlugin'); $application->asyncPlugin = $di->getShared('asyncPlugin'); }); $application = new Application($di); $application->setEventsManager($eventsManager);
In the above code, we created a FactoryDefault object and registered the queuePlugin, asyncPlugin and taskManager services to the dependency injection container. Then, we created an EventsManager object and bound an anonymous function to the application:beforeHandleRequest event. In this anonymous function, we inject queuePlugin and asyncPlugin instances into the application.
Conclusion:
By using Phalcon's middleware function, we can easily add task queue and asynchronous processing functions to the application. The above is a simple sample code, you can extend it according to your actual needs. The use of middleware can not only improve the performance and responsiveness of the application, but also make the code clearer and easier to maintain. I hope this article will help you understand the use of Phalcon middleware.
The above is the detailed content of Phalcon middleware: Add task queues and asynchronous processing capabilities to applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


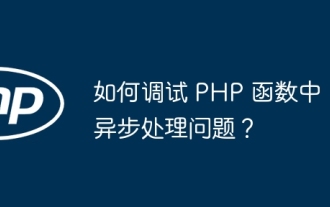
How to debug async processing issues in PHP functions? Use Xdebug to set breakpoints and inspect stack traces, looking for calls related to coroutines or ReactPHP components. Enable ReactPHP debug information and view additional log information, including exceptions and stack traces.

Gin is a web framework based on Go language and is widely used in the field of web development. However, in addition to web development, the Gin framework can also be used to implement other functions, such as task queues and message queues. Task queues and message queues are common components in modern distributed systems for asynchronous processing of data and messages. These queues can be used in scenarios such as peak shaving and valley filling, asynchronous processing of large amounts of data, etc. The task queue pays more attention to the workflow and executes each task in a certain process sequence; while the message queue pays more attention to asynchronous communication.
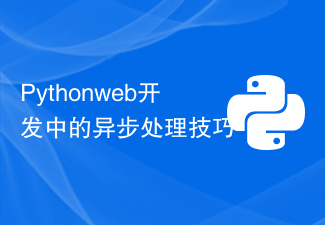
Python is a very popular programming language and is also widely used in the field of web development. With the development of technology, more and more people are beginning to use asynchronous methods to improve website performance. In this article, we will explore asynchronous processing techniques in Python web development. 1. What is asynchronous? Traditional web servers use a synchronous approach to handle requests. When a client initiates a request, the server must wait for the request to complete processing before continuing to process the next request. On high-traffic websites, this same
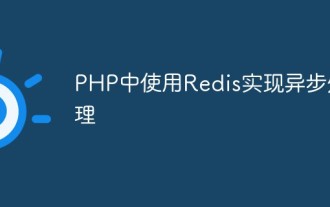
With the development of the Internet, the performance and efficiency of Web applications have become the focus of attention. PHP is a commonly used web development language, and Redis is a popular in-memory database. How to combine the two to improve the performance and efficiency of web applications has become an important issue. Redis is a non-relational in-memory database with the advantages of high performance, high scalability and high reliability. PHP can use Redis to implement asynchronous processing, thereby improving the responsiveness and concurrency of web applications
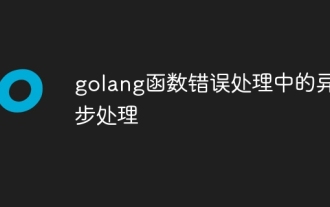
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
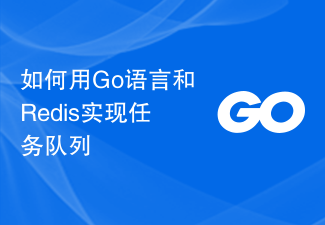
How to implement task queue using Go language and Redis Introduction: In actual software development, we often encounter scenarios where a large number of tasks need to be processed. In order to improve processing efficiency and reliability, we can use task queues to distribute and execute these tasks. This article will introduce how to use Go language and Redis to implement a simple task queue, as well as specific code examples. 1. What is a task queue? Task queue is a common mechanism for distributing and executing tasks. It stores pending tasks in a queue, which are then processed by multiple consumers (also known as
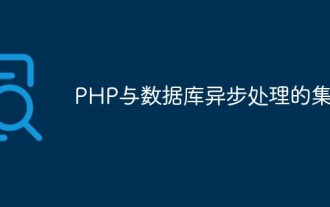
With the continuous development of Internet technology, Web applications have become one of the most important components in the Internet world. As an open source scripting language for web development, PHP is increasingly important in web application development. In most web applications, data processing is an essential link. Databases are one of the most commonly used data storage methods in web applications, so the integration of PHP with databases is a crucial part of web development. As web applications continue to grow in complexity, especially
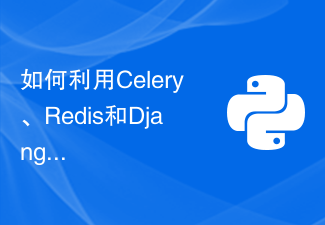
How to implement asynchronous task queue using Celery, Redis and Django Introduction: In web development, it is often necessary to handle some long-term tasks, such as sending emails, generating reports, processing large amounts of data, etc. If these tasks are handled directly in the view function, the request response time will be too long and the user experience will be poor. In order to improve the performance and response speed of the system, we can use asynchronous task queues to handle these time-consuming tasks. Celery is a widely used asynchronous task queue for Python
