How to use Python to develop the connection pool function of Redis
How to use Python to develop the connection pool function of Redis
Redis is a high-performance memory-based key-value database that is often used in applications such as caching and message queues. In Python, we can use the redis-py library to interact with Redis. In order to improve connection efficiency and performance, we can use the connection pool function of Redis. This article will introduce how to use Python to develop the connection pool function of Redis.
First, we need to install the redis-py library, which can be installed using the pip command:
pip install redis
Next, we can create a Redis connection pool through the following code:
import redis pool = redis.ConnectionPool(host='localhost', port=6379, db=0, max_connections=10)
In the above code, we create a connection pool through the ConnectionPool function of the redis module. The host
parameter specifies the host address of Redis, the port
parameter specifies the port number of Redis, the db
parameter specifies the Redis database number, the max_connections
parameter Specifies the maximum number of connections for the connection pool.
Then, we can obtain a Redis connection through the following code:
conn = redis.Redis(connection_pool=pool)
In the above code, we obtain the Redis connection object through the Redis function of the redis module. connection_pool
The parameter specifies the previously created connection pool object.
Next, we can use the obtained Redis connection object to perform Redis operations, such as reading and writing data:
# 写入数据 conn.set('key', 'value') # 读取数据 value = conn.get('key') print(value)
In the above code, we use set The
method writes a key-value pair into Redis, and the get
method is used to read the value corresponding to the specified key from Redis.
Finally, we need to return the connection to the connection pool after using the Redis connection so that other code can be reused:
pool.release(conn)
In the above code, we pass the ## of the connection pool object The #release method returns the previously acquired connection to the connection pool.
import redis # 创建连接池 pool = redis.ConnectionPool(host='localhost', port=6379, db=0, max_connections=10) # 获取Redis连接 conn = redis.Redis(connection_pool=pool) # 写入数据 conn.set('key', 'value') # 读取数据 value = conn.get('key') print(value) # 归还连接至连接池 pool.release(conn)
The above is the detailed content of How to use Python to develop the connection pool function of Redis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




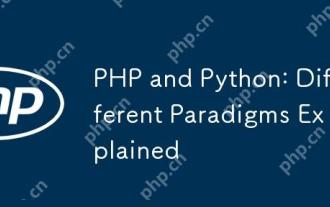
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
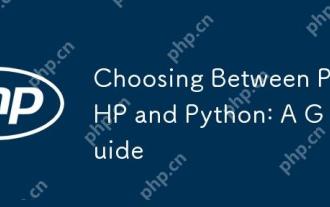
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
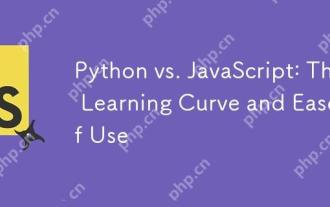
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
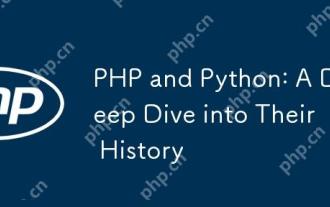
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
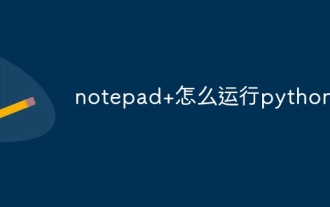
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
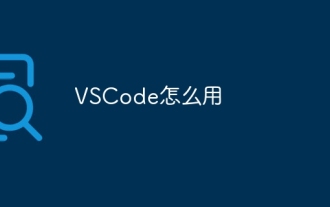
Visual Studio Code (VSCode) is a cross-platform, open source and free code editor developed by Microsoft. It is known for its lightweight, scalability and support for a wide range of programming languages. To install VSCode, please visit the official website to download and run the installer. When using VSCode, you can create new projects, edit code, debug code, navigate projects, expand VSCode, and manage settings. VSCode is available for Windows, macOS, and Linux, supports multiple programming languages and provides various extensions through Marketplace. Its advantages include lightweight, scalability, extensive language support, rich features and version
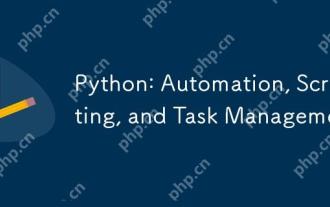
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
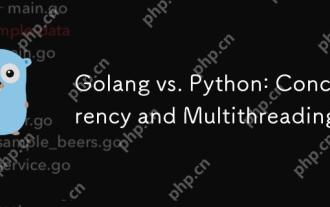
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
