


Zend Framework middleware: Add social login functionality to web applications
Zend Framework is an open source framework based on PHP that provides many powerful tools and components for building scalable web applications. This article will introduce how to use Zend Framework’s middleware to add social login functionality to web applications.
Middleware is a type of code that is executed before or after a request enters the application. It allows developers to customize and extend the process of handling requests. Zend Framework provides a flexible way to define and use middleware.
First, we need to install Zend Framework and related components. Dependencies can be managed using Composer. Run the following command in the command line:
composer require zendframework/zend-expressive zendframework/zend-diactoros
Once the installation is complete, we can create a simple social login middleware. First, create a SocialMiddleware.php
file in the project directory, and then add the following code to the file:
<?php namespace AppMiddleware; use PsrHttpMessageServerRequestInterface as Request; use PsrHttpMessageResponseInterface as Response; use ZendDiactorosResponseRedirectResponse; use ZendExpressiveHelperUrlHelper; class SocialMiddleware { private $urlHelper; public function __construct(UrlHelper $urlHelper) { $this->urlHelper = $urlHelper; } public function __invoke(Request $request, Response $response, callable $next = null) { if (!$this->isLoggedIn()) { // 用户未登录,重定向到登录页面 $loginUrl = $this->urlHelper->generate('login'); return new RedirectResponse($loginUrl); } // 用户已登录,继续处理请求 return $next($request, $response); } private function isLoggedIn() { // 检查用户是否已登录,这里只是一个示例 return isset($_SESSION['user']); } }
The basic idea of the above middleware is as follows:
- Check if the user is logged in, if not, redirect to the login page.
- If you are logged in, continue processing the request.
Next, we need to register the middleware in the application. Open the config/pipeline.php
file and add the following code before return $pipeline;
:
$app->pipe('/admin', AppMiddlewareSocialMiddleware::class);
In the above code, /admin
Indicates the path of the middleware application, AppMiddlewareSocialMiddleware::class
is the class name of the middleware.
Finally, we need to define a route to handle the login logic. Open the config/routes.php
file and add the following code:
$app->get('/login', AppActionLoginAction::class, 'login'); $app->get('/admin/dashboard', AppActionDashboardAction::class, 'dashboard');
The above code maps the /login
path to AppActionLoginAction::class
, map the /admin/dashboard
path to AppActionDashboardAction::class
.
So far, we have completed the code that uses Zend Framework middleware to implement the social login function.
In AppActionLoginAction
we can use an appropriate social login library such as OAuth or OpenID Connect to handle the actual login logic.
In AppActionDashboardAction
, we can render the user's dashboard interface.
To test this feature, we can start the built-in PHP development server:
php -S localhost:8000 -t public/
Then visit http://localhost:8000/admin/dashboard
in the browser .
In short, Zend Framework’s middleware is a powerful tool that can help us easily add social login functionality to web applications. By using an appropriate social login library we can implement user authentication and dashboard functionality.
The above is an introduction to Zend Framework middleware and social login functions. Hope this article helps you!
The above is the detailed content of Zend Framework middleware: Add social login functionality to web applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


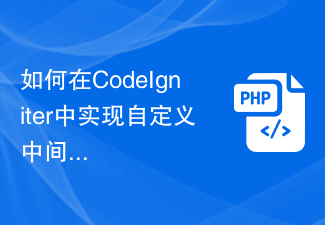
How to implement custom middleware in CodeIgniter Introduction: In modern web development, middleware plays a vital role in applications. They can be used to perform some shared processing logic before or after the request reaches the controller. CodeIgniter, as a popular PHP framework, also supports the use of middleware. This article will introduce how to implement custom middleware in CodeIgniter and provide a simple code example. Middleware overview: Middleware is a kind of request
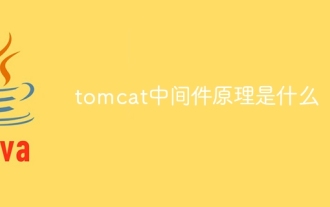
The principle of tomcat middleware is implemented based on Java Servlet and Java EE specifications. As a Servlet container, Tomcat is responsible for processing HTTP requests and responses and providing the running environment for Web applications. The principles of Tomcat middleware mainly involve: 1. Container model; 2. Component architecture; 3. Servlet processing mechanism; 4. Event listening and filters; 5. Configuration management; 6. Security; 7. Clustering and load balancing; 8. Connector technology; 9. Embedded mode, etc.
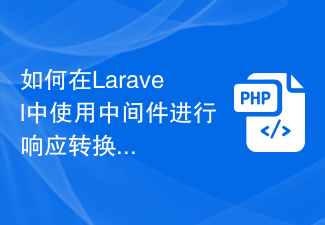
How to use middleware for response conversion in Laravel Middleware is one of the very powerful and practical features in the Laravel framework. It allows us to process requests and responses before the request enters the controller or before the response is sent to the client. In this article, I will demonstrate how to use middleware for response transformation in Laravel. Before starting, make sure you have Laravel installed and a new project created. Now we will follow these steps: Create a new middleware Open

