PHP and PDO: How to perform a full-text search in a database
PHP and PDO: How to perform a full-text search in a database
In modern web applications, the database is a very important component. Full-text search is a very useful feature when we need to search for specific information from large amounts of data. PHP and PDO (PHP Data Objects) provide a simple yet powerful way to perform full-text searches in databases. This article will introduce how to use PHP and PDO to implement full-text search, and provide some sample code to demonstrate the process.
First, we need to ensure that the database connection has been configured correctly before using PDO. This can be done by passing the correct database driver, hostname, database name, username and password in the PDO constructor.
Next, in our application, we need to create a full-text search query. To achieve this, we can use the MATCH AGAINST statement. The MATCH AGAINST statement is used to search a database table for rows that match a specified search expression. Here is a sample code that shows how to perform a full-text search query in a database:
$searchTerm = "apple"; // 创建并执行全文搜索查询 $query = "SELECT * FROM products WHERE MATCH(product_name) AGAINST(:searchTerm IN BOOLEAN MODE)"; $stmt = $pdo->prepare($query); $stmt->bindParam(':searchTerm', $searchTerm, PDO::PARAM_STR); $stmt->execute(); $results = $stmt->fetchAll(PDO::FETCH_ASSOC); // 输出搜索结果 foreach ($results as $result) { echo $result['product_name'] . "<br>"; }
In the above code, we first define a search term variable $searchTerm
, which contains our The keyword "apple" to search for. We then created a SQL query and bound the search term variable using bindParam
. In the query, we use MATCH(product_name)
to specify the columns to search and the AGAINST
clause to specify the search expression. Finally, we execute the query and output the search results by looping through the results array.
In the above example, IN BOOLEAN MODE
means that we will use Boolean search mode to perform the search. This means that we can use the
symbols in search expressions to indicate that certain keywords must be included, the -
symbols to indicate that certain keywords must be excluded, and the *
Wildcard to match any character. This makes full-text search more flexible and powerful.
In addition to full-text search, we can also use PDO to perform other types of database queries, such as inserts, updates, and deletes. Here is some sample code showing how to perform these operations:
// 插入数据 $query = "INSERT INTO products (product_name, price) VALUES (:productName, :price)"; $stmt = $pdo->prepare($query); $stmt->bindParam(':productName', $productName, PDO::PARAM_STR); $stmt->bindParam(':price', $price, PDO::PARAM_INT); $stmt->execute(); // 更新数据 $query = "UPDATE products SET price = :newPrice WHERE product_name = :productName"; $stmt = $pdo->prepare($query); $stmt->bindParam(':newPrice', $newPrice, PDO::PARAM_INT); $stmt->bindParam(':productName', $productName, PDO::PARAM_STR); $stmt->execute(); // 删除数据 $query = "DELETE FROM products WHERE product_name = :productName"; $stmt = $pdo->prepare($query); $stmt->bindParam(':productName', $productName, PDO::PARAM_STR); $stmt->execute();
Through these sample codes, we can see that PDO provides a simple and powerful way to perform full-text searches in databases as well as other types of Query operations. Using PDO, we can easily interact with a variety of different databases without having to switch between different database operation libraries.
In this article, we explored how to use PHP and PDO to perform a full-text search in a database. We learned how to create a full-text search query and showed some sample code to illustrate the process. Hopefully these examples will help readers better understand and apply the concepts and techniques of full-text search.
The above is the detailed content of PHP and PDO: How to perform a full-text search in a database. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


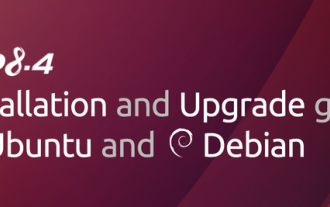
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
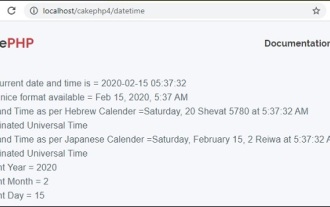
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
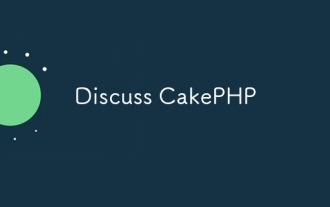
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
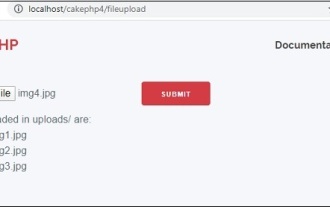
To work on file upload we are going to use the form helper. Here, is an example for file upload.
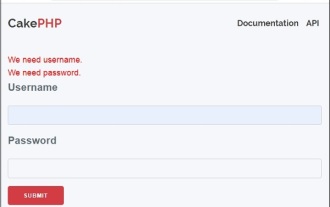
Validator can be created by adding the following two lines in the controller.
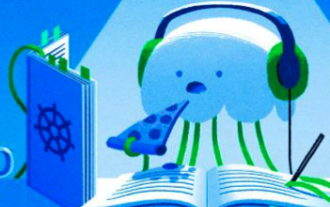
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
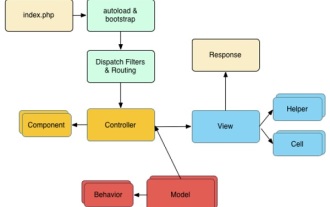
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
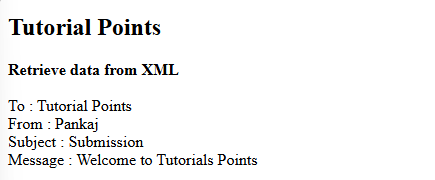
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
