How to build a real-time chat application using Redis and Node.js
How to use Redis and Node.js to build a real-time chat application
Introduction:
With the continuous development of Internet technology, real-time communication has become a part of modern people's daily life. Whether it is social networks, online games, or online customer service, real-time chat applications have been widely used. This article will introduce how to use Redis and Node.js to build a simple real-time chat application, and detail the implementation process of the real-time chat application through code examples.
1. Overview
The key to real-time chat applications is real-time messaging and instant updates. To achieve this goal, we will use the following technologies and tools:
- Redis: a high-performance in-memory database for storing chat messages.
- Node.js: An event-driven asynchronous I/O framework for building back-end servers for chat applications.
- Socket.IO: A JavaScript library for real-time application development for real-time communication between front-end and back-end.
2. Environment preparation
Before we start, we need to install and configure the following tools:
- Node.js: Please download and install the latest version according to the operating system Node.js.
- Redis: Please download and install the latest version of Redis according to your operating system.
-
Socket.IO: Execute the following command through the command line to install Socket.IO:
npm install socket.io
Copy after login
3. Implementation process
-
Create an empty Node.js project and install the dependencies:
npm init npm install express redis socket.io
Copy after login Create a file named
app.js
in the project root directory, And add the following code:const express = require('express'); const app = express(); const server = require('http').Server(app); const io = require('socket.io')(server); const redis = require('redis'); const redisClient = redis.createClient(); server.listen(3000, () => { console.log('Server is running on port 3000'); }); app.get('/', (req, res) => { res.sendFile(__dirname + '/index.html'); }); io.on('connection', (socket) => { socket.on('join', (room) => { socket.join(room); }); socket.on('sendMessage', (data) => { redisClient.lpush(data.room, data.message); io.in(data.room).emit('receiveMessage', data.message); }); });
Copy after loginCreate a file named
index.html
in the project root directory and add the following code:<!DOCTYPE html> <html> <head> <title>Real-time Chat</title> <script src="/socket.io/socket.io.js"></script> <script> const socket = io(); socket.emit('join', 'room1'); socket.on('receiveMessage', (message) => { const li = document.createElement('li'); li.textContent = message; document.getElementById('messages').appendChild(li); }); function sendMessage() { const input = document.getElementById('message'); const message = input.value; input.value = ''; socket.emit('sendMessage', { room: 'room1', message: message }); } </script> </head> <body> <ul id="messages"></ul> <input id="message" type="text" /> <button onclick="sendMessage()">Send</button> </body> </html>
Copy after loginStart the Redis service:
redis-server
Copy after loginStart the Node.js server:
node app.js
Copy after login- Access in the browser
http: //localhost:3000
, open multiple tabs or browser windows, enter a message and click the send button to achieve real-time chat.
4. Implementation Principle
- The user enters a message in the browser and clicks the send button. The front-end JavaScript code sends
sendMessage to the back-end through Socket.IO.
event, and carries message and room information. - After the back-end Node.js server receives the
sendMessage
event, it stores the message in Redis and sendsreceiveMessage# to all clients in the same room through Socket.IO. ## event and carries the same message content.
After the client receives the - receiveMessage
event, it displays the message in the chat window.
Through the above steps, we successfully built a simple real-time chat application using Redis and Node.js. This application can continue to be expanded, such as adding user authentication, message recording and other functions. I hope this article can help everyone understand and learn the development process of real-time chat applications, and apply it in actual projects.
The above is the detailed content of How to build a real-time chat application using Redis and Node.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


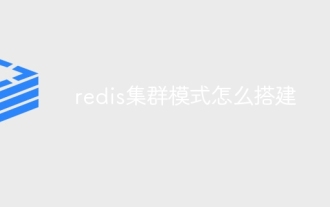
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make

Redis uses hash tables to store data and supports data structures such as strings, lists, hash tables, collections and ordered collections. Redis persists data through snapshots (RDB) and append write-only (AOF) mechanisms. Redis uses master-slave replication to improve data availability. Redis uses a single-threaded event loop to handle connections and commands to ensure data atomicity and consistency. Redis sets the expiration time for the key and uses the lazy delete mechanism to delete the expiration key.
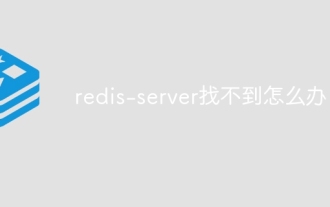
Steps to solve the problem that redis-server cannot find: Check the installation to make sure Redis is installed correctly; set the environment variables REDIS_HOST and REDIS_PORT; start the Redis server redis-server; check whether the server is running redis-cli ping.
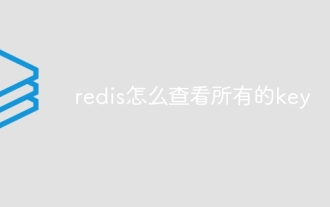
To view all keys in Redis, there are three ways: use the KEYS command to return all keys that match the specified pattern; use the SCAN command to iterate over the keys and return a set of keys; use the INFO command to get the total number of keys.
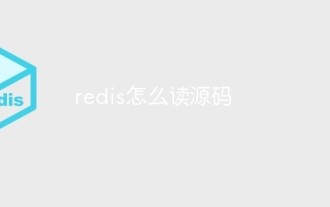
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.
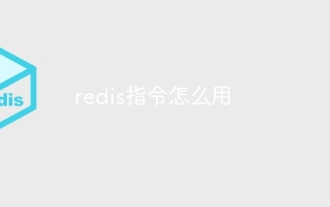
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
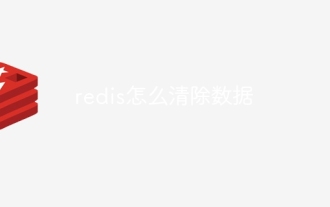
The following two methods can be used to clear data in Redis: FLUSHALL command: Delete all keys and values in the database. CONFIG RESETSTAT command: Reset all states of the database (including keys, values, and other statistics).
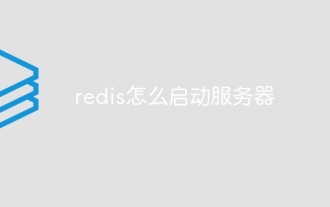
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
