


Learn the file operation functions in Go language and implement file compression, encryption, upload, download and decompression functions
Learn the file operation functions in Go language and implement file compression, encryption, upload, download and decompression functions
In modern computer applications, file operation is a very important function. For developers, learning and mastering file operation functions can not only improve development efficiency, but also add some practical functions to applications. This article will introduce how to use the file operation functions in the Go language and implement file compression, encryption, upload, download and decompression functions.
First of all, we need to understand some basic functions of file operations in Go language.
- Create file
To create a new file, we can use the Create function in the os package.
file, err := os.Create("example.txt") if err != nil { log.Fatal(err) } defer file.Close()
- Write data
To write data in a file, we can use the Write method of the file object.
data := []byte("Hello, World!") _, err = file.Write(data) if err != nil { log.Fatal(err) }
- Reading data
To read data from a file, we can use the Read method of the file object.
data := make([]byte, 100) n, err := file.Read(data) if err != nil { log.Fatal(err) } fmt.Printf("Read %d bytes: %s ", n, data[:n])
- Delete files
To delete files, you can use the Remove function in the os package.
err := os.Remove("example.txt") if err != nil { log.Fatal(err) }
Now that we have mastered the basic file operation functions, we can continue to implement file compression, encryption, upload, download and decompression functions.
First, let’s see how to compress and decompress files. We can use the archive/zip package to achieve this.
func compressFile(filename string) { zipfilename := filename + ".zip" zipFile, err := os.Create(zipfilename) if err != nil { log.Fatal(err) } defer zipFile.Close() zipWriter := zip.NewWriter(zipFile) defer zipWriter.Close() file, err := os.Open(filename) if err != nil { log.Fatal(err) } defer file.Close() fileInfo, err := file.Stat() if err != nil { log.Fatal(err) } header, err := zip.FileInfoHeader(fileInfo) if err != nil { log.Fatal(err) } writer, err := zipWriter.CreateHeader(header) if err != nil { log.Fatal(err) } _, err = io.Copy(writer, file) if err != nil { log.Fatal(err) } } func decompressFile(zipfilename string) { zipFile, err := zip.OpenReader(zipfilename) if err != nil { log.Fatal(err) } defer zipFile.Close() for _, file := range zipFile.File { rc, err := file.Open() if err != nil { log.Fatal(err) } defer rc.Close() newFile, err := os.Create(file.Name) if err != nil { log.Fatal(err) } defer newFile.Close() _, err = io.Copy(newFile, rc) if err != nil { log.Fatal(err) } } }
Next, let’s take a look at how to encrypt and decrypt files. We can use the crypto/aes package to achieve this.
func encryptFile(filename string, key []byte) { inputFile, err := os.Open(filename) if err != nil { log.Fatal(err) } defer inputFile.Close() outputFile, err := os.Create(filename + ".enc") if err != nil { log.Fatal(err) } defer outputFile.Close() block, err := aes.NewCipher(key) if err != nil { log.Fatal(err) } iv := make([]byte, aes.BlockSize) outputFile.Write(iv) outputFileWriter := cipher.StreamWriter{ S: cipher.NewCTR(block, iv), W: outputFile, } _, err = io.Copy(outputFileWriter, inputFile) if err != nil { log.Fatal(err) } } func decryptFile(filename string, key []byte) { inputFile, err := os.Open(filename + ".enc") if err != nil { log.Fatal(err) } defer inputFile.Close() outputFile, err := os.Create(filename + ".dec") if err != nil { log.Fatal(err) } defer outputFile.Close() block, err := aes.NewCipher(key) if err != nil { log.Fatal(err) } iv := make([]byte, aes.BlockSize) inputFile.Read(iv) inputFileReader := cipher.StreamReader{ S: cipher.NewCTR(block, iv), R: inputFile, } _, err = io.Copy(outputFile, inputFileReader) if err != nil { log.Fatal(err) } }
Now that we have implemented the file compression, encryption and decoding functions, let us see how to upload and download files. We can use the net/http package to achieve this.
func uploadFile(filename string, url string) { file, err := os.Open(filename) if err != nil { log.Fatal(err) } defer file.Close() body := &bytes.Buffer{} writer := multipart.NewWriter(body) part, err := writer.CreateFormFile("file", filepath.Base(filename)) if err != nil { log.Fatal(err) } _, err = io.Copy(part, file) if err != nil { log.Fatal(err) } writer.Close() request, err := http.NewRequest("POST", url, body) if err != nil { log.Fatal(err) } request.Header.Add("Content-Type", writer.FormDataContentType()) client := &http.Client{} response, err := client.Do(request) if err != nil { log.Fatal(err) } defer response.Body.Close() fmt.Println("Upload OK!") } func downloadFile(url string, filename string) { response, err := http.Get(url) if err != nil { log.Fatal(err) } defer response.Body.Close() file, err := os.Create(filename) if err != nil { log.Fatal(err) } defer file.Close() _, err = io.Copy(file, response.Body) if err != nil { log.Fatal(err) } fmt.Println("Download OK!") }
Now we have implemented the file compression, encryption, upload, download and decompression functions. We can use these functions according to our own needs to handle file operations and file transfer needs. By using these functions together, we can develop more secure and efficient file operation functions.
Summary: This article introduces the file operation functions in the Go language, and provides example codes for file compression, encryption, upload, download and decompression. By learning and mastering these functions, we can add more practical and powerful file operation functions to our applications. I hope this article will be helpful to you in learning and using Go language to handle file operations.
