How to connect to Sybase database using PDO
How to use PDO to connect to the Sybase database
Sybase database is a relational database management system commonly used in enterprise-level applications. In order to connect and operate the Sybase database, we can use the PDO extension in PHP. PDO (PHP Data Objects) provides a unified interface to connect to different databases, including Sybase.
Here is some sample code to help you connect to a Sybase database using PDO.
Step 1: Install and enable the PDO extension
First, make sure that the PDO extension has been installed in your PHP environment and the Sybase database driver is enabled. You can find the following two lines in the php.ini file and make sure they are not commented out:
extension=php_pdo.dll extension=php_pdo_sybase.dll
Step 2: Connect to the Sybase database
Next, we need to use PDO to connect to the Sybase database. First, create a PDO object and pass in the connection parameters of the database. The connection string format of Sybase is: sybase:host=hostname;dbname=database
try { $dsn = 'sybase:host=hostname;dbname=database'; $username = 'username'; $password = 'password'; $pdo = new PDO($dsn, $username, $password); echo 'Connected to Sybase database successfully!'; } catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage(); }
Step 3: Execute the query statement
After connecting to the database, we can use the PDO object to execute SQL query statement. The following is a simple SELECT query example:
try { // 创建一个PDO对象连接到数据库 $pdo = new PDO($dsn, $username, $password); // 准备查询语句 $query = 'SELECT * FROM table_name'; // 执行查询 $result = $pdo->query($query); // 处理查询结果 foreach ($result as $row) { echo $row['column_name'] . '<br>'; } } catch (PDOException $e) { echo 'Query failed: ' . $e->getMessage(); }
Step 4: Insert and update data
In addition to SELECT queries, we can also use PDO objects to execute INSERT and UPDATE statements to insert and update data in the database data.
try { // 创建一个PDO对象连接到数据库 $pdo = new PDO($dsn, $username, $password); // 准备插入语句 $query = 'INSERT INTO table_name (column1, column2) VALUES (:value1, :value2)'; // 准备数据 $values = array( 'value1' => 'Foo', 'value2' => 'Bar' ); // 执行插入语句 $pdo->prepare($query)->execute($values); echo 'Data inserted successfully!'; } catch (PDOException $e) { echo 'Insert failed: ' . $e->getMessage(); }
try { // 创建一个PDO对象连接到数据库 $pdo = new PDO($dsn, $username, $password); // 准备更新语句 $query = 'UPDATE table_name SET column1 = :value1 WHERE column2 = :value2'; // 准备数据 $values = array( 'value1' => 'NewValue', 'value2' => 'OldValue' ); // 执行更新语句 $pdo->prepare($query)->execute($values); echo 'Data updated successfully!'; } catch (PDOException $e) { echo 'Update failed: ' . $e->getMessage(); }
When using PDO to connect to the Sybase database, please ensure that you have correctly configured and entered the database connection parameters. Additionally, it is recommended to store database credentials in a safe place and use secure methods in your code to obtain the credential data.
Hope the above sample code can help you successfully connect and operate the Sybase database!
