Application of Redis in JavaScript development: How to implement data caching
Application of Redis in JavaScript development: How to implement data caching
Introduction:
In JavaScript development, data caching is a very important concept. It improves application performance and responsiveness and reduces the number of requests to the server. Redis (Remote Dictionary Server) is an open source in-memory database that can be used for data caching in high-performance applications. This article will introduce the application of Redis in JavaScript development and show how to implement data caching through code examples.
Content text:
Redis is a memory-based data storage system that can load data into memory to improve access speed. It provides rich data structures and commands to implement data caching in JavaScript development.
First, we need to introduce the Redis client library into the JavaScript project. A commonly used Redis client library is ioredis, which provides a rich API to interact with Redis. The ioredis library can be installed through npm:
npm install ioredis
After the installation is completed, introduce the ioredis library into the JavaScript file:
const Redis = require('ioredis'); const redis = new Redis();
Next, we can use Redis for data caching.
- Single data cache
We can store a data item in Redis and set the expiration time to achieve data caching. The following is a sample code:
async function getDataFromCache(key) { const cachedData = await redis.get(key); if (cachedData) { console.log('从缓存中获取数据'); return JSON.parse(cachedData); } console.log('从数据库获取数据'); const dataFromDB = await fetchDataFromDB(key); redis.set(key, JSON.stringify(dataFromDB), 'ex', 60); return dataFromDB; } async function fetchDataFromDB(key) { // 从数据库中获取数据的逻辑 } // 使用方式: const data = await getDataFromCache('exampleKey');
In the above code, we first check whether the specified key exists in Redis. If it exists, we will directly obtain the data from the cache and return it; if it does not exist, we will retrieve it from the database. Get the data and store it in Redis, and set the expiration time. In this way, the next time you request the same key, the data will be obtained directly from the Redis cache without querying the database again.
- Multiple data cache
In addition to caching single data, we can also store multiple data items in Redis. The following is a sample code:
async function getDataListFromCache(keys) { const cachedData = await redis.mget(keys); const nonCachedDataKeys = []; const dataMap = {}; for (let i = 0; i < keys.length; i++) { const key = keys[i]; const cachedDatum = cachedData[i]; if (cachedDatum) { dataMap[key] = JSON.parse(cachedDatum); } else { nonCachedDataKeys.push(key); } } if (nonCachedDataKeys.length > 0) { console.log('从数据库获取数据'); const dataFromDB = await fetchDataListFromDB(nonCachedDataKeys); for (const data of dataFromDB) { const key = data.key; redis.set(key, JSON.stringify(data), 'ex', 60); dataMap[key] = data; } } return keys.map(key => dataMap[key]); } async function fetchDataListFromDB(keys) { // 从数据库中批量获取数据的逻辑 } // 使用方式: const dataList = await getDataListFromCache(['key1', 'key2', 'key3']);
In the above code, we first obtain the data corresponding to multiple keys at one time through the mget
command. Then, iterate through the obtained data, parse the cache hit (existing) data into objects, and record the cache miss (non-existent) keys. Then, cache miss data is obtained in batches from the database and stored in Redis. Finally, all requested data is returned.
Conclusion:
This article introduces the application of Redis in JavaScript development and shows how to implement data caching through code examples. By using Redis for data caching, the performance and responsiveness of your application can be significantly improved. At the same time, Redis, as a powerful in-memory database, can also implement more advanced functions, such as publish/subscribe, rankings, etc. Therefore, in JavaScript development, we should be good at using Redis to optimize and improve application performance and user experience.
References:
- ioredis official documentation: https://github.com/luin/ioredis
The above is the detailed content of Application of Redis in JavaScript development: How to implement data caching. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


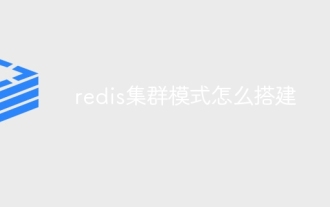
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
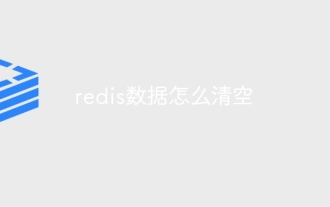
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
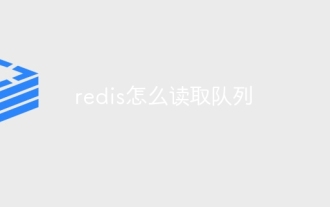
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
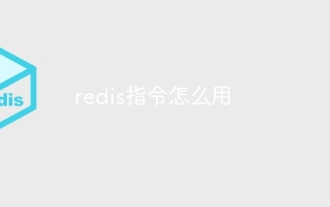
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
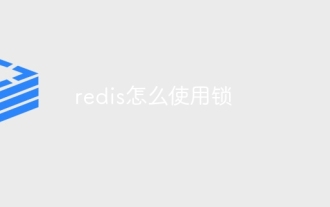
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
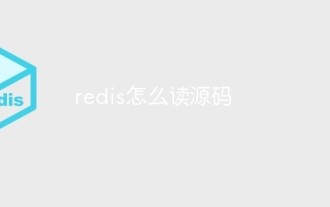
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.

Redis data loss causes include memory failures, power outages, human errors, and hardware failures. The solutions are: 1. Store data to disk with RDB or AOF persistence; 2. Copy to multiple servers for high availability; 3. HA with Redis Sentinel or Redis Cluster; 4. Create snapshots to back up data; 5. Implement best practices such as persistence, replication, snapshots, monitoring, and security measures.
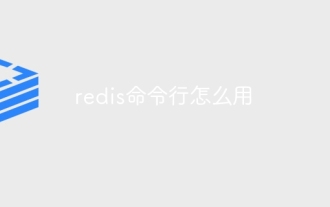
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
