


CodeIgniter middleware: Provides automated cache handling for applications
CodeIgniter middleware: Provide automated caching processing for applications
Introduction:
In the process of developing Web applications, it is very important to optimize the performance of the application. One of the common optimization techniques is caching. Caching can significantly reduce the number of database queries and server load, thereby improving application responsiveness. CodeIgniter provides middleware functions to easily implement automated caching processing. This article will introduce how to use middleware in CodeIgniter to cache the output results of the application.
1. What is middleware?
Middleware is a mechanism that performs some operation between the application processing the request and generating the response. In CodeIgniter, middleware can be used to intercept requests and perform some predefined operations, such as checking user authentication, modifying request parameters, etc.
2. Why use middleware to handle caching?
Using middleware to handle caching can achieve reuse and automation of caching logic. By intercepting the request and checking whether the corresponding data already exists in the cache, middleware can avoid repeatedly querying the database and generating the same response. This can significantly improve application performance and responsiveness.
3. Implement cache middleware in CodeIgniter
The following are the steps and sample code for implementing cache middleware in CodeIgniter:
- Create a file named 'CacheMiddleware' Middleware file and place it in the application's 'application/middleware' directory.
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class CacheMiddleware implements Middleware { private $CI; public function __construct() { $this->CI =& get_instance(); $this->CI->load->driver('cache'); } public function handle(Request $request, Closure $next) { $cacheKey = md5($request->getUri()->getBaseUrl() . $request->getUri()->getPath()); if ($this->CI->cache->get($cacheKey)) { return $this->CI->cache->get($cacheKey); } else { $response = $next($request); $this->CI->cache->save($cacheKey, $response, 3600); // 缓存1小时 return $response; } } } ?>
- Open the 'application/config/autoload.php' file and add the 'cache' library to the automatically loaded library list.
$autoload['libraries'] = array('cache');
- Use caching middleware in the controller.
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class ExampleController extends CI_Controller { public function __construct() { parent::__construct(); $this->middleware(['CacheMiddleware']); } public function index() { $this->output->set_output('Hello, World!'); } } ?>
In the above example, the CacheMiddleware
middleware intercepts the request and checks the corresponding cache. If the corresponding data already exists in the cache, the cache result is returned directly; otherwise, the request continues to be processed and the response result is generated, and the result is stored in the cache.
Middleware can be applied to the entire application or to a specific controller or route. Simply call the $this->middleware(['MiddlewareName'])
method in the constructor to apply middleware to the specified controller.
Conclusion:
CodeIgniter middleware provides developers with a simple and effective way to handle the caching logic of applications. By using middleware to automate caching processing, you can significantly improve your application's performance and responsiveness. Developers can customize middleware as needed to adapt to different caching strategies and needs.
Through the above steps and sample code, I hope readers can successfully implement automated caching processing in their own CodeIgniter applications and improve application performance.
The above is the detailed content of CodeIgniter middleware: Provides automated cache handling for applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


