Building caches for mobile apps using Redis and Objective-C
Using Redis and Objective-C to build cache for mobile applications
In the development of mobile applications, caching is an important part of improving application performance and response speed. Redis is an open source, memory-based high-performance key-value storage system that can be easily integrated with Objective-C language to provide efficient caching solutions for mobile applications. In this article, we will show how to build a cache using Redis and Objective-C to improve the performance of mobile applications.
First, we need to integrate the Redis client in the mobile application. There is a Redis client library called "Hiredis" in Objective-C that can be used to connect and operate Redis services. We can integrate Hiredis into our project through Cocoapods. First, we need to add the following content to the Podfile of the project:
pod 'Hiredis'
Then, run the following command in the project root directory to install the library file:
pod install
After completion, we can start using Hiredis.
First, we need to import the Hiredis header file into the project:
#import <hiredis/hiredis.h>
Next, we create a Redis connection object:
redisContext *context = redisConnect("127.0.0.1", 6379); if (context == NULL || context->err) { if (context) { NSLog(@"Error: %s", context->errstr); // 处理连接错误 } else { NSLog(@"Error: Failed to allocate redis context"); // 处理内存分配错误 } }
In the above code, we use redisConnect
Function connects to the Redis service. If the connection is successful, we will get a non-empty redisContext
object; otherwise, we need to handle it accordingly based on the returned error information.
Now, we can start using Redis for caching operations. The following are some common examples of Redis cache operations:
- Set cache value:
redisReply *reply = redisCommand(context, "SET %s %s", "key", "value"); if (reply->type == REDIS_REPLY_STATUS && reply->integer == 1) { NSLog(@"Success: Cache value is set"); } else { NSLog(@"Error: Failed to set cache value"); } freeReplyObject(reply);
- Get cache value:
redisReply *reply = redisCommand(context, "GET %s", "key"); if (reply->type == REDIS_REPLY_STRING) { NSString *value = [NSString stringWithUTF8String:reply->str]; NSLog(@"Success: Cache value is %@", value); } else { NSLog(@"Error: Failed to get cache value"); } freeReplyObject(reply);
- Delete cached value:
redisReply *reply = redisCommand(context, "DEL %s", "key"); if (reply->type == REDIS_REPLY_INTEGER && reply->integer == 1) { NSLog(@"Success: Cache value is deleted"); } else { NSLog(@"Error: Failed to delete cache value"); } freeReplyObject(reply);
In addition to the above examples, Redis also supports many other cache operations, such as determining whether the cache exists, setting the cache expiration time, etc. We can choose the appropriate operation according to actual needs.
In addition, in order to avoid the need to establish a connection every time we access Redis, we can create a singleton class to manage the Redis connection object. The following is a simple example of a singleton class:
@implementation RedisManager + (instancetype)sharedInstance { static RedisManager *instance = nil; static dispatch_once_t onceToken; dispatch_once(&onceToken, ^{ instance = [[RedisManager alloc] init]; }); return instance; } - (instancetype)init { self = [super init]; if (self) { _context = redisConnect("127.0.0.1", 6379); if (_context == NULL || _context->err) { if (_context) { NSLog(@"Error: %s", _context->errstr); // 处理连接错误 } else { NSLog(@"Error: Failed to allocate redis context"); // 处理内存分配错误 } return nil; } } return self; } @end
In the above code, we ensure that the singleton object is only created once through dispatch_once
and establish the Redis connection in the initialization method.
With the above sample code, we can quickly build a cache system and integrate it into mobile applications. By properly using the caching function of Redis, we can effectively reduce the number of requests to the back-end server and improve the performance and user experience of mobile applications.
To summarize, using Redis and Objective-C to build cache for mobile applications is an effective performance optimization method. By properly using the caching function of Redis, we can improve the response speed and user experience of mobile applications. I hope this article can be helpful to everyone's performance optimization work when building mobile applications.
(Note: The above examples are for demonstration purposes only. Actual use requires corresponding adjustments and optimizations based on specific needs and situations.)
