How to implement file upload in Zend framework
How to implement file upload in Zend framework
Uploading files is one of the common functions in web applications. In the Zend framework, the file upload function can be easily implemented by using ZendForm and ZendInputFilter. This article will introduce how to implement file upload in Zend framework and provide corresponding code examples.
First, we need to create a form for uploading files. Custom form classes can be created by inheriting ZendForm. In the form, we need to add a form element of type file and set the name attribute of the form element so that the uploaded file can be obtained in the controller later.
use ZendFormElement; use ZendFormForm; class UploadForm extends Form { public function __construct($name = null) { parent::__construct($name); // 添加文件上传元素 $file = new ElementFile('file'); $file->setLabel('选择文件') ->setAttribute('id', 'file-upload'); $this->add($file); // 添加提交按钮 $submit = new ElementSubmit('submit'); $submit->setValue('上传'); $this->add($submit); } }
Next, we need to handle the file upload in the controller. We can verify and filter uploaded files through ZendInputFilter. First, you need to instantiate ZendInputFilterInputFilter and add input filtering of file type. Then, the uploaded file can be verified through ZendValidatorFile, such as file size, file type, etc. Finally, we need to save the file to the specified directory in the controller.
use ZendMvcControllerAbstractActionController; use ZendViewModelViewModel; use ZendInputFilterInputFilter; use ZendValidatorFileSize; use ZendValidatorFileMimeType; class UploadController extends AbstractActionController { public function indexAction() { // 创建文件上传表单 $form = new UploadForm(); $request = $this->getRequest(); if ($request->isPost()) { // 实例化输入过滤器 $inputFilter = new InputFilter(); // 添加文件类型的输入过滤 $fileInput = new InputFilterFileInput('file'); $fileInput->setRequired(true); $fileInput->getValidatorChain() ->attach(new Size(['max' => '2MB'])) ->attach(new MimeType(['image/jpeg', 'image/png'])); $inputFilter->add($fileInput); // 将过滤后的数据绑定到表单 $form->setInputFilter($inputFilter); $form->setData($request->getPost()); // 检查表单数据是否有效 if ($form->isValid()) { // 获取上传的文件 $file = $form->get('file')->getValue(); // 保存文件到指定目录 $destination = '/path/to/save/uploaded/files/' . $file['name']; move_uploaded_file($file['tmp_name'], $destination); // 重定向到成功页面 return $this->redirect()->toRoute('upload', ['action' => 'success']); } } return new ViewModel([ 'form' => $form ]); } }
Finally, display the form in the view and process the results of the file upload. The ZendFormViewHelperForm tag can be used in the view to generate the HTML code of the form.
<h2>文件上传</h2> <?= $this->form()->openTag($form) ?> <?= $this->formRow($form->get('file')) ?> <?= $this->formSubmit($form->get('submit')) ?> <?= $this->form()->closeTag() ?>
Through the above steps, we can implement the file upload function in the Zend framework. By using ZendForm and ZendInputFilter, we can easily verify and filter uploaded files and save the files to the specified directory. Code examples in controllers and views can help you better understand and implement file upload functionality. I wish you success in developing with Zend Framework!
