


CakePHP middleware: implement file upload and download functions
CakePHP middleware: Implementing file upload and download functions
With the development of the Internet, file upload and download functions are becoming more and more common. When developing web applications, we often need to implement file upload and download. When developing applications using the CakePHP framework, middleware is a very useful tool that can help us simplify the code and implement file upload and download functions. Next, I will introduce how to use CakePHP middleware to implement file upload and download functions.
First, we need to create a new middleware class, create a file named FileHandlerMiddleware.php
in the src/Middleware
directory, and add the following code :
<?php namespace AppMiddleware; use CakeUtilityText; use CakeHttpResponse; use PsrHttpMessageResponseInterface; use PsrHttpMessageServerRequestInterface; use CakeHttpServerRequest; class FileHandlerMiddleware { public function __invoke(ServerRequestInterface $request, ResponseInterface $response, $next) { $path = WWW_ROOT . 'uploads' . DS; // 处理文件上传 if ($request->getMethod() === 'POST' && $request->getData('file')) { $file = $request->getData('file'); $fileName = Text::uuid() . '-' . $file->getClientFilename(); $file->moveTo($path . $fileName); $response = new Response(); $response = $response->withAddedHeader('Content-Type', 'application/json'); $response->getBody()->write(json_encode(['success' => true, 'message' => '文件上传成功!'])); return $response; } // 处理文件下载 $params = $request->getAttribute('params'); if (isset($params['file'])) { $fileName = $params['file']; $filePath = $path . $fileName; if (file_exists($filePath)) { $stream = fopen($filePath, 'r'); $response = new Response(); $response = $response->withAddedHeader('Content-Disposition', 'attachment; filename="' . $fileName . '"'); $response->withBody(new SlimHttpStream($stream)); return $response; } } return $next($request, $response); } }
In the above code, FileHandlerMiddleware
is a middleware class for file upload and download. When a POST
request is received and the request contains data named file
, the middleware will save the file to the uploads
folder and return a success JSON response. When a request with the file
parameter is received, the middleware will return the file content according to the file name as a response.
Next, we need to register the middleware into the application. Open the src/Application.php
file and register the middleware in the middleware
method of the Application
class. The code is as follows:
use AppMiddlewareFileHandlerMiddleware; // ... public function middleware($middlewareQueue) { $middlewareQueue ->add(new FileHandlerMiddleware()) // 其它中间件 // ... ->add(new ErrorHandlerMiddleware(Configure::read('Error'))) ->add(new AssetMiddleware()) ->add(new RoutingMiddleware($this)); return $middlewareQueue; }
In the above code, we use the add
method to register the FileHandlerMiddleware
middleware into the middleware queue. Use a middleware queue to process multiple middlewares in sequence and execute registered middlewares before executing controller actions.
Now, we can use the file upload and download functions. Suppose we have a controller method to handle file upload:
public function upload() { // 显示上传表单 }
Then, add the following form code to the corresponding view file:
<form method="POST" action="/upload" enctype="multipart/form-data"> <input type="file" name="file" /> <button type="submit">上传</button> </form>
In the above code, we create a form , which contains a file upload field and a submit button. The form's enctype
attribute is set to multipart/form-data
, which is required for file uploads.
When the user selects a file and clicks the submit button, the file will be uploaded to the server and a successful JSON response will be returned.
In addition, we can also use the following URL to download files:
/download/{file_name}
For example, to download a file named example.jpg
, you can use the following URL:
/download/example.jpg
The file will be returned to the user in downloaded format.
Summary:
This article introduces how to use CakePHP middleware to implement file upload and download functions. By creating a new middleware class, we can handle file upload and download requests and return appropriate responses. Middleware helps us simplify the code and enhance the functionality of the application. I hope this article will help you understand and use CakePHP middleware.
The above is the detailed content of CakePHP middleware: implement file upload and download functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


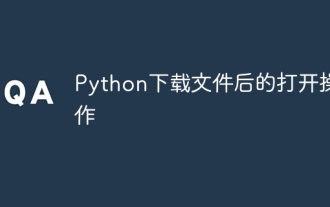
Python provides the following options to open downloaded files: open() function: open the file using the specified path and mode (such as 'r', 'w', 'a'). Requests library: Use its download() method to automatically assign a name and open the file directly. Pathlib library: Use write_bytes() and read_text() methods to write and read file contents.
