


Quick Start: Implementing Simple Machine Learning Algorithms Using Go Language Functions
Quick Start: Using Go language functions to implement simple machine learning algorithms
In today's information age, machine learning has become a popular technical field. Many programming languages provide rich machine learning libraries and frameworks, and the Go language is no exception. This article will take you quickly to understand how to use functions in the Go language to implement simple machine learning algorithms, and illustrate it with a code example.
First, we need to understand a few basic concepts. Machine learning is a technique that trains a model to learn from data and make predictions. Among them, the model is composed of many functions that map inputs to outputs. The process of training the model is to determine the parameters of the function through training data so that the function can best fit the data and make accurate predictions.
There are many advantages to using functions to implement machine learning algorithms in the Go language. First of all, Go language is an efficient and statically typed programming language with strong concurrency performance, suitable for processing large amounts of data. Secondly, functions, as a way of code reuse, can make our code more concise, structured and easier to maintain.
Code Example: Linear Regression Algorithm
Below, we will use a simple linear regression algorithm code example to demonstrate how to use Go language functions to implement machine learning algorithms.
First, we need to import the required package:
package main import ( "fmt" "math" )
Then, we define a function to calculate the predicted value of the model:
func predict(x float64, w float64, b float64) float64 { return (w * x) + b }
Next, we define a function to calculate The value of the loss function to measure how well the model fits the training data:
func loss(x []float64, y []float64, w float64, b float64) float64 { m := float64(len(x)) var totalLoss float64 for i := 0; i < len(x); i++ { prediction := predict(x[i], w, b) totalLoss += math.Pow((prediction - y[i]), 2) } return totalLoss / (2 * m) }
Next, we define a function to train the model, that is, find the parameter value that minimizes the loss function:
func train(x []float64, y []float64, learningRate float64, epochs int) (float64, float64) { var w, b float64 for epoch := 0; epoch < epochs; epoch++ { var gradientW, gradientB float64 for i := 0; i < len(x); i++ { prediction := predict(x[i], w, b) gradientW += ((prediction - y[i]) * x[i]) gradientB += (prediction - y[i]) } m := float64(len(x)) gradientW /= m gradientB /= m w -= learningRate * gradientW b -= learningRate * gradientB } return w, b }
Finally, we can use the functions defined above for model training and prediction:
func main() { x := []float64{1, 2, 3, 4, 5} y := []float64{2, 4, 6, 8, 10} learningRate := 0.01 epochs := 1000 w, b := train(x, y, learningRate, epochs) fmt.Printf("训练完成,得到的模型参数为:w=%.2f, b=%.2f ", w, b) newX := 6 prediction := predict(float64(newX), w, b) fmt.Printf("当 x=%d 时,预测值为:%.2f ", newX, prediction) }
By running the above code, we can get the following output:
训练完成,得到的模型参数为:w=2.00, b=0.00 当 x=6 时,预测值为:12.00
Through the above code example, we not only I learned how to use functions in the Go language to implement simple machine learning algorithms, and also learned the basic principles and implementation process of the linear regression algorithm.
Summary:
This article demonstrates how to use Go language functions to implement simple machine learning algorithms through a code example. Although this is just a simple example, by learning and mastering this process, we can gain a deeper understanding of the principles of machine learning and more complex algorithms. I hope this article is helpful to you, and also encourages you to continue to explore and apply machine learning algorithms, and continue to enrich and develop your technical capabilities.
The above is the detailed content of Quick Start: Implementing Simple Machine Learning Algorithms Using Go Language Functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


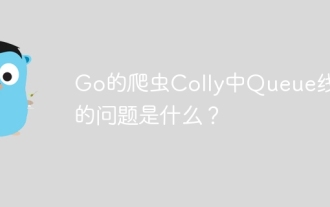
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
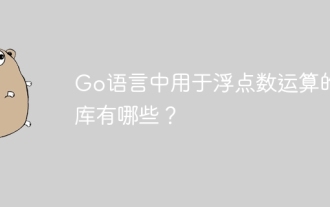
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
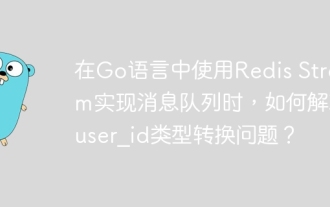
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
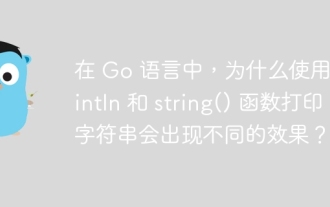
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
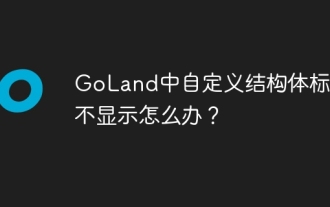
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
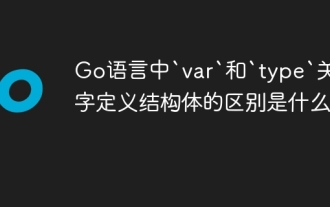
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
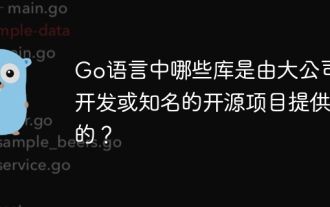
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
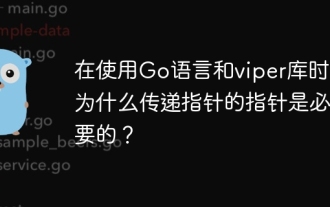
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
