


PHP mall development skills: Designing shopping cart and order management systems
PHP mall development skills: Designing a shopping cart and order management system
In the development process of a complete PHP mall, the shopping cart and order management system are very important components. The shopping cart is used to allow users to add and manage their purchased items, while the order management system is used to process and track users' orders. Here are some tips for designing a shopping cart and order management system, along with code examples.
1. Design the shopping cart system
- Database design
When starting, you first need to design the shopping cart database structure. You can create a data table named "cart", which contains the following fields:
- cart_id: Shopping cart ID (primary key)
- user_id: User ID, used to communicate with the user Association
- product_id: Product ID, used to associate with the product
- quantity: Product quantity
- created_at: Creation time
- updated_at: Update time
- Add items to the shopping cart
When the user chooses to purchase an item, the item can be added to the shopping cart through the following code:
<?php session_start(); // 创建购物车数组 if(!isset($_SESSION['cart'])){ $_SESSION['cart'] = array(); } // 获取商品详情(这里的商品信息可以从数据库查询得到) $product_id = $_POST['product_id']; $product_name = 'Test Product'; $product_price = 10.00; // 将商品添加到购物车数组 $item = array( 'product_id' => $product_id, 'product_name' => $product_name, 'product_price' => $product_price, 'quantity' => 1 ); array_push($_SESSION['cart'], $item); ?>
- Shopping cart page display
When users browse the shopping cart, they can display the list of products in the shopping cart and the total price of the shopping cart through the following code:
<?php session_start(); // 获取购物车中的商品列表 $cart = $_SESSION['cart']; // 展示购物车中的商品列表 foreach($cart as $item){ echo $item['product_name'].' - '.$item['product_price'].'<br>'; } // 计算购物车总价 $total_price = 0; foreach($cart as $item){ $total_price += $item['product_price'] * $item['quantity']; } echo 'Total Price: '.$total_price; ?>
- Delete items in the shopping cart
When the user wants to delete an item in the shopping cart, he or she can use the following code:
<?php session_start(); // 获取要删除的商品ID $product_id = $_POST['product_id']; // 查找并删除购物车中的对应商品 foreach($_SESSION['cart'] as $key => $item) { if($item['product_id'] == $product_id) { unset($_SESSION['cart'][$key]); break; } } ?>
2. Design order management system
- Database design
In the order management system, the database structure also needs to be designed. You can create a data table named "orders", which contains the following fields:
- order_id: order ID (primary key)
- user_id: user ID, used to associate with the user
- total_price: total order price
- created_at: creation time
- updated_at: update time
- Create order
After the user confirms the purchase of goods, the order information can be inserted into the order management database through the following code:
<?php session_start(); // 获取用户ID和订单总价 $user_id = 1; // 这里假设用户ID为1 $total_price = $_POST['total_price']; // 将订单信息插入到数据库 $conn = mysqli_connect('localhost', 'username', 'password', 'database_name'); $sql = "INSERT INTO orders (user_id, total_price) VALUES ('$user_id', '$total_price')"; mysqli_query($conn, $sql); // 清空购物车 unset($_SESSION['cart']); ?>
- View the order
The user can use the following code Code to view all your orders:
<?php session_start(); // 获取用户ID $user_id = 1; // 这里假设用户ID为1 // 查询数据库中与该用户相关的订单 $conn = mysqli_connect('localhost', 'username', 'password', 'database_name'); $sql = "SELECT * FROM orders WHERE user_id = '$user_id'"; $result = mysqli_query($conn, $sql); // 展示用户的所有订单 while($row = mysqli_fetch_assoc($result)){ echo 'Order ID: '.$row['order_id'].' - Total Price: '.$row['total_price'].'<br>'; } ?>
The above are some tips and code examples for designing shopping cart and order management systems in PHP mall development. I hope this helps you, and I wish you success in developing your mall!
The above is the detailed content of PHP mall development skills: Designing shopping cart and order management systems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


