How to implement custom middleware in CodeIgniter
How to implement custom middleware in CodeIgniter
Introduction:
In modern web development, middleware plays a vital role in applications. They can be used to perform some shared processing logic before or after the request reaches the controller. CodeIgniter, as a popular PHP framework, also supports the use of middleware. This article will introduce how to implement custom middleware in CodeIgniter and provide a simple code example.
Middleware Overview:
Middleware is a mechanism that performs some action before or after the request reaches the controller. They can be used to handle common business logic such as authentication, logging, access control, etc. In CodeIgniter, middleware can be thought of as an interceptor, capable of performing some additional logic before or after the request reaches the controller.
The steps to implement custom middleware are as follows:
Step 1: Create a custom middleware class
Create a middleware class, named MyMiddleware, and place it in application/middlewares Save in directory. This class needs to implement the CodeIgniterMiddlewareMiddlewareInterface interface and implement the handle() method. The handle() method is the core method of middleware and is used to handle specific logic.
<?php namespace AppMiddlewares; use CodeIgniterMiddlewareMiddlewareInterface; use CodeIgniterHTTPRequestInterface; use CodeIgniterHTTPResponseInterface; class MyMiddleware implements MiddlewareInterface { public function handle(RequestInterface $request, ResponseInterface $response, $arguments = null) { // 在请求到达控制器之前执行的代码 // 可以在这里实现身份验证、日志记录、访问控制等逻辑 // 继续处理请求 $response = $this->next($request, $response); // 在请求到达控制器之后执行的代码 // 可以在这里实现日志记录、响应处理等逻辑 return $response; } }
Step 2: Register middleware
Open the app/Config/Autoload.php file and add the following configuration in the $psr4 array to automatically load the middleware class:
$psr4 = [ 'App' => APPPATH, // 默认 'Config' => APPPATH . 'Config', 'Modules' => ROOTPATH . 'modules', // 可选 'AppMiddlewares' => APPPATH . 'middlewares' // 添加这一行 ];
Then open the app/Config/Events.php file and add the class name of the middleware in the 'middleware' key of the $filters array, as shown below:
$filters = [ 'csrf' => AppFiltersCSRF::class, 'toolbar' => AppFiltersDebugToolbar::class, 'honeypot' => AppFiltersHoneypot::class, 'middleware' => AppMiddlewaresMyMiddleware::class // 添加这一行 ];
Step 3: Use middleware
To To use middleware in a controller, just add the following code in the __construct() method of the controller:
public function __construct() { $this->middleware('MyMiddleware'); }
Middleware will be executed in the order registered in the $filters array. You can also use $this->middleware([]) in the controller's __construct() method to add multiple middlewares and define their execution order.
Summary:
This article introduces how to implement custom middleware in CodeIgniter. By creating a custom middleware class and registering it with the application, we can perform some common processing logic before or after the request reaches the controller. I hope this article can help you better understand and use middleware in CodeIgniter.
Reference:
- CodeIgniter official documentation: https://codeigniter.com/user_guide/incoming/middleware.html
The above is the detailed content of How to implement custom middleware in CodeIgniter. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


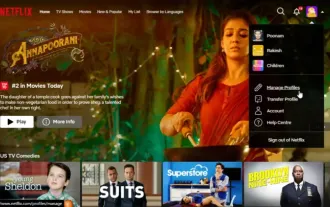
An avatar on Netflix is a visual representation of your streaming identity. Users can go beyond the default avatar to express their personality. Continue reading this article to learn how to set a custom profile picture in the Netflix app. How to quickly set a custom avatar in Netflix In Netflix, there is no built-in feature to set a profile picture. However, you can do this by installing the Netflix extension on your browser. First, install a custom profile picture for the Netflix extension on your browser. You can buy it in the Chrome store. After installing the extension, open Netflix on your browser and log into your account. Navigate to your profile in the upper right corner and click
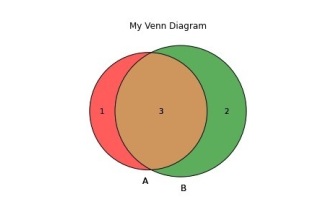
