


Building a real-time monitoring system using Redis and Golang: How to process large amounts of data quickly
Building a real-time monitoring system using Redis and Golang: How to quickly process large amounts of data
With the rapid development of the Internet and the continuous advancement of technology, the explosive growth of data volume has become a major challenge we face. In order to better monitor and process large amounts of data in real time, we can use the combination of Redis and Golang to build an efficient real-time monitoring system.
Redis is a high-performance in-memory database that supports a variety of data structures, such as strings, hashes, lists, sets, and ordered sets. Golang is an efficient programming language with concurrent programming and high performance features.
This article will introduce how to use Redis and Golang to build a real-time monitoring system, and show how to quickly process large amounts of data. First, we need to determine the indicators and data types that the monitoring system needs to monitor. We can then use Redis' ordered set data structure to store and process this data.
First, we create an ordered collection named "monitor" to store real-time data and timestamps. We can use the following code to achieve this:
package main import ( "fmt" "time" "github.com/go-redis/redis" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 这里根据实际情况填写密码 DB: 0, // 默认数据库 }) // 设置当前时间戳 timestamp := time.Now().Unix() // 添加数据到有序集合 client.ZAdd("monitor", &redis.Z{ Member: "data1", Score: float64(timestamp), }) client.ZAdd("monitor", &redis.Z{ Member: "data2", Score: float64(timestamp), }) // 查询最新的数据 res, _ := client.ZRevRangeByScore("monitor", &redis.ZRangeBy{ Min: "-inf", Max: "+inf", Offset: 0, Count: 1, }).Result() fmt.Println(res) }
Through the above code, we successfully added the data "data1" and "data2" to the ordered collection "monitor" and query the latest data.
Next, we can create a scheduled task to write data to Redis at regular intervals. For example, we can create a Goroutine named "collector" to simulate the process of data collection and writing. We can use the following code to achieve this:
package main import ( "fmt" "time" "github.com/go-redis/redis" ) func collector(client *redis.Client) { for { // 模拟数据采集和写入 data := fmt.Sprintf("data-%d", time.Now().Unix()) client.ZAdd("monitor", &redis.Z{ Member: data, Score: float64(time.Now().Unix()), }) time.Sleep(time.Second * 1) // 每秒采集一次数据 } } func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 这里根据实际情况填写密码 DB: 0, // 默认数据库 }) go collector(client) // 查询最新的数据 for { res, _ := client.ZRevRangeByScore("monitor", &redis.ZRangeBy{ Min: "-inf", Max: "+inf", Offset: 0, Count: 1, }).Result() fmt.Println(res) time.Sleep(time.Second * 1) } }
Through the above code, we will use the "collector" Goroutine to simulate the collection and writing of data, and write a new piece of data to Redis every second. At the same time, we use a loop to query the latest data.
Through the above code examples, we successfully built a real-time monitoring system using Redis and Golang. Through Redis's ordered set data structure and Golang's high concurrency features, we can quickly store and process large amounts of real-time data.
However, this is just a simple example of a real-time monitoring system. In actual projects, we also need to consider aspects such as data persistence and visual display. However, through the powerful combination of Redis and Golang, we are better able to handle large amounts of real-time data, providing us with accurate and real-time monitoring information.
I hope this article can provide you with some ideas and references for building a real-time monitoring system. I wish you success in your practice!
The above is the detailed content of Building a real-time monitoring system using Redis and Golang: How to process large amounts of data quickly. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


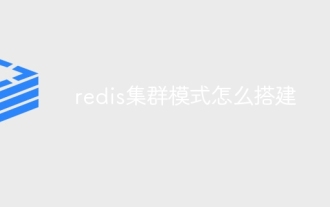
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
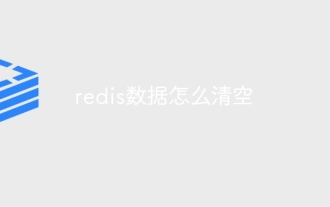
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
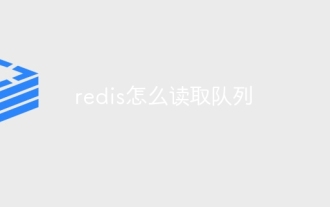
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
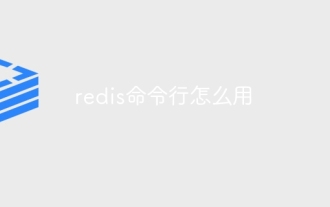
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
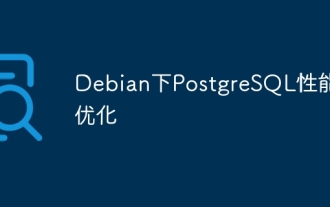
To improve the performance of PostgreSQL database in Debian systems, it is necessary to comprehensively consider hardware, configuration, indexing, query and other aspects. The following strategies can effectively optimize database performance: 1. Hardware resource optimization memory expansion: Adequate memory is crucial to cache data and indexes. High-speed storage: Using SSD SSD drives can significantly improve I/O performance. Multi-core processor: Make full use of multi-core processors to implement parallel query processing. 2. Database parameter tuning shared_buffers: According to the system memory size setting, it is recommended to set it to 25%-40% of system memory. work_mem: Controls the memory of sorting and hashing operations, usually set to 64MB to 256M
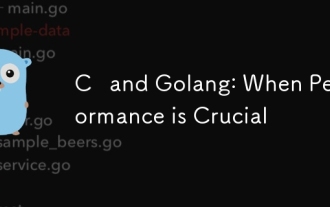
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
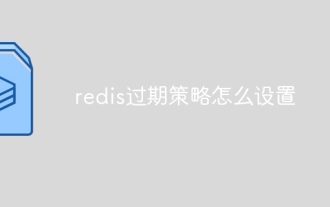
There are two types of Redis data expiration strategies: periodic deletion: periodic scan to delete the expired key, which can be set through expired-time-cap-remove-count and expired-time-cap-remove-delay parameters. Lazy Deletion: Check for deletion expired keys only when keys are read or written. They can be set through lazyfree-lazy-eviction, lazyfree-lazy-expire, lazyfree-lazy-user-del parameters.

Use of zset in Redis cluster: zset is an ordered collection that associates elements with scores. Sharding strategy: a. Hash sharding: Distribute the hash value according to the zset key. b. Range sharding: divide into ranges according to element scores, and assign each range to different nodes. Read and write operations: a. Read operations: If the zset key belongs to the shard of the current node, it will be processed locally; otherwise, it will be routed to the corresponding shard. b. Write operation: Always routed to shards holding the zset key.
