How to use Redis and Java to implement distributed current limiting function
How to use Redis and Java to implement distributed current limiting function
Introduction:
With the rapid development of the Internet, the number of concurrent requests in the system is also increasing. Current limiting in high concurrency scenarios The questions are becoming more and more important. In distributed systems, how to implement effective current limiting strategies and protect the stability and performance of the system has become an urgent problem for developers to solve. This article will introduce how to use Redis and Java to implement distributed current limiting function, and give some code examples.
1. Introduction to Redis:
Redis is an open source memory-based data structure storage system with high performance, high availability and flexibility. Redis supports a variety of data structures, such as strings, hash tables, lists, sets, ordered sets, etc., and provides a rich set of instructions for operating on these data structures. It also provides advanced functions such as publish/subscribe, transactions, and persistence, allowing developers to respond to various scenarios more flexibly.
2. Current limiting algorithm:
The current limiting algorithm refers to limiting the number of concurrent requests accepted by the system within a certain period of time to prevent the system from being overwhelmed by too many requests and affecting the stability and performance of the system. . Common current limiting algorithms include counters, sliding windows, and token buckets. Below we will use code examples to implement the distributed current limiting function of the sliding window algorithm.
3. Code example:
-
First, we need to introduce the Java client library of Redis, such as Jedis.
import redis.clients.jedis.Jedis;
Copy after login Initialize Redis connection:
Jedis jedis = new Jedis("localhost", 6379);
Copy after loginDefine a method for current limiting, which requires passing in an identifier (such as IP address) and a time window size:
public boolean limitAccess(String identifier, int windowSize) { long currentTime = System.currentTimeMillis(); String key = identifier + ":" + currentTime / 1000; // 按时间窗口划分key long count = jedis.incr(key); // 原子操作,每次增加1 if (count == 1) { jedis.expire(key, windowSize); // 设置过期时间 } if (count > 10) { // 设置最大请求数 return false; } return true; }
Copy after loginCall this method at the entrance of the system to determine the current limit:
public void processRequest(String identifier) { int windowSize = 60; // 设置时间窗口大小为60秒 boolean isAllowed = limitAccess(identifier, windowSize); if (isAllowed) { // 处理请求 } else { // 返回限流提示 } }
Copy after login
4. Summary :
This article introduces how to use Redis and Java to implement distributed current limiting function, and gives a code example of the sliding window algorithm. By using Redis as a distributed caching and counting tool, we can easily implement a variety of current limiting algorithms and enhance the stability and performance of the system. Of course, in actual scenarios, it is necessary to choose an appropriate current limiting strategy based on specific needs and business characteristics to achieve the best results.
References:
- Redis official website: https://redis.io/
- Jedis GitHub page: https://github.com/redis/ jedis
The above is the detailed content of How to use Redis and Java to implement distributed current limiting function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


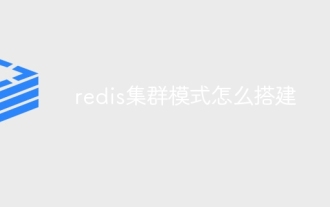
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
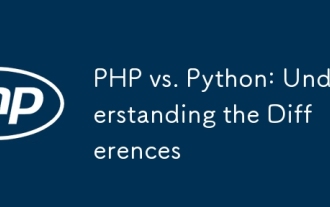
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
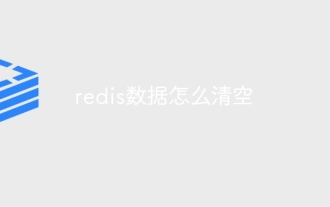
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
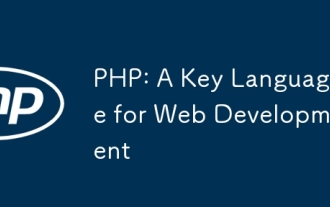
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
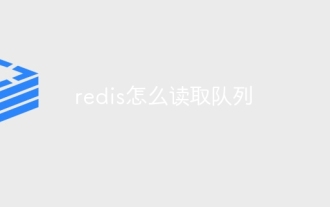
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
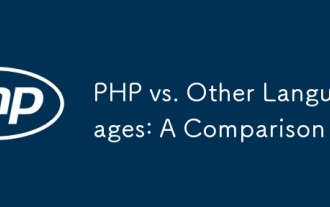
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
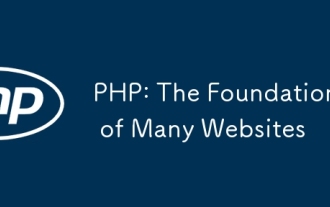
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
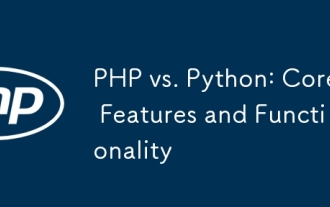
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
