What's new in Java 12: How to use the new String API for string comparison
Java is a powerful and widely used programming language, and its versions are constantly updated to provide better functionality and performance. Java 12 is one of the latest versions of Java, which introduces many interesting new features. One of the important new features is the new String API, which provides a more concise and easy-to-use way to handle string comparisons. This article will explain how to use the new String API in Java 12 for string comparison.
In previous Java versions, we usually used the equals() method to compare whether two strings are equal. However, this approach is sometimes clumsy and error-prone. The new String API was introduced in Java 12, which contains some convenient methods for string comparison.
First, let's look at a simple example that shows how to use the new String API to compare two strings for equality:
String str1 = "Hello"; String str2 = "hello"; if (str1.equalsIgnoreCase(str2)) { System.out.println("两个字符串相等"); } else { System.out.println("两个字符串不相等"); }
In this example, we use equalsIgnoreCase() Method to compare two strings for equality. This method ignores the case of the string, so in this example, even though "Hello" and "hello" have different case, the final returned results are equal. This greatly simplifies the string comparison process.
In addition to the equalsIgnoreCase() method, the String API in Java 12 also provides a more intuitive way to compare strings, which is to use the new compareToIgnoreCase() method. This method compares two strings based on their alphabetical order and ignores case. Here is an example:
String str1 = "apple"; String str2 = "banana"; int result = str1.compareToIgnoreCase(str2); if (result < 0) { System.out.println(str1 + " 在 " + str2 + " 之前"); } else if (result > 0) { System.out.println(str1 + " 在 " + str2 + " 之后"); } else { System.out.println(str1 + " 和 " + str2 + " 相等"); }
In this example, we compare "apple" and "banana" using the compareToIgnoreCase() method. If the returned result is less than 0, it means str1 is before str2; if the returned result is greater than 0, it means str1 is after str2; if the returned result is equal to 0, it means str1 and str2 are equal.
Another useful new feature is the startsWith() and endsWith() methods. These two methods are used to check whether a string starts or ends with a specified substring. Here is an example:
String str = "Hello, world!"; if (str.startsWith("Hello")) { System.out.println("字符串以 'Hello' 开头"); } if (str.endsWith("world!")) { System.out.println("字符串以 'world!' 结尾"); }
In this example, we use the startsWith() and endsWith() methods to check whether a string starts or ends with a specified substring. If the return value is true, it means that the condition is met; if the return value is false, it means that the condition is not met.
In addition to the methods mentioned above, the String API in Java 12 also provides many other practical methods to handle strings. For example, the strip() method is used to remove spaces at both ends of a string; the stripLeading() method is used to remove spaces at the beginning of a string; and the stripTrailing() method is used to remove spaces at the end of a string. In addition, Java 12 also introduces the indent() method, transform() method, etc. These methods can greatly simplify string processing.
To sum up, the new String API in Java 12 provides us with a more concise and easy-to-use way to handle string comparisons. Whether it's ignoring case comparisons, comparing the alphabetical order of strings, or checking whether a string starts or ends with a certain substring, these new methods make our code clearer and more readable. By using these new features, we can handle string comparisons more efficiently, reduce errors, and improve code maintainability.
I hope the content of this article can help you understand the new String API in Java 12 and how to use it for string comparison. By taking full advantage of the new String API, we can write cleaner, more readable code and improve development efficiency.
The above is the detailed content of What's new in Java 12: How to use the new String API for string comparison. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
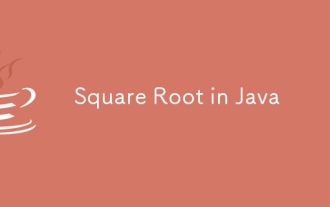
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
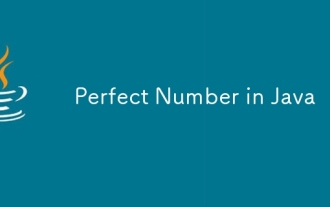
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
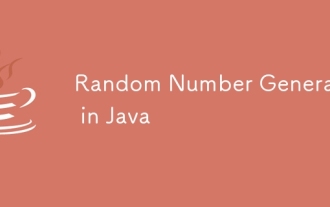
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
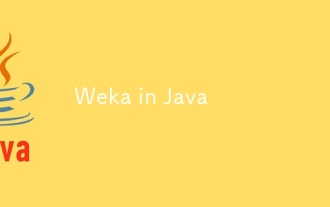
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
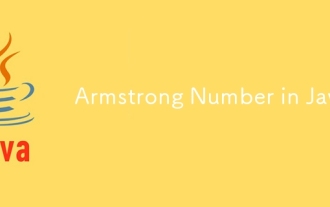
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
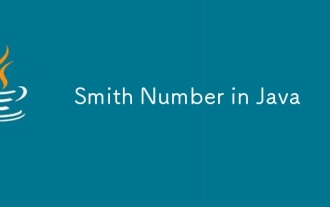
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
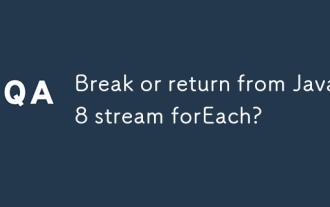
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
