


How to use PHP and GMP to perform RSA encryption and decryption algorithms for large integers
How to use PHP and GMP to perform RSA encryption and decryption algorithm for large integers
RSA encryption algorithm is an asymmetric encryption algorithm that is widely used in the field of data security. It implements the process of public key encryption and private key decryption based on two particularly large prime numbers and some simple mathematical operations. In the PHP language, the calculation of large integers can be realized through the GMP (GNU Multiple Precision) library, and the encryption and decryption functions can be realized by combining the RSA algorithm. This article will introduce how to use PHP and GMP libraries to implement RSA encryption and decryption algorithms for large integers, and give corresponding code examples.
1. Generate RSA public and private key pairs
In the RSA algorithm, both the public key and the private key are generated from a pair of large prime numbers. First, we need to generate two large prime numbers $p$ and $q$.
1 2 3 4 5 6 7 8 9 10 |
|
Next, we need to calculate $n$ and $phi(n)$, where $n=pq$, $phi(n)=(p-1)(q-1)$.
1 2 |
|
Then, we choose an integer $e$ as the public key index, satisfying $1
1 |
|
Using the extended Euclidean algorithm, we can calculate the private key index $d$, which satisfies $dequiv e^{-1}pmod{phi(n)}$.
1 2 3 4 5 6 7 8 9 10 11 12 |
|
Finally, we got the RSA public key $(n, e)$ and private key $(n, d)$.
2. Encryption and decryption process
Using the generated public key and private key, we can perform the RSA encryption and decryption process.
1 2 3 4 5 6 7 8 9 10 11 |
|
During the encryption process, we convert the plaintext message into a large integer $msg$, and then use the public key exponent $e$ and the modulus $n$ to calculate to obtain the ciphertext $cipher$. During the decryption process, we convert the ciphertext $cipher$ into a large integer, and then use the private key exponent $d$ and the modulus $n$ to perform calculations to obtain the decrypted plaintext message.
3. Sample code
The following is a complete sample code, including the generation of RSA public and private key pairs and the encryption and decryption process.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
|
The above code implements the RSA encryption and decryption algorithm for large integers using PHP through the GMP library. You can modify the parameters and logic in the code according to your specific needs. Through understanding and practice, I believe everyone can master and flexibly apply this basic cryptographic algorithm.
The above is the detailed content of How to use PHP and GMP to perform RSA encryption and decryption algorithms for large integers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
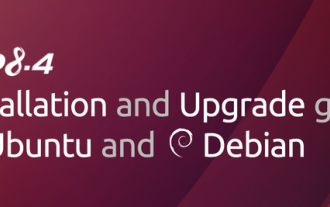
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
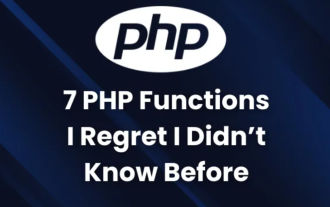
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
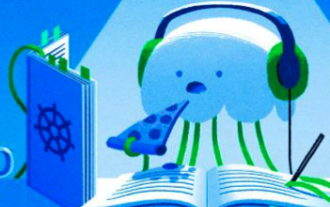
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
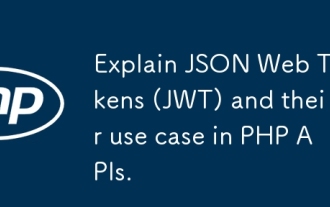
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
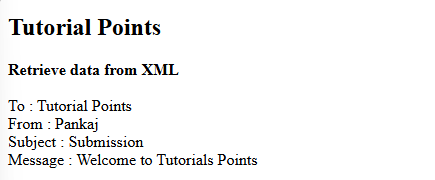
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
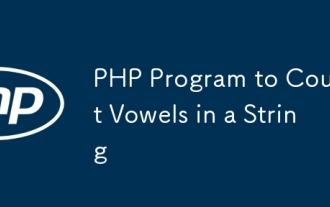
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
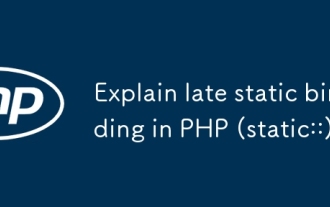
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
