OAuth in PHP: Integrating third-party login functionality
OAuth in PHP: Integrating third-party login functions
With the rapid development of social media, more and more websites and applications provide third-party login functions, such as using the user's Facebook, Log in with Google or WeChat account. This method is convenient for users and also improves the conversion rate of user registration and login. In PHP, we can implement this function through the OAuth protocol. In this article, we will explore how to leverage OAuth in PHP to integrate third-party login functionality.
OAuth is an authorization protocol that allows users to authorize third-party applications to access their data without providing their password. In third-party login, OAuth provides a secure way, allowing us to use the API of the third-party platform to obtain the user's basic information and authorization.
First, we need to register a developer account to obtain OAuth authorization credentials. This process usually involves creating an application and obtaining credentials such as client ID and client secret. Taking Facebook as an example, we need to go to the Facebook Developer Platform to create an application.
Once we obtain the credentials, we can write PHP code to implement the third-party login function. We will use a popular PHP library such as League/OAuth2-Client to simplify the entire process.
First, we need to install this library, which can be installed through Composer. Run the following command in the terminal:
composer require league/oauth2-client
Next, we can write a file called oauth_callback.php that will handle the authorization callback logic. Here is a basic example:
<?php require 'vendor/autoload.php'; $provider = new LeagueOAuth2ClientProviderGenericProvider([ 'clientId' => 'YOUR_CLIENT_ID', 'clientSecret' => 'YOUR_CLIENT_SECRET', 'redirectUri' => 'http://your-domain.com/oauth_callback.php', 'urlAuthorize' => 'https://oauth.provider.com/authorize', 'urlAccessToken' => 'https://oauth.provider.com/access_token', 'urlResourceOwnerDetails' => 'https://oauth.provider.com/resource_owner' ]); if (!isset($_GET['code'])) { $authorizationUrl = $provider->getAuthorizationUrl(); $_SESSION['oauth2state'] = $provider->getState(); header('Location: ' . $authorizationUrl); exit; } elseif (empty($_GET['state']) || ($_GET['state'] !== $_SESSION['oauth2state'])) { unset($_SESSION['oauth2state']); exit('Invalid state'); } else { $accessToken = $provider->getAccessToken('authorization_code', [ 'code' => $_GET['code'] ]); $resourceOwner = $provider->getResourceOwner($accessToken); $user = $resourceOwner->toArray(); // 获取第三方登录用户的基本信息 $email = $user['email']; $name = $user['name']; // 进行自己的业务逻辑 }
In this example, we use GenericProvider as the OAuth provider. We need to replace YOUR_CLIENT_ID and YOUR_CLIENT_SECRET with the credentials we obtained on the developer platform. redirectUri is the callback URL after user authorization, which needs to be consistent with the callback URL we set in the application.
In the front-end page, we can add a button or link pointing to the following address:
<a href="oauth_callback.php">使用第三方登录</a>
When users click this link, they will be redirected to the authentication page of the third-party platform, Ask them to authorize our application to access their account information. Once they authorize successfully, they will be redirected back to the oauth_callback.php page with a code parameter.
By calling the getAccessToken method, we can obtain the access token. Then, we can use the getResourceOwner method to obtain the user's basic information, such as email and name.
Finally, we can process this information according to our own business logic, such as creating a user account or logging in to an existing account.
To sum up, by using the OAuth protocol in PHP, we can easily integrate third-party login functionality. I hope this article has been helpful for you to add more login options to your application.