The above is the detailed content of How to connect to Sybase database using PDO. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


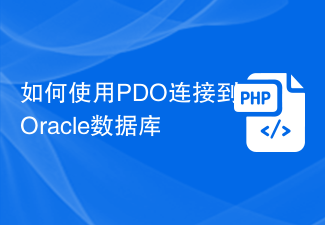
Overview of how to use PDO to connect to Oracle database: PDO (PHPDataObjects) is an extension library for operating databases in PHP. It provides a unified API to access multiple types of databases. In this article, we will discuss how to use PDO to connect to an Oracle database and perform some common database operations. Step: Install the Oracle database driver extension. Before using PDO to connect to the Oracle database, we need to install the corresponding Oracle
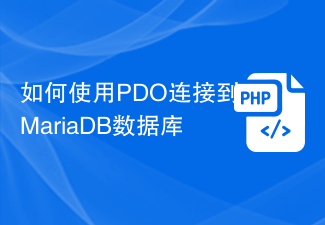
How to use PDO to connect to MariaDB database 1. Introduction PDO (PHPDataObjects) is a lightweight abstraction layer used in PHP to access the database. It provides developers with a unified set of interfaces to connect and operate different types of databases, including MariaDB, MySQL, SQLite, etc. This article will introduce how to use PDO to connect to the MariaDB database and give sample code. 2. Install and configure using PDO to connect to MariaDB
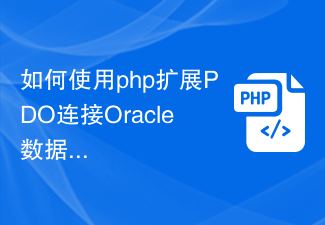
How to use PHP to extend PDO to connect to Oracle database Introduction: PHP is a very popular server-side programming language, and Oracle is a commonly used relational database management system. This article will introduce how to use PHP extension PDO (PHPDataObjects) to connect to Oracle database. 1. Install the PDO_OCI extension. To connect to the Oracle database, you first need to install the PDO_OCI extension. Here are the steps to install the PDO_OCI extension: Make sure
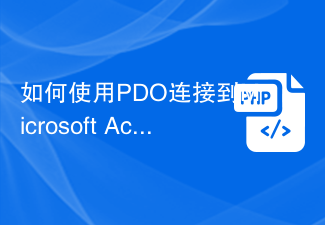
How to use PDO to connect to the Microsoft Access database Microsoft Access is a commonly used relational database management system that provides a user-friendly graphical interface and powerful data management functions. For many developers, using PHP to connect to a Microsoft Access database is a challenge. However, by using PHP's PDO (PHPDataObject) extension, connecting to an Access database becomes quite
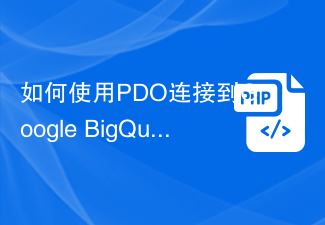
How to connect to the Google BigQuery database using PDO Google BigQuery is a fully managed cloud data warehouse solution that provides powerful data analysis and query capabilities. PDO is a database abstraction layer of PHP that allows us to interact with various databases more conveniently. This article will teach you how to use PDO to connect to the Google BigQuery database and provide corresponding code examples. Configure the Google Cloud project first
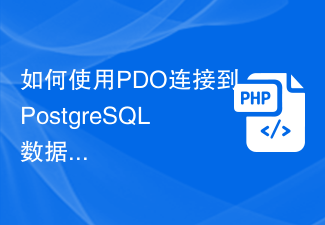
How to connect to a PostgreSQL database using PDO Introduction: When developing web applications using PHP, the database is an essential part. Using PDO (PHPDataObjects) for database operations is a widely used method, which provides a simple, efficient, and safe database operation method. This article explains how to use PDO to connect to a PostgreSQL database and provides corresponding code examples. 1. Install and configure PostgreSQL data
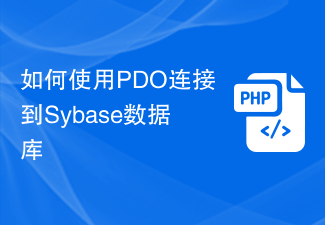
How to use PDO to connect to the Sybase database Sybase database is a relational database management system commonly used in enterprise-level applications. In order to connect and operate the Sybase database, we can use the PDO extension in PHP. PDO (PHPDataObjects) provides a unified interface to connect to different databases, including Sybase. Below is some sample code to help you connect to a Sybase database using PDO. Step1:Install and enable PD
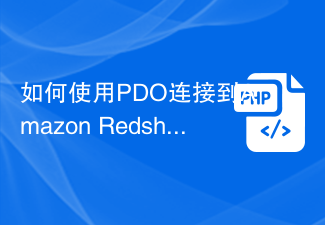
How to use PDO to connect to the Amazon Redshift database Amazon Redshift is a scalable, high-performance cloud data warehouse service commonly used to handle large-scale data analysis and report generation. In PHP development, you can use the PDO extension to connect to the Amazon Redshift database and perform data read and write operations. This article explains how to connect using PDO and provides corresponding code examples. Step 1: Install PDO extension and AmazonR
