How to implement distributed transaction processing using Redis and C#
How to use Redis and C# to implement distributed transaction processing
Introduction:
With the rapid development of the Internet, distributed systems have become the first choice for many enterprise architectures. Distributed systems can provide greater reliability, scalability, and performance, but they also bring transaction processing challenges. In a distributed system, maintaining data consistency becomes more difficult due to network delays and randomness between different services. This article will introduce how to use Redis and C# to implement distributed transaction processing.
1. Introduction to Redis
Redis is an open source in-memory data structure storage system that can be used as database, cache and message middleware. It supports a variety of data structures such as strings, lists, hashes, and sets, and provides many powerful features such as transaction processing, publish/subscribe, and script execution.
2. Redis transaction processing
Redis implements transaction processing through instructions such as MULTI, EXEC, DISCARD and WATCH. The MULTI instruction is used to start a transaction, the EXEC instruction is used to execute commands in the transaction, the DISCARD instruction is used to cancel a transaction, and the WATCH instruction is used to monitor one or more keys and detect key changes during transaction execution. Cancel transaction.
The following is an example using Redis transaction processing:
using (var redis = ConnectionMultiplexer.Connect("localhost")) { var database = redis.GetDatabase(); var transaction = database.CreateTransaction(); transaction.AddCondition(Condition.HashNotExists("user:1", "name")); transaction.HashSetAsync("user:1", new[] { new HashEntry("name", "Alice"), new HashEntry("age", "25") }); transaction.StringSetAsync("user:1:birthday", "1990-01-01"); var result = transaction.ExecuteAsync(); Console.WriteLine(result.Result); }
In the above example, we used the HashNotExists condition to ensure that the "name" field of user 1 does not exist. If present, the transaction will be canceled.
3. C# implements distributed transaction processing
In distributed systems, distributed transaction processing is very critical. In order to ensure data consistency, we need to coordinate their operations between various services. The following is an example of using C# and Redis to implement distributed transaction processing:
using (var redis = ConnectionMultiplexer.Connect("localhost")) { var database = redis.GetDatabase(); using (var transaction = new TransactionScope()) { // 在此处进行业务逻辑的操作,如数据库插入、更新操作等 database.HashSet("user:1", new[] { new HashEntry("name", "Alice"), new HashEntry("age", "25") }); database.StringSet("user:1:birthday", "1990-01-01"); // 如果需要调用其他服务的话,可以在此处调用 transaction.Complete(); } }
In the above example, we used the TransactionScope class to implement distributed transaction processing. The TransactionScope class automatically coordinates the operations of various services and ensures data consistency.
4. Summary
This article introduces how to use Redis and C# to implement distributed transaction processing. By using Redis's transaction processing and C#'s transaction scope, we can effectively ensure data consistency between various services in a distributed system. This is important for building distributed systems with high reliability, scalability, and performance.
Of course, how to correctly handle distributed transactions still needs to be appropriately adjusted according to specific business scenarios. But through the introduction of this article, you can understand the basic principles and methods of how to use Redis and C# to implement distributed transaction processing, thereby providing some guidance for the design and development of distributed systems.
Reference:
- Redis official website: https://redis.io/
- StackExchange.Redis GitHub repository: https://github.com/ StackExchange/StackExchange.Redis
The above sample code is for reference only. Please make appropriate modifications and extensions according to actual needs. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of How to implement distributed transaction processing using Redis and C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


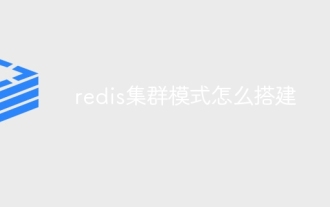
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
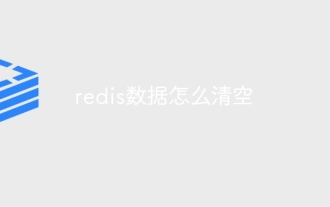
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
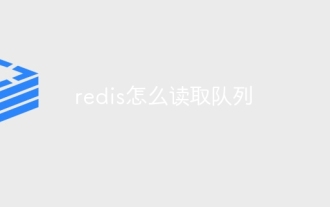
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
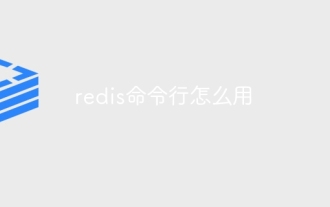
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
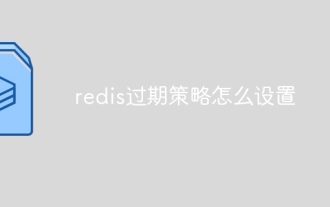
There are two types of Redis data expiration strategies: periodic deletion: periodic scan to delete the expired key, which can be set through expired-time-cap-remove-count and expired-time-cap-remove-delay parameters. Lazy Deletion: Check for deletion expired keys only when keys are read or written. They can be set through lazyfree-lazy-eviction, lazyfree-lazy-expire, lazyfree-lazy-user-del parameters.
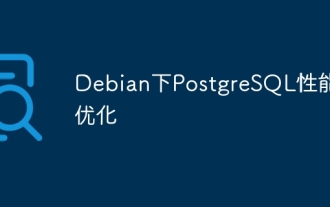
To improve the performance of PostgreSQL database in Debian systems, it is necessary to comprehensively consider hardware, configuration, indexing, query and other aspects. The following strategies can effectively optimize database performance: 1. Hardware resource optimization memory expansion: Adequate memory is crucial to cache data and indexes. High-speed storage: Using SSD SSD drives can significantly improve I/O performance. Multi-core processor: Make full use of multi-core processors to implement parallel query processing. 2. Database parameter tuning shared_buffers: According to the system memory size setting, it is recommended to set it to 25%-40% of system memory. work_mem: Controls the memory of sorting and hashing operations, usually set to 64MB to 256M
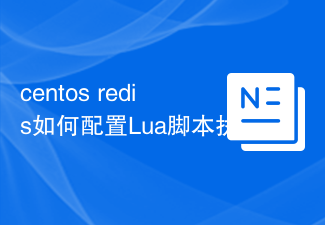
On CentOS systems, you can limit the execution time of Lua scripts by modifying Redis configuration files or using Redis commands to prevent malicious scripts from consuming too much resources. Method 1: Modify the Redis configuration file and locate the Redis configuration file: The Redis configuration file is usually located in /etc/redis/redis.conf. Edit configuration file: Open the configuration file using a text editor (such as vi or nano): sudovi/etc/redis/redis.conf Set the Lua script execution time limit: Add or modify the following lines in the configuration file to set the maximum execution time of the Lua script (unit: milliseconds)
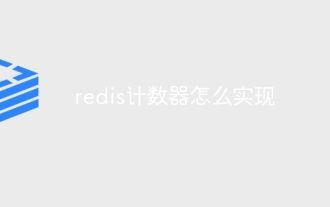
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.
