How to implement cache preloading function using Redis and JavaScript
How to use Redis and JavaScript to implement cache preloading function
In modern web applications, caching is one of the important means to improve performance and reduce server response time. Cache preloading actively loads data into the cache before user requests to reduce user waiting time and reduce the load on the server. This article will introduce how to use Redis and JavaScript to implement cache preloading function.
- Introduction to Redis
Redis is a high-performance key-value storage database that supports a variety of data structures and functions. Among them, one of the most commonly used data structures is cache (Cache), which can store data in memory to speed up data reading and access. - JavaScript implements cache preloading
In front-end development, JavaScript is a very commonly used language. We can call Redis related operations through JavaScript code to achieve the cache preloading function.
First of all, we need to introduce the Redis JavaScript client library on the front end, such as ioredis. Install the ioredis library through npm and introduce it into the project.
$npm install ioredis
import Redis from 'ioredis'; const redis = new Redis({ host: 'localhost', port: 6379, password: 'your_password', }); redis.on('ready', () => { console.log('Redis connection ready'); }); redis.on('error', (err) => { console.error('Redis connection error', err); }); // 示例代码 function preloadCache(key, value) { // 将数据存储到缓存中 redis.set(key, value).catch((err) => { console.error(`Failed to cache data for key ${key}`, err); }); } // 定义需要预加载的数据 const dataToPreload = [ { key: 'user:1', value: JSON.stringify({ id: 1, name: '张三' }) }, { key: 'user:2', value: JSON.stringify({ id: 2, name: '李四' }) }, // 更多的数据... ]; // 预加载数据 dataToPreload.forEach((data) => { preloadCache(data.key, data.value); });
In the above code, we create a connection with the Redis database through the ioredis library, and print a successful connection message in the redis.on('ready')
callback function . Next, we store the data in the Redis cache by defining the preloadCache
function. Finally, by traversing the dataToPreload
array, we can implement the function of preloading data into the cache.
It should be noted that this is just a simple sample code, you can define and process cache data according to your actual needs.
- Advantages and application scenarios of cache preloading
The advantage of cache preloading is to load data into the cache in advance, reducing the waiting time for the user's first visit and improving the user experience. At the same time, since data already exists in the cache, subsequent requests can be read directly from the cache, reducing access to the database and reducing the burden on the server.
Cache preloading is suitable for application scenarios where a large amount of data needs to be loaded in the early stage, such as product information on e-commerce websites, article lists on news websites, etc. By preloading this data into the cache, you can improve the response speed when users access these pages and reduce the loading time.
- Summary
This article introduces how to use Redis and JavaScript to implement the cache preloading function. Through the ioredis library, we can establish a connection with the Redis database and store the data into the cache through JavaScript code. Cache preloading can improve application performance and user experience, and is suitable for application scenarios that require loading large amounts of data in advance. I hope this article will help you understand cache preloading, and I hope you can flexibly use this technology in actual development.
The above is the detailed content of How to implement cache preloading function using Redis and JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
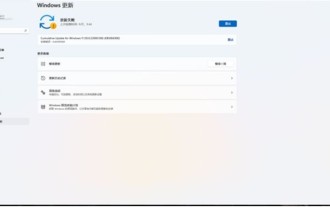
1. Start the [Start] menu, enter [cmd], right-click [Command Prompt], and select Run as [Administrator]. 2. Enter the following commands in sequence (copy and paste carefully): SCconfigwuauservstart=auto, press Enter SCconfigbitsstart=auto, press Enter SCconfigcryptsvcstart=auto, press Enter SCconfigtrustedinstallerstart=auto, press Enter SCconfigwuauservtype=share, press Enter netstopwuauserv , press enter netstopcryptS
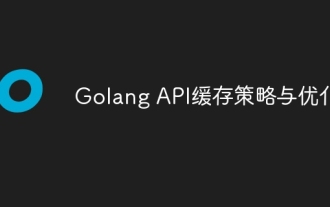
The caching strategy in GolangAPI can improve performance and reduce server load. Commonly used strategies are: LRU, LFU, FIFO and TTL. Optimization techniques include selecting appropriate cache storage, hierarchical caching, invalidation management, and monitoring and tuning. In the practical case, the LRU cache is used to optimize the API for obtaining user information from the database. The data can be quickly retrieved from the cache. Otherwise, the cache can be updated after obtaining it from the database.
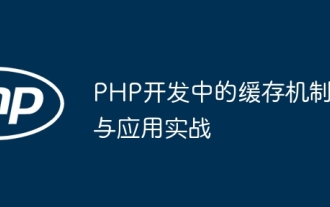
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.
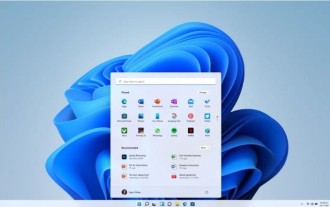
First you need to set the system language to Simplified Chinese display and restart. Of course, if you have changed the display language to Simplified Chinese before, you can just skip this step. Next, start operating the registry, regedit.exe, directly navigate to HKEY_LOCAL_MACHINESYSTEMCurrentControlSetControlNlsLanguage in the left navigation bar or the upper address bar, and then modify the InstallLanguage key value and Default key value to 0804 (if you want to change it to English en-us, you need First set the system display language to en-us, restart the system and then change everything to 0409) You must restart the system at this point.
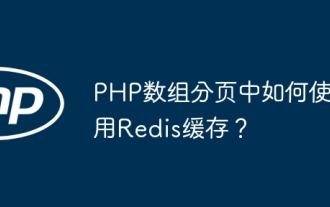
Using Redis cache can greatly optimize the performance of PHP array paging. This can be achieved through the following steps: Install the Redis client. Connect to the Redis server. Create cache data and store each page of data into a Redis hash with the key "page:{page_number}". Get data from cache and avoid expensive operations on large arrays.
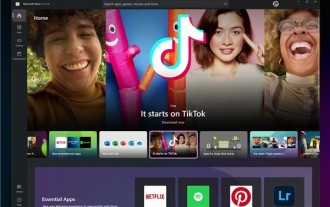
1. First, double-click the [This PC] icon on the desktop to open it. 2. Then double-click the left mouse button to enter [C drive]. System files will generally be automatically stored in C drive. 3. Then find the [windows] folder in the C drive and double-click to enter. 4. After entering the [windows] folder, find the [SoftwareDistribution] folder. 5. After entering, find the [download] folder, which contains all win11 download and update files. 6. If we want to delete these files, just delete them directly in this folder.
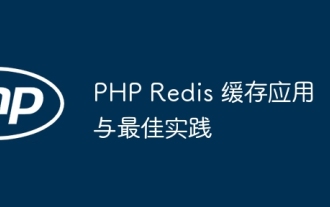
Redis is a high-performance key-value cache. The PHPRedis extension provides an API to interact with the Redis server. Use the following steps to connect to Redis, store and retrieve data: Connect: Use the Redis classes to connect to the server. Storage: Use the set method to set key-value pairs. Retrieval: Use the get method to obtain the value of the key.
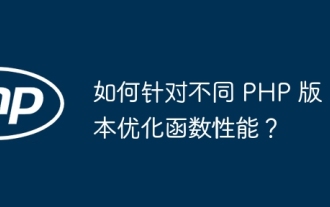
Methods to optimize function performance for different PHP versions include: using analysis tools to identify function bottlenecks; enabling opcode caching or using an external caching system; adding type annotations to improve performance; and selecting appropriate string concatenation and sorting algorithms according to the PHP version.