The above is the detailed content of Building caches for mobile apps using Redis and Objective-C. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
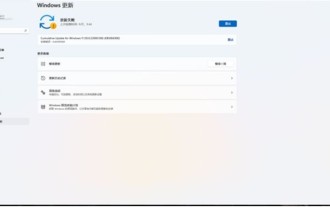
1. Start the [Start] menu, enter [cmd], right-click [Command Prompt], and select Run as [Administrator]. 2. Enter the following commands in sequence (copy and paste carefully): SCconfigwuauservstart=auto, press Enter SCconfigbitsstart=auto, press Enter SCconfigcryptsvcstart=auto, press Enter SCconfigtrustedinstallerstart=auto, press Enter SCconfigwuauservtype=share, press Enter netstopwuauserv , press enter netstopcryptS
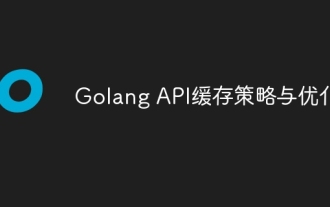
The caching strategy in GolangAPI can improve performance and reduce server load. Commonly used strategies are: LRU, LFU, FIFO and TTL. Optimization techniques include selecting appropriate cache storage, hierarchical caching, invalidation management, and monitoring and tuning. In the practical case, the LRU cache is used to optimize the API for obtaining user information from the database. The data can be quickly retrieved from the cache. Otherwise, the cache can be updated after obtaining it from the database.
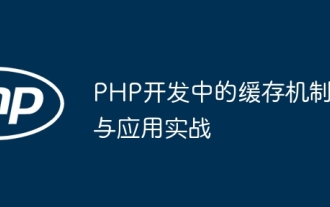
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.
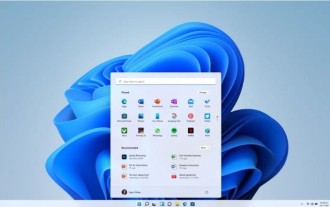
First you need to set the system language to Simplified Chinese display and restart. Of course, if you have changed the display language to Simplified Chinese before, you can just skip this step. Next, start operating the registry, regedit.exe, directly navigate to HKEY_LOCAL_MACHINESYSTEMCurrentControlSetControlNlsLanguage in the left navigation bar or the upper address bar, and then modify the InstallLanguage key value and Default key value to 0804 (if you want to change it to English en-us, you need First set the system display language to en-us, restart the system and then change everything to 0409) You must restart the system at this point.
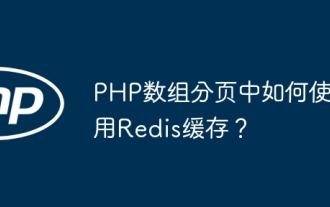
Using Redis cache can greatly optimize the performance of PHP array paging. This can be achieved through the following steps: Install the Redis client. Connect to the Redis server. Create cache data and store each page of data into a Redis hash with the key "page:{page_number}". Get data from cache and avoid expensive operations on large arrays.

Yes, Navicat can connect to Redis, which allows users to manage keys, view values, execute commands, monitor activity, and diagnose problems. To connect to Redis, select the "Redis" connection type in Navicat and enter the server details.
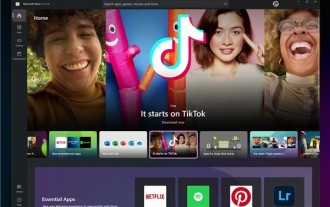
1. First, double-click the [This PC] icon on the desktop to open it. 2. Then double-click the left mouse button to enter [C drive]. System files will generally be automatically stored in C drive. 3. Then find the [windows] folder in the C drive and double-click to enter. 4. After entering the [windows] folder, find the [SoftwareDistribution] folder. 5. After entering, find the [download] folder, which contains all win11 download and update files. 6. If we want to delete these files, just delete them directly in this folder.
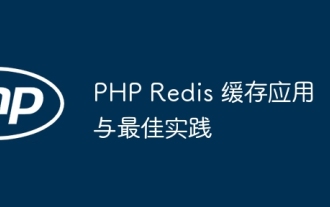
Redis is a high-performance key-value cache. The PHPRedis extension provides an API to interact with the Redis server. Use the following steps to connect to Redis, store and retrieve data: Connect: Use the Redis classes to connect to the server. Storage: Use the set method to set key-value pairs. Retrieval: Use the get method to obtain the value of the key.
