How to use thinkorm to quickly filter and sort data
How to use ThinkORM to quickly implement data filtering and sorting
Introduction:
With the continuous increase of data, quickly finding the required data has become an important task in development. ThinkORM is a powerful and easy-to-use ORM (Object Relational Mapping) tool that can help us quickly filter and sort data. This article describes how to use ThinkORM to filter and sort data, and provides code examples.
1. Install ThinkORM:
First, we need to install ThinkORM. Execute the following command in the command line:
pip install think-orm
2. Connect to the database:
Before starting to use ThinkORM, we need to connect to the database first. Import ThinkORM in the code and create a database connection:
from thinkorm import Database db = Database('mysql', host='localhost', port=3306, user='root', password='password', database='test_db')
The above code uses the MySQL database as an example, you can choose other types of databases according to the actual situation.
3. Define the model:
Next, we need to define the model to map the tables in the database. Suppose we have a table named User
, containing three fields: id
, name
, and age
. We can create a User
class to represent the table:
from thinkorm import Model, Field class User(Model): id = Field(primary_key=True) name = Field() age = Field()
The above code defines a User
class and uses Field
to define it fields in the table.
4. Data filtering:
Using ThinkORM, we can easily implement data filtering. Suppose we want to query users who are older than 18 years old, we can use the following code:
users = db.query(User).filter(User.age > 18).all()
The above code uses the filter()
method to implement data filtering, and its parameter is a conditional expression, That is, filter conditions.
5. Data sorting:
In addition to data filtering, ThinkORM also supports data sorting. Suppose we want to sort the user list in ascending order of age, we can use the following code:
users = db.query(User).order_by(User.age).all()
The above code uses the order_by()
method to implement data sorting, and its parameter is the sorting field.
6. Comprehensive application:
Of course, we can also combine data filtering and sorting. The following is a sample code for a comprehensive application:
users = db.query(User).filter(User.age > 18).order_by(User.age).all()
The above code will first filter out users older than 18 years old, and then sort them in ascending order of age.
Summary:
This article introduces how to use ThinkORM to quickly filter and sort data. First, we need to install and connect ThinkORM. Then, define the model to map the tables in the database. Then, we can use the filter()
method to filter data and the order_by()
method to sort data. Finally, we can combine data filtering and sorting. I hope this article will be helpful to you when using ThinkORM for data processing.
The above is the detailed content of How to use thinkorm to quickly filter and sort data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


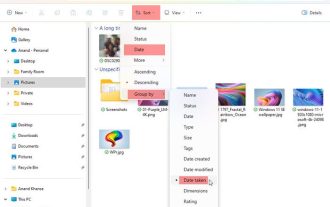
This article will introduce how to sort pictures according to shooting date in Windows 11/10, and also discuss what to do if Windows does not sort pictures by date. In Windows systems, organizing photos properly is crucial to making it easy to find image files. Users can manage folders containing photos based on different sorting methods such as date, size, and name. In addition, you can set ascending or descending order as needed to organize files more flexibly. How to Sort Photos by Date Taken in Windows 11/10 To sort photos by date taken in Windows, follow these steps: Open Pictures, Desktop, or any folder where you place photos In the Ribbon menu, click

Outlook offers many settings and features to help you manage your work more efficiently. One of them is the sorting option that allows you to categorize your emails according to your needs. In this tutorial, we will learn how to use Outlook's sorting feature to organize emails based on criteria such as sender, subject, date, category, or size. This will make it easier for you to process and find important information, making you more productive. Microsoft Outlook is a powerful application that makes it easy to centrally manage your email and calendar schedules. You can easily send, receive, and organize email, while built-in calendar functionality makes it easy to keep track of your upcoming events and appointments. How to be in Outloo
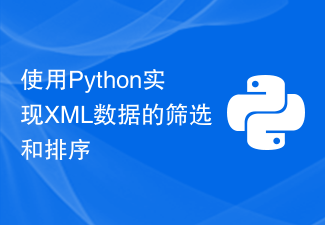
Implementing filtering and sorting of XML data using Python Introduction: XML is a commonly used data exchange format that stores data in the form of tags and attributes. When processing XML data, we often need to filter and sort the data. Python provides many useful tools and libraries to process XML data. This article will introduce how to use Python to filter and sort XML data. Reading the XML file Before we begin, we need to read the XML file. Python has many XML processing libraries,
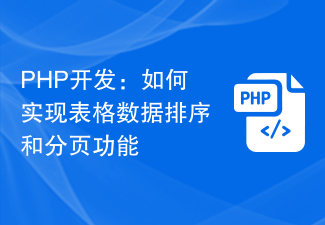
PHP development: How to implement table data sorting and paging functions In web development, processing large amounts of data is a common task. For tables that need to display a large amount of data, it is usually necessary to implement data sorting and paging functions to provide a good user experience and optimize system performance. This article will introduce how to use PHP to implement the sorting and paging functions of table data, and give specific code examples. The sorting function implements the sorting function in the table, allowing users to sort in ascending or descending order according to different fields. The following is an implementation form

How does Arrays.sort() method in Java sort arrays by custom comparator? In Java, the Arrays.sort() method is a very useful method for sorting arrays. By default, this method sorts in ascending order. But sometimes, we need to sort the array according to our own defined rules. At this time, you need to use a custom comparator (Comparator). A custom comparator is a class that implements the Comparator interface.
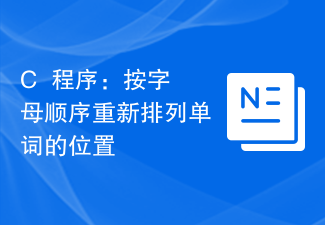
In this problem, a string is given as input and we have to sort the words appearing in the string in lexicographic order. To do this, we assign an index starting from 1 to each word in the string (separated by spaces) and get the output in the form of sorted indices. String={"Hello","World"}"Hello"=1 "World"=2 Since the words in the input string are in lexicographic order, the output will print "12". Let's look at some input/result scenarios - Assuming all words in the input string are the same, let's look at the results - Input:{"hello","hello","hello"}Result:3 Result obtained
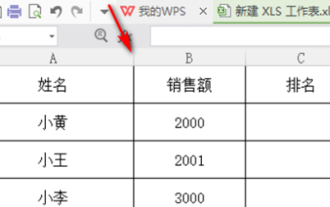
In our work, we often use wps software. There are many ways to process data in wps software, and the functions are also very powerful. We often use functions to find averages, summaries, etc. It can be said that as long as The methods that can be used for statistical data have been prepared for everyone in the WPS software library. Below we will introduce the steps of how to sort the scores in WPS. After reading this, you can learn from the experience. 1. First open the table that needs to be ranked. As shown below. 2. Then enter the formula =rank(B2, B2: B5, 0), and be sure to enter 0. As shown below. 3. After entering the formula, press the F4 key on the computer keyboard. This step is to change the relative reference into an absolute reference.
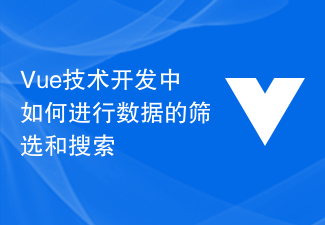
How to filter and search data in Vue technology development In Vue technology development, data filtering and search are very common requirements. Through reasonable data filtering and search functions, users can quickly and easily find the information they need. This article will introduce how to use Vue to implement data filtering and search functions, and give specific code examples. Data filtering: Data filtering refers to filtering data according to specific conditions and filtering out data that meets the conditions. In Vue, you can use the computed attribute and v-for directive
