


How to use the thread module to create and manage threads in Python 2.x
How to use the thread module to create and manage threads in Python 2.x
Introduction:
In multi-threaded programming, we often need to create and manage multiple threads to implement concurrently executed tasks. Python provides the thread module to support multi-threaded programming. This article will introduce how to use the thread module to create and manage threads, and provide some code examples.
- Thread module overview:
The thread module provides some thread-related functions and classes for creating and managing threads. The following is a brief introduction to commonly used thread module functions and classes:
- thread.start_new_thread(function, args[, kwargs]): Create a new thread and execute the function function, args and kwargs are parameters passed to functions.
- thread.allocate_lock(): Create a new lock object for synchronization between threads.
- thread.exit(): The thread exits and ends the execution of the thread.
- thread.get_ident(): Get the identifier of the current thread.
- thread.interrupt_main(): Interrupt the execution of the main thread.
- thread.stack_size([size]): Get or set the thread stack size.
- Example of creating a thread:
The following example demonstrates how to use the thread module to create a thread.
import thread import time # 定义线程执行的函数 def print_time(threadName, delay): count = 0 while count < 5: time.sleep(delay) count += 1 print("%s: %s" % (threadName, time.ctime(time.time()))) # 创建两个线程 try: thread.start_new_thread(print_time, ("Thread-1", 2,)) thread.start_new_thread(print_time, ("Thread-2", 4,)) except: print("Error: 无法启动线程") # 主线程等待子线程结束 while 1: pass
Running the above code will create two threads that print the current time every 2 seconds and 4 seconds respectively. The main thread will wait for the child thread to end.
- Thread synchronization and locking:
In thread programming, it is often necessary to ensure the correct cooperation between multiple threads to avoid competition and conflicts. The thread module provides lock objects to achieve synchronization between threads. The following example shows how to use locks to guarantee mutually exclusive execution of threads.
import thread import time # 全局变量 counter = 0 lock = thread.allocate_lock() # 线程函数 def increment_counter(threadName, delay): global counter while True: lock.acquire() counter += 1 print("%s: %d" % (threadName, counter)) lock.release() time.sleep(delay) # 创建两个线程 try: thread.start_new_thread(increment_counter, ("Thread-1", 1,)) thread.start_new_thread(increment_counter, ("Thread-2", 2,)) except: print("Error: 无法启动线程") # 主线程等待子线程结束 while 1: pass
The above code creates two threads, increments the counter variable at different speeds and prints the results. Through the use of locks, mutually exclusive access to counter between threads is ensured, and race conditions are avoided.
Conclusion:
This article introduces the basic method of using the thread module to create and manage threads in Python 2.x, and provides some code examples. It is important to understand and master multi-threaded programming to improve the performance and responsiveness of your application. In actual development, you can also use more advanced and flexible multi-threading libraries, such as the threading module, which provides more functions and easier-to-use interfaces, but the basic principles and ideas are similar.
Reference materials:
- Python thread module documentation: https://docs.python.org/2/library/thread.html
The above is the detailed content of How to use the thread module to create and manage threads in Python 2.x. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


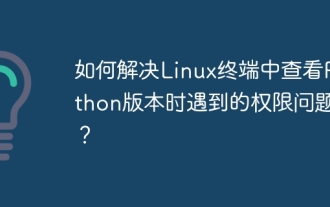
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
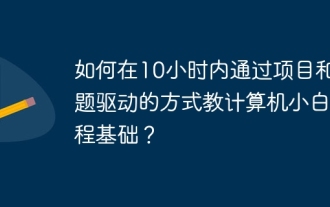
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
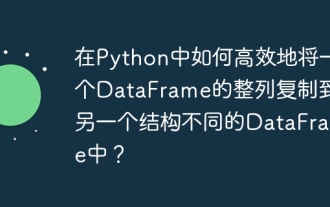
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
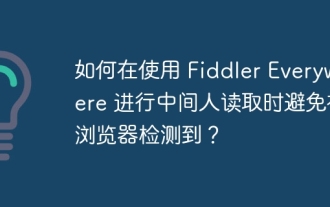
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
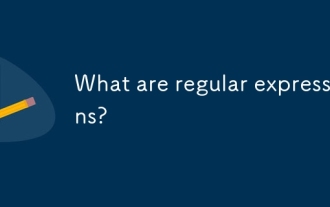
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
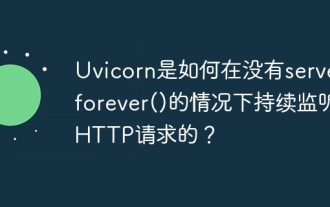
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
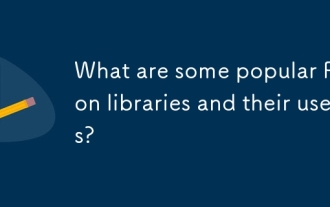
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
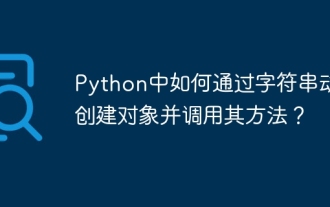
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