The above is the detailed content of OAuth in PHP: Integrating third-party login functionality. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

PHP and OAuth: Implementing Microsoft login integration With the development of the Internet, more and more websites and applications need to support users to log in using third-party accounts to provide a convenient registration and login experience. Microsoft account is one of the widely used accounts around the world, and many users want to use Microsoft account to log in to websites and applications. In order to achieve Microsoft login integration, we can use the OAuth (Open Authorization) protocol to achieve it. OAuth is an open-standard authorization protocol that allows users to authorize third-party applications to act on their behalf
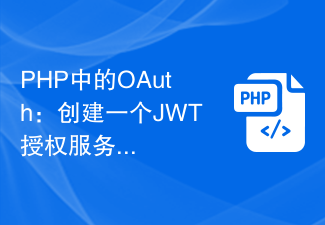
OAuth in PHP: Creating a JWT authorization server With the rise of mobile applications and the trend of separation of front-end and back-end, OAuth has become an indispensable part of modern web applications. OAuth is an authorization protocol that protects users' resources from unauthorized access by providing standardized processes and mechanisms. In this article, we will learn how to create a JWT (JSONWebTokens) based OAuth authorization server using PHP. JWT is a type of
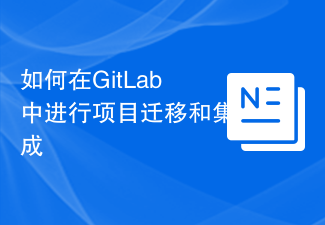
How to migrate and integrate projects in GitLab Introduction: In the software development process, project migration and integration is an important task. As a popular code hosting platform, GitLab provides a series of convenient tools and functions to support project migration and integration. This article will introduce the specific steps for project migration and integration in GitLab, and provide some code examples to help readers better understand. 1. Project migration Project migration is to migrate the existing code base from a source code management system to GitLab

How to do GoogleDrive integration using PHP and OAuth GoogleDrive is a popular cloud storage service that allows users to store files in the cloud and share them with other users. Through GoogleDriveAPI, we can use PHP to write code to integrate with GoogleDrive to implement file uploading, downloading, deletion and other operations. To use GoogleDriveAPI we need to authenticate via OAuth and
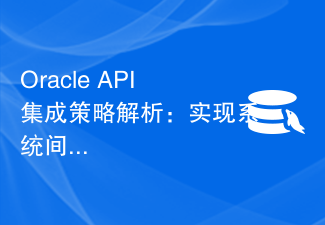
OracleAPI integration strategy analysis: To achieve seamless communication between systems, specific code examples are required. In today's digital era, internal enterprise systems need to communicate with each other and share data, and OracleAPI is one of the important tools to help achieve seamless communication between systems. This article will start with the basic concepts and principles of OracleAPI, explore API integration strategies, and finally give specific code examples to help readers better understand and apply OracleAPI. 1. Basic Oracle API
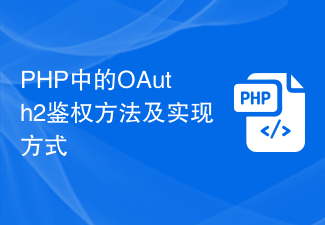
OAuth2 authentication method and implementation in PHP With the development of the Internet, more and more applications need to interact with third-party platforms. In order to protect user privacy and security, many third-party platforms use the OAuth2 protocol to implement user authentication. In this article, we will introduce the OAuth2 authentication method and implementation in PHP, and attach corresponding code examples. OAuth2 is an authorization framework that allows users to authorize third-party applications to access their resources on another service provider without mentioning
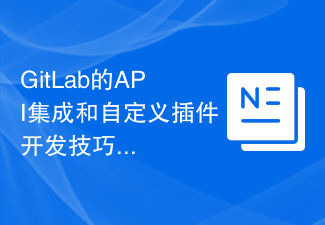
GitLab's API integration and custom plug-in development skills Introduction: GitLab is an open source code hosting platform that provides a rich API interface for developers to use to facilitate integration and custom plug-in development. This article will introduce how to integrate GitLab's API and some tips on custom plug-in development, and provide specific code examples. 1. Obtain API access token for GitLab's API integration. Before API integration, you first need to obtain GitLab's API access token. beat
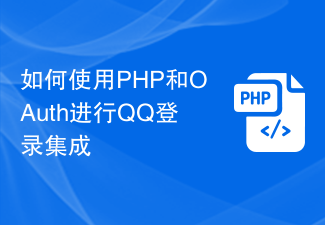
Introduction to how to use PHP and OAuth for QQ login integration: With the development of social media, more and more websites and applications are beginning to provide third-party login functions to facilitate users to quickly register and log in. As one of China's largest social media platforms, QQ has also become a third-party login service provided by many websites and applications. This article will introduce the steps on how to use PHP and OAuth for QQ login integration, with code examples. Step 1: Register as a QQ open platform developer. Before starting to integrate QQ login, I