The above is the detailed content of How to implement file upload in Zend framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


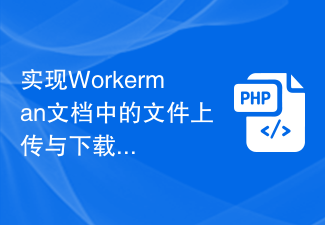
To implement file upload and download in Workerman documents, specific code examples are required. Introduction: Workerman is a high-performance PHP asynchronous network communication framework that is simple, efficient, and easy to use. In actual development, file uploading and downloading are common functional requirements. This article will introduce how to use the Workerman framework to implement file uploading and downloading, and give specific code examples. 1. File upload: File upload refers to the operation of transferring files on the local computer to the server. The following is used
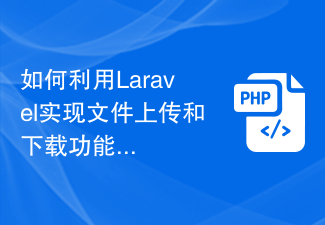
How to use Laravel to implement file upload and download functions Laravel is a popular PHP Web framework that provides a wealth of functions and tools to make developing Web applications easier and more efficient. One of the commonly used functions is file upload and download. This article will introduce how to use Laravel to implement file upload and download functions, and provide specific code examples. File upload File upload refers to uploading local files to the server for storage. In Laravel we can use file upload
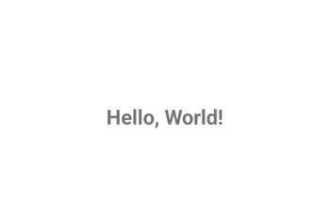
Polling in Android is a key technology that allows applications to retrieve and update information from a server or data source at regular intervals. By implementing polling, developers can ensure real-time data synchronization and provide the latest content to users. It involves sending regular requests to a server or data source and getting the latest information. Android provides multiple mechanisms such as timers, threads, and background services to complete polling efficiently. This enables developers to design responsive and dynamic applications that stay in sync with remote data sources. This article explores how to implement polling in Android. It covers the key considerations and steps involved in implementing this functionality. Polling The process of periodically checking for updates and retrieving data from a server or source is called polling in Android. pass
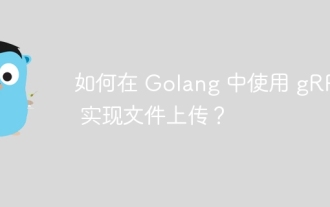
How to implement file upload using gRPC? Create supporting service definitions, including request and response messages. On the client, the file to be uploaded is opened and split into chunks, then streamed to the server via a gRPC stream. On the server side, file chunks are received and stored into a file. The server sends a response after the file upload is completed to indicate whether the upload was successful.
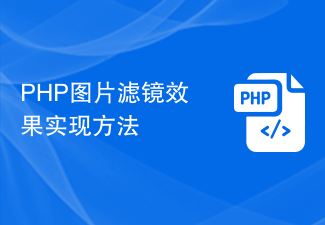
How to implement PHP image filter effects requires specific code examples. Introduction: In the process of web development, image filter effects are often used to enhance the vividness and visual effects of images. The PHP language provides a series of functions and methods to achieve various picture filter effects. This article will introduce some commonly used picture filter effects and their implementation methods, and provide specific code examples. 1. Brightness adjustment Brightness adjustment is a common picture filter effect, which can change the lightness and darkness of the picture. By using imagefilte in PHP
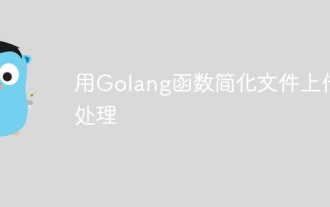
Answer: Yes, Golang provides functions that simplify file upload processing. Details: The MultipartFile type provides access to file metadata and content. The FormFile function gets a specific file from the form request. The ParseForm and ParseMultipartForm functions are used to parse form data and multipart form data. Using these functions simplifies the file processing process and allows developers to focus on business logic.
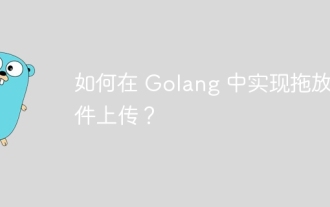
How to implement drag and drop file upload in Golang? Enable middleware; handle file upload requests; create HTML code for the drag and drop area; add JavaScript code for handling drag and drop events.
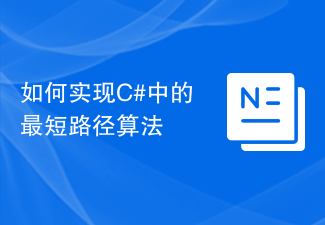
How to implement the shortest path algorithm in C# requires specific code examples. The shortest path algorithm is an important algorithm in graph theory and is used to find the shortest path between two vertices in a graph. In this article, we will introduce how to use C# language to implement two classic shortest path algorithms: Dijkstra algorithm and Bellman-Ford algorithm. Dijkstra's algorithm is a widely used single-source shortest path algorithm. Its basic idea is to start from the starting vertex, gradually expand to other nodes, and update the discovered nodes.
