


Building a distributed cache system using Java and Redis: How to improve application scalability
Building a distributed cache system using Java and Redis: How to improve application scalability
Introduction:
In modern distributed applications, caching is a key component to improve performance and scalability one. Redis is a widely used in-memory data storage system that provides fast and efficient data access. This article will introduce how to use Java and Redis to build a distributed cache system, and demonstrate how to improve the scalability of the application through code examples.
1. Overview:
The distributed cache system improves the performance and scalability of the cache by dispersing cache data on multiple nodes. It can provide a fast caching layer on the front end of the application, reducing access to the underlying storage. Here, we will use Redis as our cache server and Java as our application development language.
2. Preparation:
First, we need to install the Redis server and ensure that it can run normally. You can find installation instructions on Redis' official website.
Then, we need to configure the Java project to be able to use Redis. We can use Java's Redis client library to communicate with Redis. Here we will use the Jedis client library.
You can add Jedis to the Maven project in the following ways:
<groupId>redis.clients</groupId> <artifactId>jedis</artifactId> <version>2.10.2</version>
3. Build Distributed cache system:
The following is a simple example showing how to build a distributed cache system using Java and Redis.
First, we need to create a cache manager class. This class will be responsible for interacting with the Redis server and providing methods for operating cached data. The following is a code example for this class:
import redis.clients.jedis.Jedis;
public class CacheManager {
private static CacheManager instance; private Jedis jedis; private CacheManager() { jedis = new Jedis("localhost"); // 连接到Redis服务器 } public static synchronized CacheManager getInstance() { if (instance == null) { instance = new CacheManager(); } return instance; } public String get(String key) { return jedis.get(key); // 从缓存中获取数据 } public void set(String key, String value) { jedis.set(key, value); // 将数据存入缓存 }
}
above In the code, we use the singleton pattern to ensure that there is only one CacheManager instance. This is to ensure that our application only connects to the Redis server once.
Next, we can use CacheManager in the application to read and write cache data. The following is a simple example:
public class MyApp {
public static void main(String[] args) { CacheManager cacheManager = CacheManager.getInstance(); // 写入缓存 cacheManager.set("username", "john"); // 从缓存中读取数据 String username = cacheManager.get("username"); System.out.println(username); // 输出:john }
}
In the above example, we first obtain the instance of CacheManager, and then set the Data is written to cache. Then, we read the data from the cache using the get method and printed it out.
4. Improve the scalability of the application:
In order to improve the scalability of the application, we can use Redis's sharding technology to disperse cache data and store it on multiple Redis servers.
The following is a sample code that shows how to use JedisCluster to implement Redis sharding:
import redis.clients.jedis.JedisCluster;
public class ShardCacheManager {
private static ShardCacheManager instance; private JedisCluster jedisCluster; private ShardCacheManager() { jedisCluster = new JedisCluster(new HostAndPort("localhost", 7000)); // 连接到集群服务器 } public static synchronized ShardCacheManager getInstance() { if (instance == null) { instance = new ShardCacheManager(); } return instance; } public String get(String key) { return jedisCluster.get(key); // 从缓存中获取数据 } public void set(String key, String value) { jedisCluster.set(key, value); // 将数据存入缓存 }
}
In the above code, we use JedisCluster to connect to the Redis cluster server. This class will automatically store data distributed across multiple nodes, improving cache scalability.
Using ShardCacheManager is exactly the same as CacheManager. Just use one of them as a cache manager in our application.
Summary:
This article introduces how to use Java and Redis to build a distributed cache system, and demonstrates how to improve the scalability of the application through code examples. By building a scalable distributed cache system, we can improve the performance and scalability of applications and provide users with a better experience.
Reference materials:
- Redis official website: https://redis.io/
- Jedis GitHub repository: https://github.com/redis/ jedis
The above is the detailed content of Building a distributed cache system using Java and Redis: How to improve application scalability. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


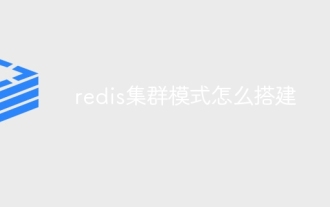
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
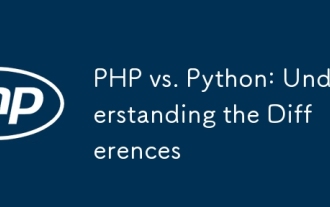
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
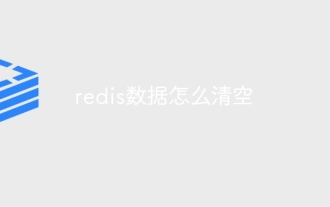
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
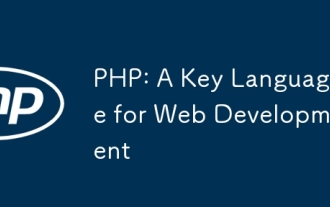
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
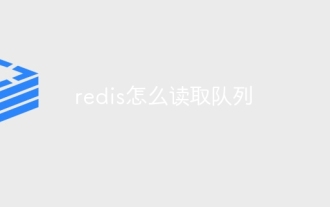
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
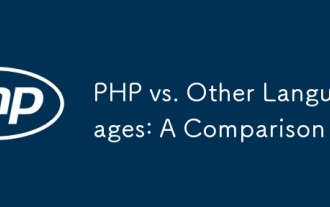
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
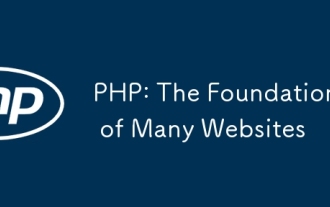
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
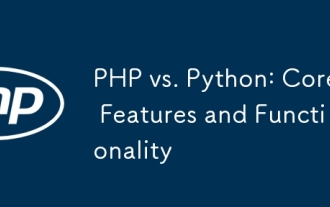
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