The above is the detailed content of Learn the file operation functions in Go language and implement file compression, encryption, upload, download and decompression functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


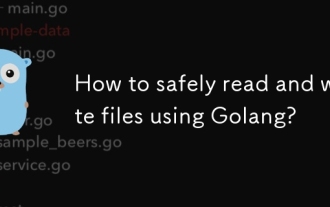
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
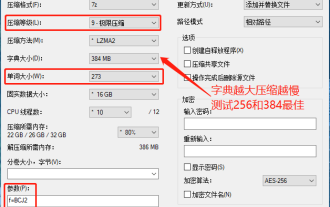
I found that the compressed package downloaded from a download website will be larger than the original compressed package after decompression. The difference is tens of Kb for a small one and several dozen Mb for a large one. If it is uploaded to a cloud disk or paid space, it does not matter if the file is small. , if there are many files, the storage cost will be greatly increased. I studied it specifically and can learn from it if necessary. Compression level: 9-Extreme compression Dictionary size: 256 or 384, the more compressed the dictionary, the slower it is. The compression rate difference is larger before 256MB, and there is no difference in compression rate after 384MB. Word size: maximum 273 Parameters: f=BCJ2, test and add parameter compression rate will be higher
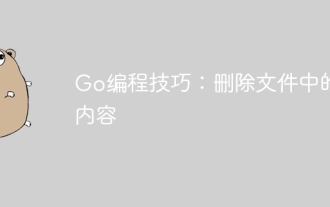
The Go language provides two methods to clear file contents: using io.Seek and io.Truncate, or using ioutil.WriteFile. Method 1 involves moving the cursor to the end of the file and then truncating the file, method 2 involves writing an empty byte array to the file. The practical case demonstrates how to use these two methods to clear content in Markdown files.
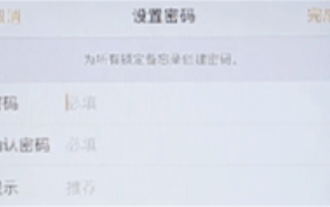
In Apple mobile phones, users can encrypt photo albums according to their own needs. Some users don't know how to set it up. You can add the pictures that need to be encrypted to the memo, and then lock the memo. Next, the editor will introduce the method of setting up the encryption of mobile photo albums for users. Interested users, come and take a look! Apple mobile phone tutorial How to set up iPhone photo album encryption A: After adding the pictures that need to be encrypted to the memo, go to lock the memo for detailed introduction: 1. Enter the photo album, select the picture that needs to be encrypted, and then click [Add to] below. 2. Select [Add to Notes]. 3. Enter the memo, find the memo you just created, enter it, and click the [Send] icon in the upper right corner. 4. Click [Lock Device] below
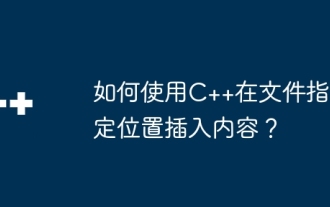
In C++, use the ofstream class to insert content at a specified location in a file: open the file and locate the insertion point. use
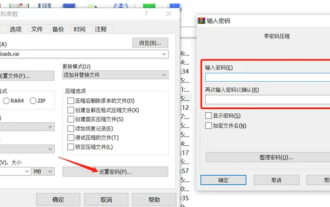
The editor will introduce to you three methods of encryption and compression: Method 1: Encryption The simplest encryption method is to enter the password you want to set when encrypting the file, and the encryption and compression are completed. Method 2: Automatic encryption Ordinary encryption method requires us to enter a password when encrypting each file. If you want to encrypt a large number of compressed packages and the passwords are the same, then we can set automatic encryption in WinRAR, and then just When compressing files normally, WinRAR will add a password to each compressed package. The method is as follows: Open WinRAR, click Options-Settings in the setting interface, switch to [Compression], click Create Default Configuration-Set Password Enter the password we want to set here, click OK to complete the setting, we only need to correct
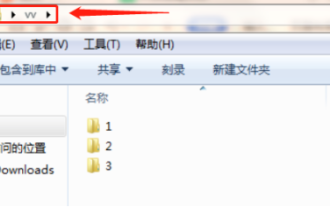
Office workers use wps software very frequently at work. Sometimes they input multiple files a day and then send them to the leader or to a designated location. So how does wps software compress a folder and package it for sending? The editor below will teach you. This operation step. First, organize the files and folders you want to send into the same folder. If you have a lot of files, it's a good idea to name each file so it's easier to identify when sending. Second step, this time click on this large folder and then right-click. Select "Add to archive". Step 3: At this time, the software will automatically help us package our files. Select "Compress to XX.zip". This zip is the packaging format, and then click Compress Now.
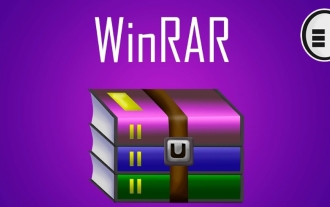
WinRAR is a powerful compressed file management tool that provides rich features and an easy-to-use interface. WinRAR 64-bit version is specially optimized for 64-bit operating systems and can better utilize system resources and performance. Next, let the editor introduce you to winrar 64-bit and explain how to decompress winrar! 1. What is winrar 64-bit software? WinRAR is a powerful compressed package manager. This software can be used to back up your data, reduce the size of email attachments, decompress RAR, ZIP and other files downloaded from the Internet, and create new files in RAR and ZIP formats. The latest WINRAR version is Wi