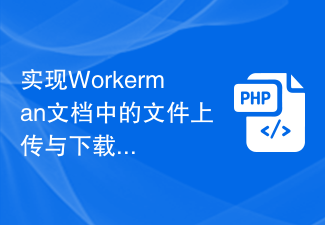
To implement file upload and download in Workerman documents, specific code examples are required. Introduction: Workerman is a high-performance PHP asynchronous network communication framework that is simple, efficient, and easy to use. In actual development, file uploading and downloading are common functional requirements. This article will introduce how to use the Workerman framework to implement file uploading and downloading, and give specific code examples. 1. File upload: File upload refers to the operation of transferring files on the local computer to the server. The following is used
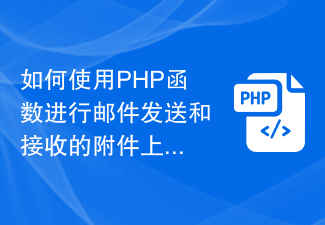
How to use PHP functions to upload and download attachments for sending and receiving emails? With the rapid development of modern communication technology, email has become an important way for people to communicate and transmit information in daily life. In web development, we often encounter the need to send and receive emails with attachments. As a powerful server-side scripting language, PHP provides a wealth of functions and class libraries that can simplify the email processing process. This article will introduce how to use PHP functions to upload and download attachments for sending and receiving emails. Email is sent first, we
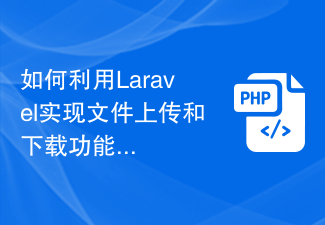
How to use Laravel to implement file upload and download functions Laravel is a popular PHP Web framework that provides a wealth of functions and tools to make developing Web applications easier and more efficient. One of the commonly used functions is file upload and download. This article will introduce how to use Laravel to implement file upload and download functions, and provide specific code examples. File upload File upload refers to uploading local files to the server for storage. In Laravel we can use file upload
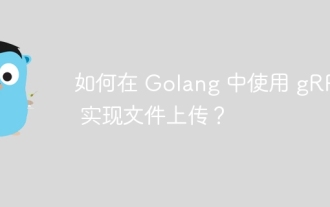
How to implement file upload using gRPC? Create supporting service definitions, including request and response messages. On the client, the file to be uploaded is opened and split into chunks, then streamed to the server via a gRPC stream. On the server side, file chunks are received and stored into a file. The server sends a response after the file upload is completed to indicate whether the upload was successful.
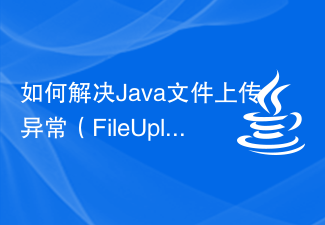
How to solve Java file upload exception (FileUploadException). One problem that is often encountered in web development is FileUploadException (file upload exception). It may occur due to various reasons such as file size exceeding limit, file format mismatch, or incorrect server configuration. This article describes some ways to solve these problems and provides corresponding code examples. Limit the size of uploaded files In most scenarios, limit the file size
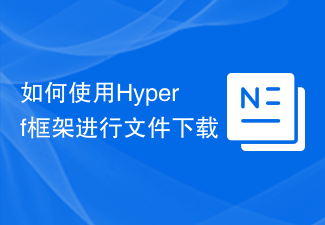
How to use the Hyperf framework for file downloading Introduction: File downloading is a common requirement when developing web applications using the Hyperf framework. This article will introduce how to use the Hyperf framework for file downloading, including specific code examples. 1. Preparation Before starting, make sure you have installed the Hyperf framework and successfully created a Hyperf application. 2. Create a file download controller First, we need to create a controller to handle file download requests. Open the terminal and enter
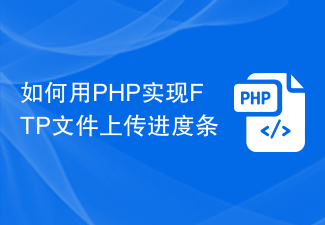
How to use PHP to implement FTP file upload progress bar 1. Background introduction In website development, file upload is a common function. For the upload of large files, in order to improve the user experience, we often need to display an upload progress bar to the user to let the user know the file upload process. This article will introduce how to use PHP to implement the FTP file upload progress bar function. 2. The basic idea of implementing the progress bar of FTP file upload. The progress bar of FTP file upload is usually calculated by calculating the size of the uploaded file and the size of the uploaded file.