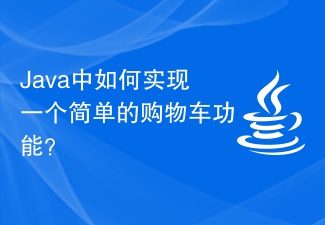
How to implement a simple shopping cart function in Java? The shopping cart is an important feature of an online store, which allows users to add items they want to purchase to the shopping cart and manage the items. In Java, we can implement a simple shopping cart function by using object-oriented approach. First, we need to define a product category. This class contains attributes such as product name, price, and quantity, as well as corresponding Getter and Setter methods. For example: publicclassProduct
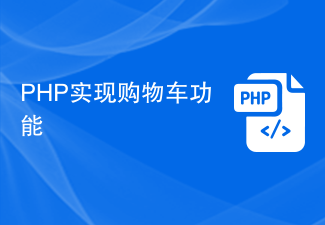
In our daily lives, online shopping has become a very common way of consumption, and the shopping cart function is also one of the important components of online shopping. So, this article will introduce how to use PHP language to implement shopping cart related functions. 1. Technical background The shopping cart is a common function on online shopping websites. When users browse some products on a website, they can add those items to a virtual shopping cart for easy selection and management during the subsequent checkout process. A shopping cart usually includes the following basic functions: Add items:
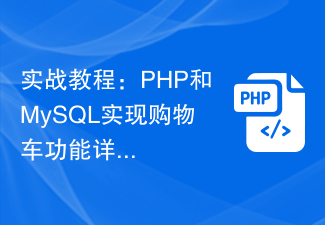
Practical tutorial: Detailed explanation of the shopping cart function with PHP and MySQL. The shopping cart function is one of the common functions in website development. Through the shopping cart, users can easily add the goods they want to buy to the shopping cart, and then proceed with settlement and payment. In this article, we will detail how to implement a simple shopping cart function using PHP and MySQL and provide specific code examples. To create a database and data table, you first need to create a data table in the MySQL database to store product information. The following is a simple data table

PHP mall development skills: Design shopping cart and order synchronization functions In a mall website, shopping cart and orders are indispensable functions. The shopping cart is used for users to purchase products and save them to a temporary shopping cart, while the order is a record generated after the user confirms the purchase of the product. In order to improve user experience and reduce errors, it is very important to design a shopping cart and order synchronization function. 1. The Concept of Shopping Cart and Order A shopping cart is usually a temporary container used to store items purchased by users. Users can add products to the shopping cart for easy browsing and management.
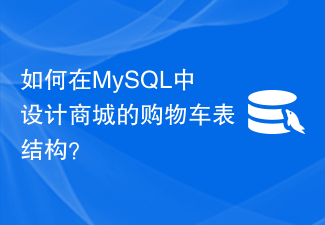
How to design the shopping cart table structure of the mall in MySQL? With the rapid development of e-commerce, shopping carts have become an important part of online malls. The shopping cart is used to save the products purchased by users and related information, providing users with a convenient and fast shopping experience. Designing a reasonable shopping cart table structure in MySQL can help developers store and manage shopping cart data effectively. This article will introduce how to design the shopping cart table structure of the mall in MySQL and provide some specific code examples. First, the shopping cart table should contain
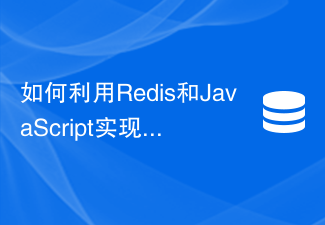
How to use Redis and JavaScript to implement the shopping cart function. The shopping cart is one of the very common functions in e-commerce websites. It allows users to add items of interest to the shopping cart, making it convenient for users to view and manage purchased items at any time. In this article, we will introduce how to implement the shopping cart function using Redis and JavaScript, and provide specific code examples. 1. Preparation Before starting, we need to ensure that Redis has been installed and configured, which can be done through the official website [https:/
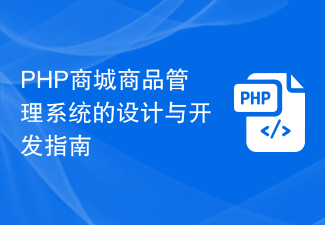
Guide to the Design and Development of PHP Mall Product Management System Summary: This article will introduce how to use PHP to develop a powerful mall product management system. The system includes functions such as adding, editing, deleting, and searching products, as well as product classification management, inventory management, and order management. Through the guide in this article, readers will be able to master the basic processes and techniques of the PHP development mall product management system. Introduction With the rapid development of e-commerce, more and more companies choose to open shopping malls online. As one of the core functions of the mall, the product management system
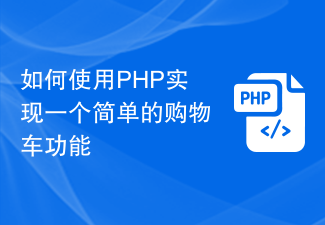
How to use PHP to implement a simple shopping cart function The shopping cart function is an essential part of an e-commerce website. It allows users to add items of interest to the shopping cart, and then proceed to checkout or continue browsing and adding items. This article will introduce how to use PHP to implement a simple shopping cart function and provide specific code examples. Creating the database and tables First, we need to create a database and a table to store the shopping cart data. CREATEDATABASEshopping_ca