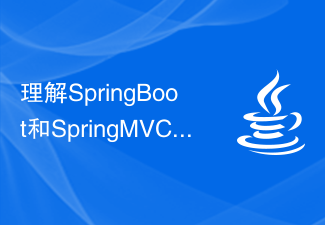
Compare SpringBoot and SpringMVC and understand their differences. With the continuous development of Java development, the Spring framework has become the first choice for many developers and enterprises. In the Spring ecosystem, SpringBoot and SpringMVC are two very important components. Although they are both based on the Spring framework, there are some differences in functions and usage. This article will focus on comparing SpringBoot and Spring
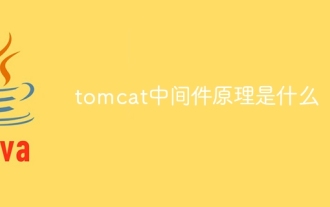
The principle of tomcat middleware is implemented based on Java Servlet and Java EE specifications. As a Servlet container, Tomcat is responsible for processing HTTP requests and responses and providing the running environment for Web applications. The principles of Tomcat middleware mainly involve: 1. Container model; 2. Component architecture; 3. Servlet processing mechanism; 4. Event listening and filters; 5. Configuration management; 6. Security; 7. Clustering and load balancing; 8. Connector technology; 9. Embedded mode, etc.
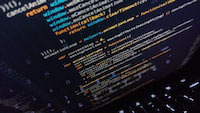
In modern software development, continuous integration (CI) has become an important practice to improve code quality and development efficiency. Among them, Jenkins is a mature and powerful open source CI tool, especially suitable for PHP applications. The following content will delve into how to use Jenkins to implement PHP continuous integration, and provide specific sample code and detailed steps. Jenkins installation and configuration First, Jenkins needs to be installed on the server. Just download and install the latest version from its official website. After the installation is complete, some basic configuration is required, including setting up an administrator account, plug-in installation, and job configuration. Create a new job On the Jenkins dashboard, click the "New Job" button. Select "Frees
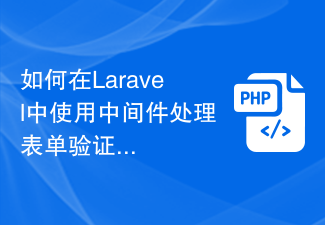
How to use middleware to handle form validation in Laravel, specific code examples are required Introduction: Form validation is a very common task in Laravel. In order to ensure the validity and security of the data entered by users, we usually verify the data submitted in the form. Laravel provides a convenient form validation function and also supports the use of middleware to handle form validation. This article will introduce in detail how to use middleware to handle form validation in Laravel and provide specific code examples.
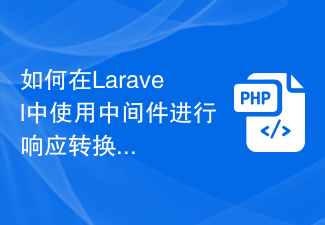
How to use middleware for response conversion in Laravel Middleware is one of the very powerful and practical features in the Laravel framework. It allows us to process requests and responses before the request enters the controller or before the response is sent to the client. In this article, I will demonstrate how to use middleware for response transformation in Laravel. Before starting, make sure you have Laravel installed and a new project created. Now we will follow these steps: Create a new middleware Open
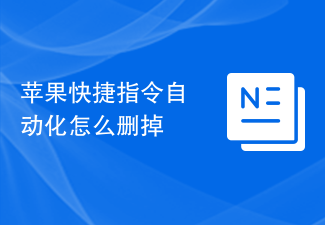
How to Delete Apple Shortcut Automation With the launch of Apple's new iOS13 system, users can use shortcuts (Apple Shortcuts) to customize and automate various mobile phone operations, which greatly improves the user's mobile phone experience. However, sometimes we may need to delete some shortcuts that are no longer needed. So, how to delete Apple shortcut command automation? Method 1: Delete through the Shortcuts app. On your iPhone or iPad, open the "Shortcuts" app. Select in the bottom navigation bar

Laravel is a widely used PHP framework that provides many convenient features and tools, including middleware that supports multiple languages. In this article, we will detail how to use middleware to implement Laravel's multi-language support and provide some specific code examples. Configuring the language pack First, we need to configure Laravel's language pack so that it can support multiple languages. In Laravel, language packages are usually placed in the resources/lang directory, where each language
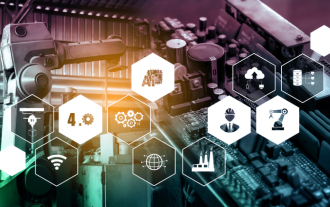
Automation technology is being widely used in different industries, especially in the supply chain field. Today, it has become an important part of supply chain management software. In the future, with the further development of automation technology, the entire supply chain and supply chain management software will undergo major changes. This will lead to more efficient logistics and inventory management, improve the speed and quality of production and delivery, and in turn promote the development and competitiveness of enterprises. Forward-thinking supply chain players are ready to deal with the new situation. CIOs should take the lead in ensuring the best outcomes for their organizations, and understanding the role of robotics, artificial intelligence, and automation in the supply chain is critical. What is supply chain automation? Supply chain automation refers to the use of technological means to reduce or eliminate human participation in supply chain activities. it covers a variety of
