


How to use time function in Go language to generate calendar and output to HTML file?
How to use the time function in Go language to generate a calendar and output it to an HTML file?
With the development of the Internet, many traditional tools and applications have gradually migrated to electronic devices. Calendar, as an important time management tool, is no exception. Using the time function in the Go language, we can easily generate a calendar and output it as an HTML file, which is convenient for us to view and use on a computer or mobile phone.
To complete this task, we first need to understand the time function of the Go language, which can help us handle date and time related operations. The built-in time package of Go language provides a series of functions, such as Now() to obtain the current time, Parse() to parse a string into time, Format() to format time, etc. In this article, we will use these functions to generate a calendar.
First, we need to create a Go language program file and import the time and os packages. Then, we create a function to generate the HTML snippet for the calendar. The specific code is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
|
In the above code, we define a generateCalendar
function, which generates a calendar HTML code snippet based on the given year and month. First, we use the time.Date
function to get the first day of the month, and then calculate the number of days in the month. Next, we generate the calendar's title and table content based on this information. We then piece this information together to generate a complete calendar table.
In the main
function, we use the time.Now
function to get the current year and month, and call the generateCalendar
function to generate the current month calendar. We then output the calendar to an HTML file named calendar.html
.
To run the above code, you need to install the Go language development environment on your machine. You can use the go run
command to run the code and generate an HTML file named calendar.html
in the same directory.
Now, you can open the calendar.html
file in the browser to view and use the calendar generated by the Go language.
In summary, using the time function in the Go language, we can easily generate a calendar and output it as an HTML file. This simple example shows how to use Go's time functions and string formatting to manipulate dates and times and output the results to an HTML file. I hope the content of this article will be helpful to you in learning and using the Go language!
The above is the detailed content of How to use time function in Go language to generate calendar and output to HTML file?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




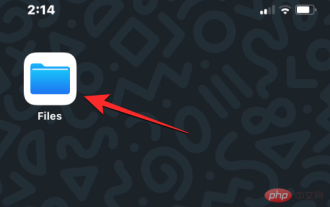
How to Open HTML Files on iPhone HTML files are usually saved in the iPhone’s local storage, so to open them you need to use iOS’s built-in Files app, where you can find all the HTML files you have stored on your iPhone for a long time. If you have an HTML file saved on your iPhone, open the Files app on iOS. When the app opens, tap the Browse tab at the bottom and select On My iPhone under "Locations." In My iPhone, go to the folder or location where you may have saved the HTML file, probably the Downloads folder. find it
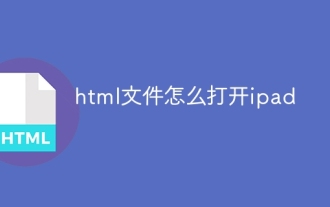
Opening method: 1. With the help of browser software, such as Safari or Chrome. Users can transfer HTML files to iPad and then open them through a browser. 2. Use a file management application (such as local file management or cloud storage service) to open the HTML file. 3. Download an application specifically for viewing and editing HTML files.
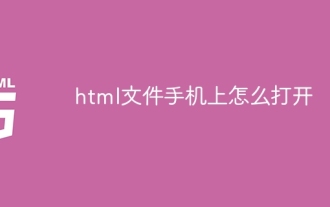
Three methods: 1. Open through a browser, use a special HTML viewer or upload to a web server. 2. Use a dedicated HTML viewer application. It is easiest to open the browser, but the viewer application provides more functions. 3. View through a web server. The web server allows you to access your HTML files through a browser on any device, making it an excellent choice for sharing files or viewing files between different devices.
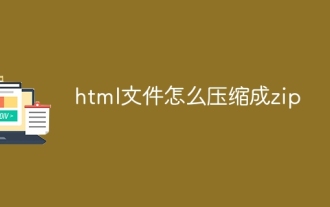
Compressing HTML files into a ZIP file involves gathering the HTML files and their associated resources (such as CSS, JavaScript, images), organizing them into a folder, and using a compression tool (such as Windows' "Send to" function or Mac's " Compress" function) to compress it. The compression process requires considerations such as path validity, file size, and backing up the original files. After successful compression, ZIP files facilitate storage, transmission, and deployment, ensuring file integrity and portability.
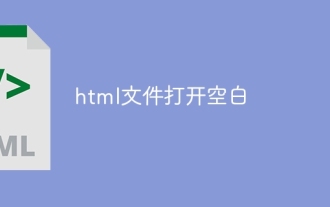
HTML files opening blank is a common problem that can be caused by several reasons. This article introduces common reasons why HTML files open blank, including HTML structure issues, CSS style issues, JavaScript issues, encoding issues, server or network issues, browser compatibility issues, and other reasons. For each reason, this article provides corresponding solutions, including checking DOCTYPE declarations, closing HTML tags, fixing CSS errors, checking JavaScript code, matching file encoding, checking server configuration, clearing browser cache, updating browsers, etc. By following these workarounds, users can troubleshoot and resolve the issue of HTML files opening blank step by step.

Opening steps: 1. Log in to Baidu Netdisk and upload the HTML file; 2. Find the uploaded file in the Netdisk, double-click or right-click and select "Preview" to open it; 3. Baidu Netdisk has a simple built-in file preview function. The content of the HTML file can be previewed in the network disk; 4. The file can be downloaded locally and then opened with a browser to view the complete web page effect.
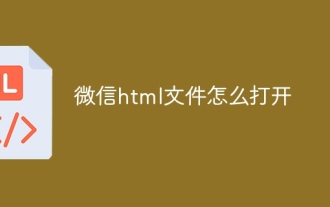
Methods to open WeChat html files: 1. Use the default browser to open; 2. Share the file to the browser; 3. Use a third-party application.
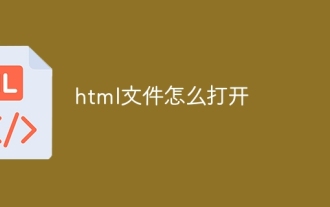
There are several ways to open HTML files. This can be achieved by double-clicking or right-clicking on the browser and selecting the open method. You can also edit using a text editor such as Notepad or a professional development tool such as Visual Studio Code.
