Build efficient Ruby applications with Redis
Build efficient Ruby applications using Redis
Redis is a fast, open source in-memory data structure storage system that can be used as a database, cache, and messaging middleware. It supports a variety of data structures (such as strings, lists, hashes, sets, etc.) and provides rich functions, including data persistence, replication, and master-slave mode. Using Redis in Ruby applications can improve performance and scalability.
This article will introduce how to use Redis to build efficient Ruby applications and provide some code examples.
- Installing Redis
First, you need to install the Redis server locally. You can download the latest stable version from the Redis official website (https://redis.io/download) and install it according to the official documentation.
- Install Redis gem
To use Redis in a Ruby application, you need to install the corresponding Redis gem. You can install it by running the following command:
gem install redis
- Connect to the Redis server
To connect to the Redis server, you need to use the Redis class provided by the Redis gem. The following is an example of connecting to a local Redis server:
require 'redis' redis = Redis.new(host: 'localhost', port: 6379)
- Storing and retrieving data
Redis supports a variety of data structures, and you can choose the appropriate one based on the needs of your application data structure. Here are some common data manipulation examples:
- Store and get strings:
redis.set('key', 'value') value = redis.get('key')
- Store and get hashes:
redis.hset('hash', 'field', 'value') value = redis.hget('hash', 'field')
- Storing and retrieving lists:
redis.lpush('list', 'value1', 'value2') values = redis.lrange('list', 0, -1)
- Storing and retrieving sets:
redis.sadd('set', 'value1', 'value2') values = redis.smembers('set')
- Storing and retrieving ordered sets:
redis.zadd('sorted_set', 1, 'value1') values = redis.zrange('sorted_set', 0, -1)
- Using Redis Cache
Redis can be used as a cache to improve application performance. Frequently executed calculation results or database query results can be stored in Redis so that they can be quickly obtained the next time they are used.
The following is an example of using Redis as a cache:
def get_data_from_cache(key) value = redis.get(key) if value.nil? value = expensive_operation() redis.set(key, value) end return value end # 使用缓存获取数据 data = get_data_from_cache('data_key')
In the above example, if cached data exists in Redis, it is obtained directly from Redis; if cached data does not exist in Redis, Then perform expensive operations (such as reading the database) and store the results in Redis for next time use. This avoids frequent expensive operations and improves application performance.
- Use Redis to publish and subscribe to messages
Redis can also be used as message middleware to support the function of publishing and subscribing messages. You can use the methods provided by the Redis gem to implement publishing and subscribing messages.
The following is an example of using Redis to publish and subscribe to messages:
require 'redis' redis = Redis.new # 发布消息 redis.publish('channel', 'message') # 订阅消息 redis.subscribe('channel') do |on| on.message do |channel, message| puts "Received message: #{message} from channel #{channel}" end end
In the above example, data is sent to the specified channel by publishing the message, and then using the subscribe message to receive and process the data from Messages from this channel.
Summary
Redis is a feature-rich in-memory data storage system that can be used to build efficient Ruby applications. By using Redis, fast data storage and retrieval can be achieved, improving application performance and scalability. This article describes how to install and connect to the Redis server, and provides some common Redis data operation examples. In addition, it also introduces how to use Redis as cache and message middleware. I hope this article was helpful in building efficient Ruby applications using Redis.
References:
- Redis official website: https://redis.io/
- Redis gem documentation: https://github.com/redis/ redis-rb
The above is the detailed content of Build efficient Ruby applications with Redis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


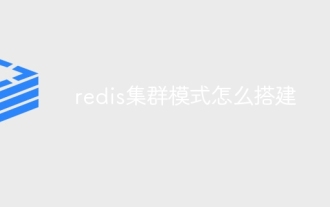
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
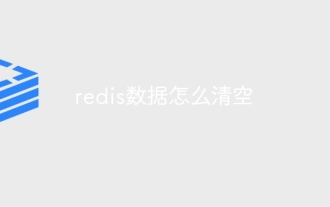
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
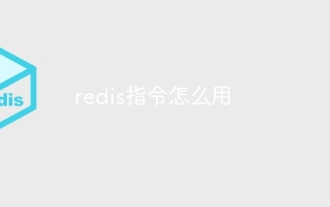
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
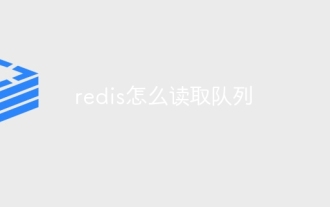
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
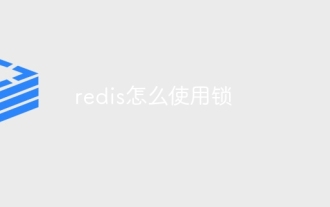
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.

Redis uses hash tables to store data and supports data structures such as strings, lists, hash tables, collections and ordered collections. Redis persists data through snapshots (RDB) and append write-only (AOF) mechanisms. Redis uses master-slave replication to improve data availability. Redis uses a single-threaded event loop to handle connections and commands to ensure data atomicity and consistency. Redis sets the expiration time for the key and uses the lazy delete mechanism to delete the expiration key.
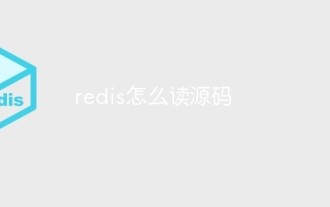
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.

Redis, as a message middleware, supports production-consumption models, can persist messages and ensure reliable delivery. Using Redis as the message middleware enables low latency, reliable and scalable messaging.
