


How to use the ConfigParser module for configuration file operations in Python 2.x
How to use the ConfigParser module for configuration file operations in Python 2.x
1. Introduction
In actual project development, we often encounter situations where we need to read configuration files. Configuration files can be used to store some configuration information, such as database connection information, API keys, etc. The ConfigParser module in Python provides a simple yet powerful solution to easily read and modify configuration file contents. This article will introduce how to use the ConfigParser module for configuration file operations.
2. Introduction to the ConfigParser module
The ConfigParser module is a module in Python used to operate the INI file format. INI files are a common configuration file format, usually composed of sections and key-value pairs. The ConfigParser module provides methods to read, write, and modify configuration file contents.
3. Install the ConfigParser module
The ConfigParser module is part of the Python standard library and is already installed by default in Python 2.x. No additional installation is required.
4. Use the ConfigParser module
First, import the ConfigParser module:
import ConfigParser
The following is a typical INI file format:
[database] host = localhost port = 3306 username = root password = password123 [api] key = myapikey
The following is the use Sample code for the ConfigParser module to read INI files:
config = ConfigParser.ConfigParser() config.read('config.ini') # 获取 'database' 节中的 'host' 键的值 database_host = config.get('database', 'host') print(database_host) # 输出: localhost # 获取 'api' 节中的 'key' 键的值 api_key = config.get('api', 'key') print(api_key) # 输出: myapikey
config.get(section, option)
method is used to obtain the value of the specified key (option) in the specified section (section) .
In the above code example, we first create a ConfigParser object config
, and then use the config.read('config.ini')
method to read the configuration file . Next, you can use the config.get()
method to get the key values in each section.
If the key in the configuration file does not exist, or the section does not exist, the config.get()
method will throw a ConfigParser.Error
exception. We can use the config.has_option()
and config.has_section()
methods to check whether the key or section exists to avoid exceptions.
The following is a sample code for using the ConfigParser module to modify the INI file:
config = ConfigParser.ConfigParser() config.read('config.ini') # 修改 'database' 节中的 'host' 键的值 config.set('database', 'host', 'newhost') # 添加新的节和键值对 config.add_section('new_section') config.set('new_section', 'new_option', 'value') # 保存修改后的配置文件 with open('config.ini', 'w') as f: config.write(f)
config.set(section, option, value)
method is used to modify the configuration file Specify the value of the key, the config.add_section(section)
method is used to add a new section, and the config.write(file)
method is used to write the modified configuration file to disk.
5. Summary
The ConfigParser module provides a convenient way to read, write and modify INI format configuration files. Profile manipulation is easy with simple API calls. In actual project development, the ConfigParser module can be used to effectively manage configuration information and improve the scalability and maintainability of the code.
This article introduces the basic usage and common operations of the ConfigParser module, including reading and modifying configuration files. I hope this article can provide readers with a simple and practical method to handle configuration files, which will be helpful in actual development work.
For more detailed information about the ConfigParser module, please refer to the official Python documentation: https://docs.python.org/2/library/configparser.html
The above is the detailed content of How to use the ConfigParser module for configuration file operations in Python 2.x. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


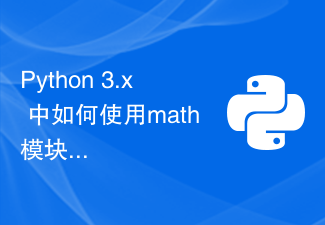
How to use the math module to perform mathematical operations in Python 3.x Introduction: In Python programming, performing mathematical operations is a common requirement. In order to facilitate processing of mathematical operations, Python provides the math library, which contains many functions and constants for mathematical calculations and mathematical functions. This article will introduce how to use the math module to perform common mathematical operations and provide corresponding code examples. 1. Basic mathematical operation addition is performed using the function math.add() in the math module.
