


PHP 5.5 function analysis: How to use the array_reduce function to combine array elements into one value
PHP 5.5 Function Analysis: How to use the array_reduce function to combine array elements into one value
In PHP programming, we often need to process arrays, and sometimes we need to combine array elements into one value. At this time, we can use the array_reduce function introduced in PHP 5.5 version to implement this function. This article will introduce the use of array_reduce function in detail and provide corresponding code examples.
The array_reduce function is a very practical PHP function that accepts an array and a callback function as parameters. The callback function accepts two parameters, one is the result value in the last iteration, and the other is the value of the current array element. The callback function will calculate the result of the current iteration based on these two parameters, return the result, and then pass it to the callback function as the first parameter of the next iteration.
Let us illustrate the use of array_reduce function through a simple example. Suppose we have an array of integers and we want to add all the elements in the array and return the added result. The following is a code example that uses the array_reduce function to implement this function:
$arr = [1, 2, 3, 4, 5]; $result = array_reduce($arr, function($carry, $item) { return $carry + $item; }, 0); echo $result; // 输出15
In the above code, we first define an integer array $arr, which contains some integer values. Then, we call the array_reduce function and pass it $arr as the first argument. For the callback function, we use an anonymous function, where $carry represents the result value of the last iteration and $item represents the value of the current array element. In each iteration, we add $carry and $item and return the result. The initial value is 0.
Finally, we use the echo statement to output $result, which is the result of adding all elements of the array.
In addition to the function of adding array elements, we can also use the array_reduce function to implement other types of merge operations. For example, we can concatenate all the elements in the string array to generate a long string as shown below:
$arr = ['Hello', ' ', 'PHP']; $result = array_reduce($arr, function($carry, $item) { return $carry . $item; }, ''); echo $result; // 输出Hello PHP
In the above example, we defined a string array $arr, which contains some string elements. Then, we call the array_reduce function and pass it $arr as the first argument. For the callback function, we also use an anonymous function, where $carry represents the result value of the last iteration and $item represents the value of the current array element. In each iteration, we concatenate $carry with $item and return the result. The initial value is the empty string ''.
Finally, we use the echo statement to output $result, which is the result of concatenating all string elements.
Through the above two examples, we can see the powerful function of array_reduce function. It can not only add array elements, but also concatenate array elements, and even achieve more complex merge operations. As long as we define the appropriate callback function, the array_reduce function can help us complete the merge operation.
Summary: The array_reduce function is a very practical function introduced in PHP version 5.5. It accepts an array and a callback function as parameters, which can combine the array elements into a single value. According to the callback function we define, we can implement various types of merge operations. By mastering the use of the array_reduce function, we can handle array operations more efficiently and improve our programming efficiency.
The above is the detailed content of PHP 5.5 function analysis: How to use the array_reduce function to combine array elements into one value. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


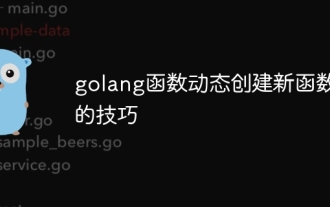
Go language provides two dynamic function creation technologies: closure and reflection. closures allow access to variables within the closure scope, and reflection can create new functions using the FuncOf function. These technologies are useful in customizing HTTP routers, implementing highly customizable systems, and building pluggable components.
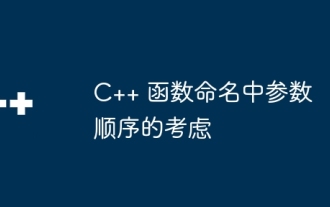
In C++ function naming, it is crucial to consider parameter order to improve readability, reduce errors, and facilitate refactoring. Common parameter order conventions include: action-object, object-action, semantic meaning, and standard library compliance. The optimal order depends on the purpose of the function, parameter types, potential confusion, and language conventions.
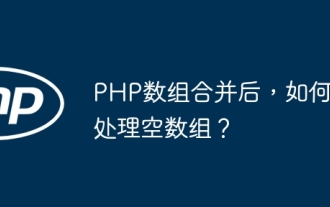
When using array_merge() in PHP to merge arrays, it will produce confusing results if it contains empty strings or empty arrays. Solution: 1. Use array_filter() to filter null values. 2. For cases containing empty arrays, use the recursive merge function array_merge_recursive_distinct() to maintain a consistent array structure.
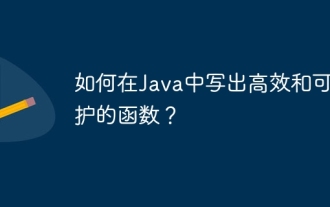
The key to writing efficient and maintainable Java functions is: keep it simple. Use meaningful naming. Handle special situations. Use appropriate visibility.
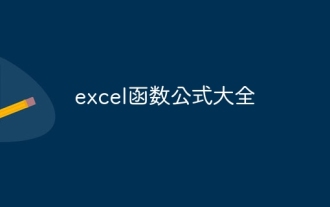
1. The SUM function is used to sum the numbers in a column or a group of cells, for example: =SUM(A1:J10). 2. The AVERAGE function is used to calculate the average of the numbers in a column or a group of cells, for example: =AVERAGE(A1:A10). 3. COUNT function, used to count the number of numbers or text in a column or a group of cells, for example: =COUNT(A1:A10) 4. IF function, used to make logical judgments based on specified conditions and return the corresponding result.
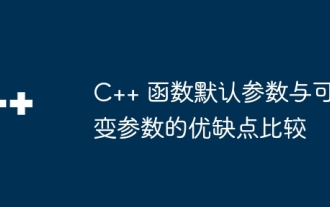
The advantages of default parameters in C++ functions include simplifying calls, enhancing readability, and avoiding errors. The disadvantages are limited flexibility and naming restrictions. Advantages of variadic parameters include unlimited flexibility and dynamic binding. Disadvantages include greater complexity, implicit type conversions, and difficulty in debugging.
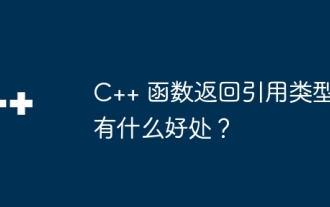
The benefits of functions returning reference types in C++ include: Performance improvements: Passing by reference avoids object copying, thus saving memory and time. Direct modification: The caller can directly modify the returned reference object without reassigning it. Code simplicity: Passing by reference simplifies the code and requires no additional assignment operations.
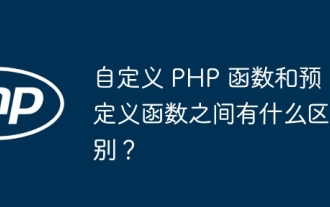
The difference between custom PHP functions and predefined functions is: Scope: Custom functions are limited to the scope of their definition, while predefined functions are accessible throughout the script. How to define: Custom functions are defined using the function keyword, while predefined functions are defined by the PHP kernel. Parameter passing: Custom functions receive parameters, while predefined functions may not require parameters. Extensibility: Custom functions can be created as needed, while predefined functions are built-in and cannot be modified.
