How to parse and generate SOAP messages in PHP
How to parse and generate SOAP messages in PHP
SOAP (Simple Object Access Protocol) is a protocol used to communicate in the network, which allows applications on different platforms to use XML to exchange structured information. In PHP, we can use the built-in SOAP extension to parse and generate SOAP messages.
Before we begin, we need to ensure that our PHP environment has the SOAP extension enabled. This extension can be enabled by uncommenting the line extension=soap
in the php.ini configuration file.
Parsing SOAP messages
To parse SOAP messages, we can use the SoapClient
class in PHP. The following is a simple example that demonstrates how to parse a SOAP message using the SoapClient
class:
// 创建SoapClient对象 $client = new SoapClient("http://example.com/soap-service?wsdl"); // 调用远程SOAP方法 $result = $client->SomeSoapMethod($param1, $param2); // 解析返回的SOAP消息 $response = $client->__getLastResponse(); $parsedResponse = new SimpleXMLElement($response);
In the above code, we first create a SoapClient
object, A URL with SOAP Description Language (WSDL) is used. Then, we called the remote SOAP method and saved the returned SOAP message into the $response
variable. Finally, we use the SimpleXMLElement
class to parse the SOAP message into a simple XML object.
Generating SOAP messages
To generate SOAP messages, we can use the SoapClient
class and the SoapVar
class in PHP. The following is an example that shows how to use these classes to generate SOAP messages:
// 创建SoapClient对象 $client = new SoapClient("http://example.com/soap-service?wsdl"); // 创建参数 $params = array( 'param1' => new SoapVar('value1', XSD_STRING), 'param2' => new SoapVar('value2', XSD_STRING) ); // 生成SOAP消息 $soapMessage = $client->__soapCall('SomeSoapMethod', array($params), array('soapaction' => 'SomeSoapMethod'));
In the above code, we first create a SoapClient
object, also using a SOAP description Language (WSDL) URL. We then created an array containing the parameters using the SoapVar
class. Next, we generate a SOAP message using the __soapCall
method and save it to the $soapMessage
variable.
Summary
By using the built-in SOAP extension in PHP, we can easily parse and generate SOAP messages. We can use the SoapClient
class to parse and generate SOAP messages, and the SimpleXMLElement
class to parse the returned SOAP message. We can also use the SoapVar
class to generate SOAP messages containing parameters.
The above is an example of parsing and generating SOAP messages in PHP. Hope it helps you!
The above is the detailed content of How to parse and generate SOAP messages in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



With the popularity of Internet applications, website response speed has become more and more of a focus for users. In order to quickly respond to user requests, websites often use caching technology to cache data, thereby reducing the number of database queries. However, cache expiration time has an important impact on response speed. This article will discuss methods of controlling cache expiration time to help PHP developers better apply caching technology. 1. What is cache expiration time? Cache expiration time refers to the time when the data in the cache is considered expired. It determines when the data in the cache is needed
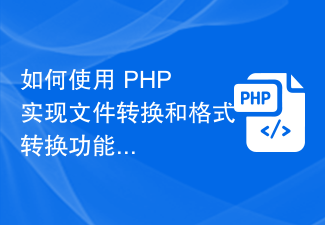
How to use PHP to implement file conversion and format conversion functions 1. Introduction In the process of developing web applications, we often need to implement file conversion and format conversion functions. Whether you are converting image files to other formats or converting text files from one encoding to another, these operations are common needs. This article will describe how to implement these functions using PHP, with code examples. 2. File conversion 2.1 Convert image files to other formats In PHP, we can use
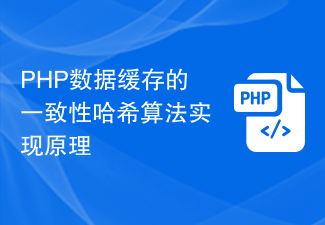