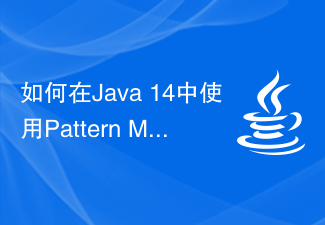
How to use PatternMatching for type pattern matching in Java14 Introduction: Java14 introduces a new feature, PatternMatching, which is a powerful tool that can be used for type pattern matching at compile time. This article will introduce how to use PatternMatching for type pattern matching in Java14 and provide code examples. Understand the concept of PatternMatchingPattern
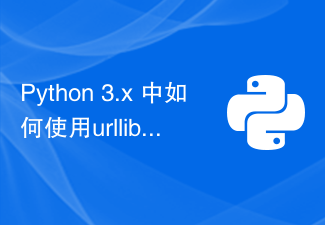
How to use the urllib.parse.unquote() function to decode URLs in Python 3.x. In Python's urllib library, the urllib.parse module provides a series of tool functions for URL encoding and decoding, among which urllib.parse.unquote() Functions can be used to decode URLs. This article will introduce how to use urllib.parse.un
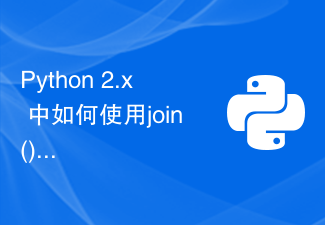
How to use the join() function in Python2.x to merge a list of strings into one string. In Python, we often need to merge multiple strings into one string. Python provides a variety of ways to achieve this goal, one of the common ways is to use the join() function. The join() function can concatenate a list of strings into a string, and can specify the delimiter when concatenating. The basic syntax for using the join() function is as follows: &
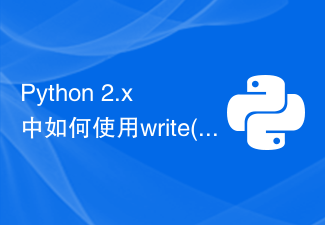
How to use the write() function to write content to a file in Python2.x In Python2.x, we can use the write() function to write content to a file. The write() function is one of the methods of the file object and can be used to write string or binary data to the file. In this article, I will explain in detail how to use the write() function and some common use cases. Open the file Before writing to the file using the write() function, I
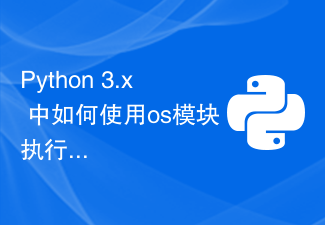
How to use the os module to execute system commands in Python3.x In the standard library of Python3.x, the os module provides a series of methods for executing system commands. In this article, we will learn how to use the os module to execute system commands and give corresponding code examples. The os module in Python is an interface for interacting with the operating system. It provides methods such as executing system commands, accessing files and directories, etc. The following are some commonly used os module methods, which can be used to execute system commands.
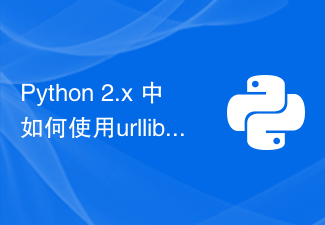
How to use the urllib.quote() function to encode URLs in Python 2.x. URLs contain a variety of characters, including letters, numbers, special characters, etc. In order for the URL to be transmitted and parsed correctly, we need to encode the special characters in it. In Python2.x, you can use the urllib.quote() function to encode the URL. Let's introduce its usage in detail below. urllib.quote
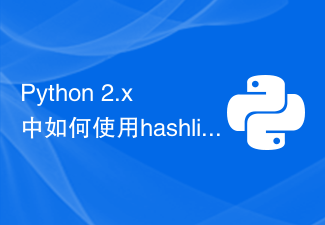
How to use the hashlib module for hash algorithm calculation in Python 2.x. In Python programming, the hash algorithm is a commonly used algorithm used to generate a unique identification of data. Python provides the hashlib module to perform hash algorithm calculations. This article will introduce how to use the hashlib module to perform hash algorithm calculations and give some sample codes. The hashlib module is part of the Python standard library and provides a variety of common hash algorithms, such as MD5, SH
