How to implement request logging and monitoring in FastAPI
How to implement request logging and monitoring in FastAPI
Introduction:
FastAPI is a high-performance web framework based on Python 3.7, which provides many powerful functions and features, including automated requests and response model verification, security, performance optimization, etc. In actual development, we often need to record request logs in the application for debugging and monitoring analysis. This article will introduce how to implement request logging and monitoring in FastAPI and provide corresponding code examples.
1. Install dependency packages
Before we start, we need to install some necessary dependency packages. Open the terminal and execute the following command:
1 |
|
Among them, loguru is an easy-to-use logging library, we will use it to record request logs.
2. Create a FastAPI application
First, we need to create a FastAPI application. In the project directory, create a file named main.py and write the following code:
1 2 3 4 5 6 7 |
|
3. Record the request log
Next, we will use the loguru library to record the request log. Add the following code to the main.py file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
In the above code, we first import the logger object of the loguru library and add a file logger. We specified the path of the log file as logs/request.log and set the maximum size of the log file to 10MB. Then, in the root() function, we use the logger.info() method to log the request.
4. Start the application
Save the main.py file and return to the terminal, execute the following command to start the FastAPI application:
1 |
|
The terminal will output the access URL of the application, such as http: //127.0.0.1:8000. Accessing this URL in the browser we will see the "Hello World" message. Open the logs/request.log file and we will see the request log records.
5. Monitoring requests
In addition to recording request logs, we can also monitor the processing time and status code of the request. In order to implement this function, we need to use the Middleware provided by FastAPI. Add the following code to the main.py file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
|
In the above code, we first import the time module and add a sleep time to the root() function to simulate the request processing time. Then, we added the logic to calculate the request processing time and record the request status code in the log_request() middleware function. In the shutdown_event() function, we delete the previously added logger.
Now, restart the FastAPI application and visit the application URL in your browser. Refresh the page in the browser and open the logs/request.log file. We will see the request log records including the request method, URL, status code and processing time.
Conclusion:
This article introduces how to implement request logging and monitoring in FastAPI. We use the loguru library to record request logs, and use FastAPI's Middleware to monitor the request processing time and status code. These features allow us to better track and monitor application requests and responses. The above is a code example to implement request logging and monitoring.
Reference materials:
- FastAPI official documentation: https://fastapi.tiangolo.com/
- loguru official documentation: https://loguru.readthedocs. io/
The above is the detailed content of How to implement request logging and monitoring in FastAPI. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




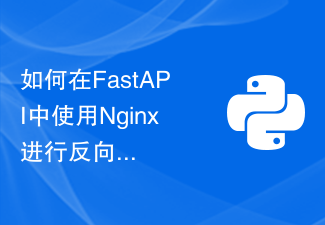
How to use Nginx with FastAPI for reverse proxy and load balancing Introduction: FastAPI and Nginx are two very popular web development tools. FastAPI is a high-performance Python framework, and Nginx is a powerful reverse proxy server. Using these two tools together can improve the performance and reliability of your web applications. In this article, we will learn how to use Nginx with FastAPI for reverse proxy and load balancing. What is reverse generation
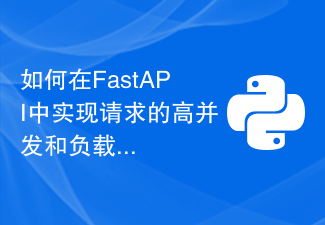
How to achieve high concurrency and load balancing of requests in FastAPI Introduction: With the development of the Internet, high concurrency of web applications has become a common problem. When handling a large number of requests, we need to use efficient frameworks and technologies to ensure system performance and scalability. FastAPI is a high-performance Python framework that can help us achieve high concurrency and load balancing. This article will introduce how to use FastAPI to achieve high concurrency and load balancing of requests. We will use Python3.7
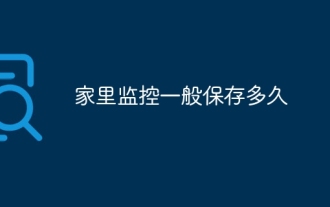
Home monitoring is generally kept for one to two weeks. Detailed introduction: 1. The larger the storage capacity, the longer the video can be saved; 2. The larger the capacity of the hard disk, the longer the video can be saved; 3. According to the requirements of different regions and laws and regulations, the number of surveillance videos The storage time may vary; 4. Some advanced surveillance systems can also trigger recording based on motion detection or specific events, thereby saving storage space and providing more useful recordings.
