


How to parse the data returned by the API using JSON processing functions in Go language?
How to use the JSON processing function in Go language to parse the data returned by the API?
1. Introduction
Modern web applications often rely on RESTful APIs to obtain data. Many APIs will return data in JSON format, so when writing web applications using Go language, we often need to process JSON data.
In the Go language, JSON data can be processed through the encoding/json
package provided by the standard library. This package has powerful features that help us easily parse the data returned by the API.
2. Parse the JSON data returned by the API
Suppose we call an API that returns the following data in JSON format:
{ "name": "John", "age": 25, "email": "john@example.com" }
We can define a structure to represent this The structure of JSON data:
type Person struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` }
Then, we can use the json.Unmarshal()
function to parse the JSON data returned by the API:
import ( "encoding/json" "fmt" ) func main() { jsonData := []byte(`{ "name": "John", "age": 25, "email": "john@example.com" }`) var person Person err := json.Unmarshal(jsonData, &person) if err != nil { fmt.Println("解析JSON数据失败:", err) return } fmt.Println("名称:", person.Name) fmt.Println("年龄:", person.Age) fmt.Println("邮箱:", person.Email) }
The output result is:
名称: John 年龄: 25 邮箱: john@example.com
3. Processing the JSON array returned by the API
Sometimes, the data returned by the API may be a JSON array. For example, suppose we call an API that returns a list of users, and it returns the following data in JSON format:
[ { "name": "John", "age": 25, "email": "john@example.com" }, { "name": "Alice", "age": 28, "email": "alice@example.com" } ]
We can define a structure slice corresponding to the JSON array:
type Person struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` } type PersonList []Person
Then , we can use the json.Unmarshal()
function to parse the JSON array into slices:
import ( "encoding/json" "fmt" ) func main() { jsonData := []byte(`[ { "name": "John", "age": 25, "email": "john@example.com" }, { "name": "Alice", "age": 28, "email": "alice@example.com" } ]`) var personList PersonList err := json.Unmarshal(jsonData, &personList) if err != nil { fmt.Println("解析JSON数据失败:", err) return } for i, person := range personList { fmt.Printf("用户%d: ", i+1) fmt.Println("名称:", person.Name) fmt.Println("年龄:", person.Age) fmt.Println("邮箱:", person.Email) fmt.Println("---------") } }
The output result is:
用户1: 名称: John 年龄: 25 邮箱: john@example.com --------- 用户2: 名称: Alice 年龄: 28 邮箱: alice@example.com ---------
4. Summary
Using Go language The encoding/json
package makes it easy to parse the JSON data returned by the API. We only need to define the structure corresponding to the JSON data, and then use the json.Unmarshal()
function to parse the JSON data into a Go language data structure. In this way, we can easily process the data obtained from the API, making the web application more powerful and flexible.
The above is the detailed content of How to parse the data returned by the API using JSON processing functions in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


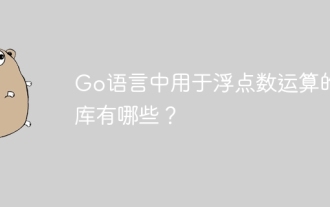
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
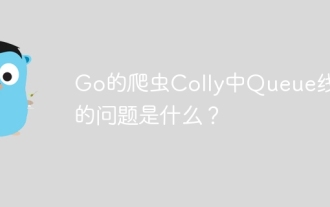
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
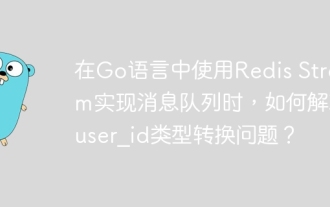
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
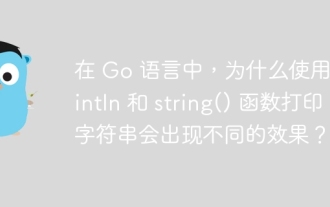
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
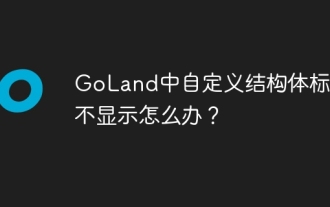
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
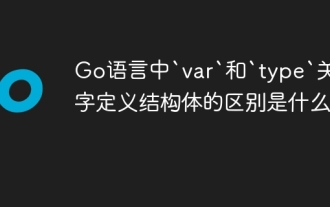
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
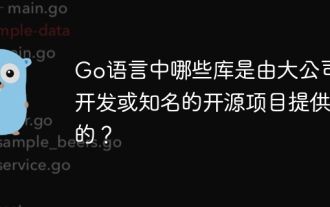
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
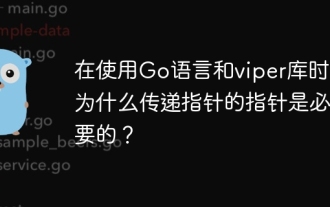
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