A Venn diagram is a diagram used to represent relationships between sets. To create a Venn diagram we will use matplotlib. Matplotlib is a commonly used data visualization library in Python for creating interactive charts and graphs. It is also used to create interactive images and charts. Matplotlib provides many functions to customize charts and graphs. In this tutorial, we will illustrate three examples to customize Venn diagrams. The Chinese translation of Example is: Example This is a simple example of creating the intersection of two Venn diagrams; first, we imported the necessary libraries and imported venns. Then we create the dataset as a Python set, after that we use the "venn2()" function to create
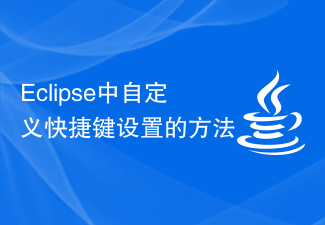
How to customize shortcut key settings in Eclipse? As a developer, mastering shortcut keys is one of the keys to improving efficiency when coding in Eclipse. As a powerful integrated development environment, Eclipse not only provides many default shortcut keys, but also allows users to customize them according to their own preferences. This article will introduce how to customize shortcut key settings in Eclipse and give specific code examples. Open Eclipse First, open Eclipse and enter
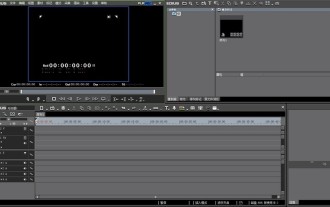
1. The picture below is the default screen layout of edius. The default EDIUS window layout is a horizontal layout. Therefore, in a single-monitor environment, many windows overlap and the preview window is in single-window mode. 2. You can enable [Dual Window Mode] through the [View] menu bar to make the preview window display the playback window and recording window at the same time. 3. You can restore the default screen layout through [View menu bar>Window Layout>General]. In addition, you can also customize the layout that suits you and save it as a commonly used screen layout: drag the window to a layout that suits you, then click [View > Window Layout > Save Current Layout > New], and in the pop-up [Save Current Layout] Layout] enter the layout name in the small window and click OK
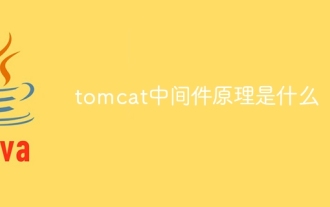
The principle of tomcat middleware is implemented based on Java Servlet and Java EE specifications. As a Servlet container, Tomcat is responsible for processing HTTP requests and responses and providing the running environment for Web applications. The principles of Tomcat middleware mainly involve: 1. Container model; 2. Component architecture; 3. Servlet processing mechanism; 4. Event listening and filters; 5. Configuration management; 6. Security; 7. Clustering and load balancing; 8. Connector technology; 9. Embedded mode, etc.
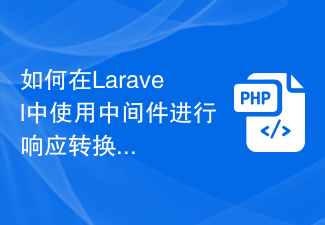
How to use middleware for response conversion in Laravel Middleware is one of the very powerful and practical features in the Laravel framework. It allows us to process requests and responses before the request enters the controller or before the response is sent to the client. In this article, I will demonstrate how to use middleware for response transformation in Laravel. Before starting, make sure you have Laravel installed and a new project created. Now we will follow these steps: Create a new middleware Open
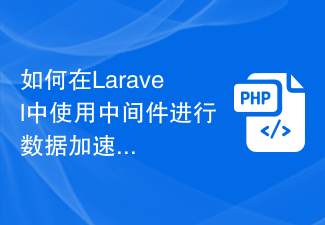
How to use middleware for data acceleration in Laravel Introduction: When developing web applications using the Laravel framework, data acceleration is the key to improving application performance. Middleware is an important feature provided by Laravel that handles requests before they reach the controller or before the response is returned. This article will focus on how to use middleware to achieve data acceleration in Laravel and provide specific code examples. 1. What is middleware? Middleware is a mechanism in the Laravel framework. It is used
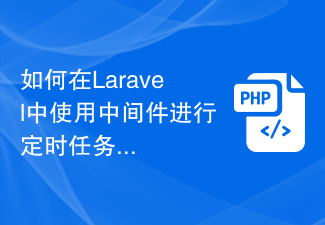
How to use middleware for scheduled task scheduling in Laravel Introduction: Laravel is a popular PHP open source framework that provides convenient and powerful tools to develop web applications. One of the important features is scheduled tasks, which allows developers to run specific tasks at specified intervals. In this article, we will introduce how to use middleware to implement Laravel's scheduled task scheduling, and provide specific code examples. Environment Preparation Before starting, we need to make sure