Implementing user authentication using middleware in the Slim framework With the development of web applications, user authentication has become a crucial feature. In order to protect users' personal information and sensitive data, we need a reliable method to verify the user's identity. In this article, we will introduce how to implement user authentication using the Slim framework’s middleware. The Slim framework is a lightweight PHP framework that provides a simple and fast way to build web applications. One of the powerful features is the middle
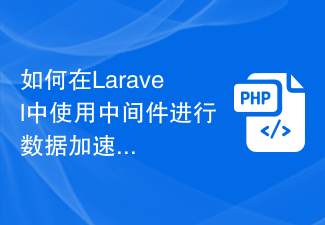
How to use middleware for data acceleration in Laravel Introduction: When developing web applications using the Laravel framework, data acceleration is the key to improving application performance. Middleware is an important feature provided by Laravel that handles requests before they reach the controller or before the response is returned. This article will focus on how to use middleware to achieve data acceleration in Laravel and provide specific code examples. 1. What is middleware? Middleware is a mechanism in the Laravel framework. It is used
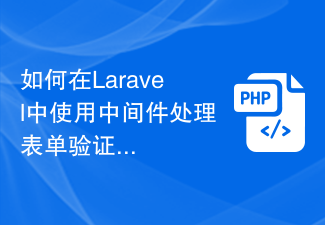
How to use middleware to handle form validation in Laravel, specific code examples are required Introduction: Form validation is a very common task in Laravel. In order to ensure the validity and security of the data entered by users, we usually verify the data submitted in the form. Laravel provides a convenient form validation function and also supports the use of middleware to handle form validation. This article will introduce in detail how to use middleware to handle form validation in Laravel and provide specific code examples.
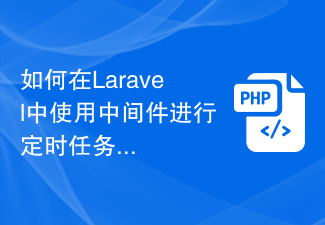
How to use middleware for scheduled task scheduling in Laravel Introduction: Laravel is a popular PHP open source framework that provides convenient and powerful tools to develop web applications. One of the important features is scheduled tasks, which allows developers to run specific tasks at specified intervals. In this article, we will introduce how to use middleware to implement Laravel's scheduled task scheduling, and provide specific code examples. Environment Preparation Before starting, we need to make sure
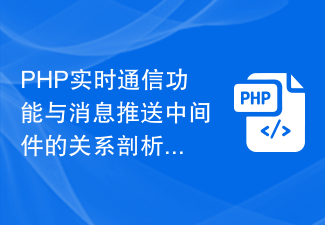
Analysis of the relationship between PHP real-time communication function and message push middleware With the development of the Internet, the importance of real-time communication function in Web applications has become increasingly prominent. Real-time communication allows users to send and receive messages in real-time in applications, and can be applied to a variety of scenarios, such as real-time chat, instant notification, etc. In the field of PHP, there are many ways to implement real-time communication functions, and one of the common ways is to use message push middleware. This article will introduce the relationship between PHP real-time communication function and message push middleware, and how to use message push