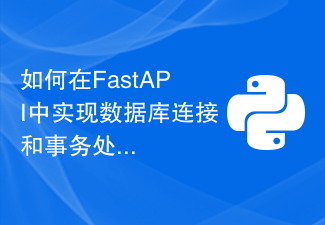
How to implement database connection and transaction processing in FastAPI Introduction: With the rapid development of web applications, database connection and transaction processing have become a very important topic. FastAPI is a high-performance Python web framework loved by developers for its speed and ease of use. In this article, we will introduce how to implement database connections and transactions in FastAPI to help you build reliable and efficient web applications. Part 1: Database connection in FastA
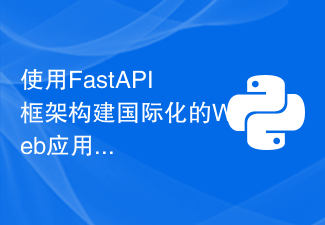
Use the FastAPI framework to build international Web applications. FastAPI is a high-performance Python Web framework that combines Python type annotations and high-performance asynchronous support to make developing Web applications simpler, faster, and more reliable. When building an international Web application, FastAPI provides convenient tools and concepts that can make the application easily support multiple languages. Below I will give a specific code example to introduce how to use the FastAPI framework to build
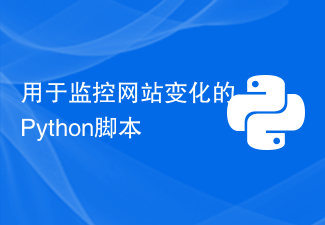
In today's digital age, being aware of the latest changes on your website is crucial for a variety of purposes, such as tracking updates on your competitors' websites, monitoring product availability, or staying informed of important information. Manually checking your website for changes can be time-consuming and inefficient. This is where automation comes into play. In this blog post, we will explore how to create a Python script to monitor website changes. By leveraging the power of Python and some handy libraries, we can automate the process of retrieving website content, comparing it to previous versions, and notifying us of any changes. This allows us to remain proactive and react promptly to updates or modifications to the sites we monitor. Setting up the environment Before we start writing scripts to monitor website changes, we need to set up P
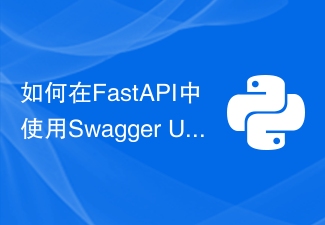
How to use SwaggerUI to display API documentation in FastAPI Introduction: In modern web development, API is an integral part. In order to facilitate development and maintenance, we need to provide a friendly and easy-to-use API documentation so that other developers can understand and use our API. Swagger is a popular API documentation format and tool that provides an interactive UI interface that can visually display the details of the API. In this article I will show you how to use Fas
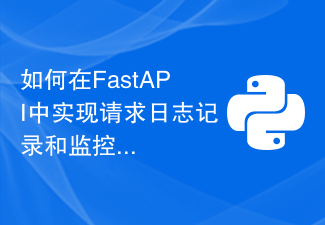
How to implement request logging and monitoring in FastAPI Introduction: FastAPI is a high-performance web framework based on Python3.7+. It provides many powerful functions and features, including automated request and response model verification, security, and performance optimization. wait. In actual development, we often need to record request logs in the application for debugging and monitoring analysis. This article will introduce how to implement request logging and monitoring in FastAPI and provide corresponding code examples. 1. Installation
