


Quick Start: Use Go language functions to implement simple data aggregation functions
Quick Start: Use Go language functions to implement simple data aggregation functions
In software development, we often encounter situations where we need to aggregate a set of data. Aggregation operations can count, summarize, calculate, etc., to analyze and display data. In the Go language, we can use functions to implement simple data aggregation functions.
First, we need to define a data type to represent the data we want to aggregate. Suppose we have a student's grade table, and each student has two fields: name and grade. Then we can create the following structure type:
type Student struct { Name string Score int }
Next, we can create a slice containing student data, And initialize some sample data:
students := []Student { {Name: "张三", Score: 90}, {Name: "李四", Score: 80}, {Name: "王五", Score: 85}, {Name: "赵六", Score: 92}, {Name: "钱七", Score: 88}, }
Now, we can start writing aggregate functions. We can create a function that receives a slice of student data as a parameter and returns the aggregated result. We can use a loop to iterate through each student in the slice and then perform statistical or computational operations as needed.
The following is a simple sample code that implements the calculation of student average grades:
func GetAverageScore(students []Student) float64 { total := 0 count := len(students) for _, student := range students { total += student.Score } if count > 0 { return float64(total) / float64(count) } else { return 0 } }
In the above code, we first initialize an accumulator total
and A counter count
is used to count the total score and the number of students respectively. We then use a loop to loop through the student data slices and add each student's grade into total
. Finally, we return the average grade based on the value of the counter, or 0 if the number of students is zero.
We can call the aggregate function in the main function and print the result:
func main() { averageScore := GetAverageScore(students) fmt.Println("学生平均成绩:", averageScore) }
The above code will output:
学生平均成绩: 87
In addition to calculating the average grade, we can also implement it as needed Other aggregation functions, such as statistics of the highest score, lowest score, total score, etc. The implementation is similar, but different code logic needs to be written according to specific needs.
To sum up, using Go language functions to implement simple data aggregation functions is a simple and efficient method. We can quickly implement statistics, summary, calculation and other operations on a set of data by defining appropriate data types and writing appropriate aggregation functions. Such code has a clear structure, is easy to understand and maintain, and can improve development efficiency and code quality.
I hope this article will help you understand and use Go language functions to implement data aggregation functions!
The above is the detailed content of Quick Start: Use Go language functions to implement simple data aggregation functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




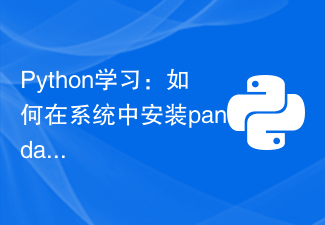
Quick Start: How to install the pandas library in Python requires specific code examples 1. Overview Python is a widely used programming language with a powerful development ecosystem that includes many practical libraries. Pandas is one of the most popular data analysis libraries. It provides efficient data structures and data analysis tools, making data processing and analysis easier. This article will introduce how to install the pandas library in Python and provide corresponding code examples. 2. Install Py
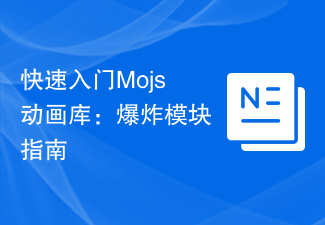