Implementation Principle of Consistent Hash Algorithm for PHP Data Cache Consistent Hashing algorithm (ConsistentHashing) is an algorithm commonly used for data caching in distributed systems, which can minimize the number of data migrations when the system expands and shrinks. In PHP, implementing consistent hashing algorithms can improve the efficiency and reliability of data caching. This article will introduce the principles of consistent hashing algorithms and provide code examples. The basic principle of consistent hashing algorithm. Traditional hashing algorithm disperses data to different nodes, but when the node
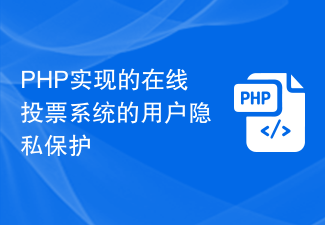
User privacy protection of online voting system implemented in PHP With the development and popularization of the Internet, more and more voting activities have begun to be moved to online platforms. The convenience of online voting systems brings many benefits to users, but it also raises concerns about user privacy leaks. Privacy protection has become an important aspect in the design of online voting systems. This article will introduce how to use PHP to write an online voting system, and focus on the issue of user privacy protection. When designing and developing an online voting system, the following principles need to be followed to ensure
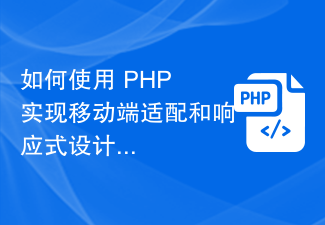
How to use PHP to implement mobile adaptation and responsive design Mobile adaptation and responsive design are important practices in modern website development. They can ensure good display effects of the website on different devices. In this article, we will introduce how to use PHP to implement mobile adaptation and responsive design, with code examples. 1. Understand the concepts of mobile adaptation and responsive design Mobile adaptation refers to providing different styles and layouts for different devices based on the different characteristics and sizes of the device. Responsive design refers to the use of
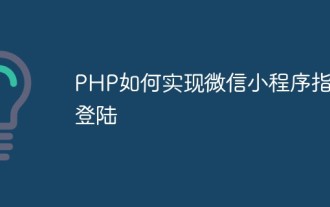
With the continuous development of WeChat mini programs, more and more users are beginning to choose WeChat mini programs to log in. In order to improve users’ login experience, WeChat mini programs began to support fingerprint login. In this article, we will introduce how to use PHP to implement fingerprint login for WeChat mini programs. 1. Understand the fingerprint login of WeChat mini programs On the basis of WeChat mini programs, developers can use WeChat's fingerprint recognition function to allow users to log in to WeChat mini programs through fingerprints, thereby improving the security and convenience of the login experience. 2. Preparation work is implemented using PHP
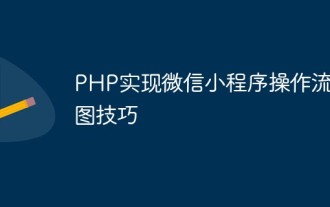
With the rapid development of the mobile Internet, WeChat mini programs are becoming more and more popular among users, and PHP, as a powerful programming language, also plays an important role in the development process of mini programs. This article will introduce the techniques of implementing WeChat applet operation flow chart in PHP. Obtain access_token During the development process of using WeChat applet, you first need to obtain access_token, which is an important credential for realizing the operation of WeChat applet. The code to obtain access_token in PHP is as follows: f
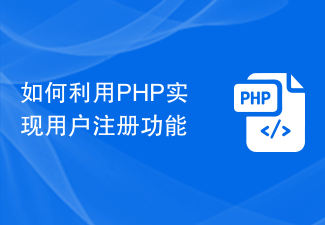
How to use PHP to implement user registration function In modern network applications, user registration function is a very common requirement. Through the registration function, users can create their own accounts and use corresponding functions. This article will implement the user registration function through the PHP programming language and provide detailed code examples. First, we need to create an HTML form to receive the user's registration information. In the form, we need to include some input fields, such as username, password, email, etc. Form fields can be customized according to actual needs.