We start this series by learning how to animate HTML elements using mojs. In this second tutorial, we continue using the Shape module to animate built-in SVG shapes. The third tutorial covers more ways to animate SVG shapes using ShapeSwirl and the stagger module. Now we will learn how to animate different SVG shapes in bursts using the Burst module. This tutorial will depend on the concepts we introduced in the previous three tutorials. If you haven't read them yet, I recommend reading them first. Creating a Basic Burst Animation The first thing we need to do before creating any burst animation is to instantiate a Burst object. Afterwards, we can specify different properties
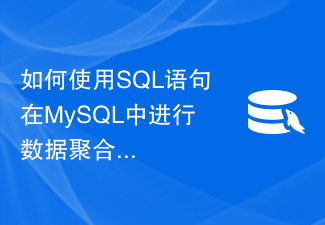
How to use SQL statements for data aggregation and statistics in MySQL? Data aggregation and statistics are very important steps when performing data analysis and statistics. As a powerful relational database management system, MySQL provides a wealth of aggregation and statistical functions, which can easily perform data aggregation and statistical operations. This article will introduce the method of using SQL statements to perform data aggregation and statistics in MySQL, and provide specific code examples. 1. Use the COUNT function for counting. The COUNT function is the most commonly used
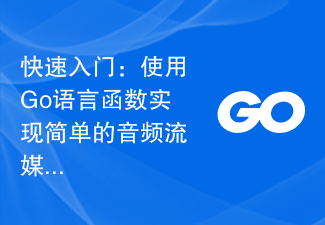
Quick Start: Implementing a Simple Audio Streaming Service Using Go Language Functions Introduction: Audio streaming services are becoming more and more popular in today's digital world, which allow us to play audio files directly over the network without performing a complete download. This article will introduce how to use Go language functions to quickly implement a simple audio streaming service so that you can better understand and use this function. Step 1: Preparation First, you need to install the Go language development environment. You can download it from the official website (https://golan
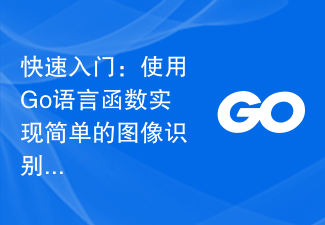
Quick Start: Use Go language functions to implement simple image recognition functions In today's technological development, image recognition technology has become a hot topic. As a fast and efficient programming language, Go language has the ability to implement image recognition functions. This article will provide readers with a quick start guide by using Go language functions to implement simple image recognition functions. First, we need to install the Go language development environment. You can download the appropriate version on the Go language official website (https://golang.org/)
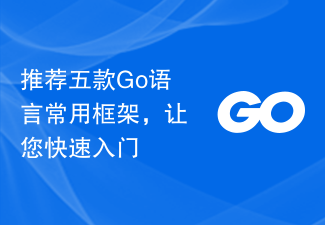
Title: Get Started Quickly: Recommended Five Common Go Language Frameworks In recent years, with the popularity of the Go language, more and more developers have chosen to use Go for project development. The Go language has received widespread attention for its efficiency, simplicity and superior performance. In Go language development, choosing a suitable framework can improve development efficiency and code quality. This article will introduce five commonly used frameworks in the Go language, and attach code examples to help readers get started quickly. Gin framework Gin is a lightweight web framework that is fast and efficient.
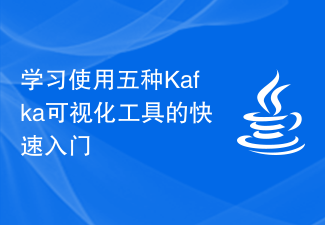
Quick Start: A Guide to Using Five Kafka Visualization Tools 1. Kafka Monitoring Tools: Introduction Apache Kafka is a distributed publish-subscribe messaging system that can handle large amounts of data and provide high throughput and low latency. Due to the complexity of Kafka, visualization tools are needed to help monitor and manage Kafka clusters. 2.Kafka visualization tools: five major choices KafkaManager: KafkaManager is an open source web community
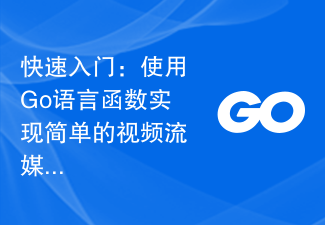
Quick Start: Implementing a Simple Video Streaming Service Using Go Language Functions Introduction: Video streaming services play an important role in modern applications. This article will introduce how to use Go language functions to implement a simple video streaming service. We will use the net/http package of Go language to handle HTTP requests, and combine it with the FFmpeg library to handle the encoding and decoding of video streams. Step 1: Install FFmpeg Before we start writing code, we need to install the FFmpeg library. Can be accessed through FFmpeg official website
